Using jQuery with Bootstrap: Supercharging Your Web Projects
In the realm of web development, combining the strengths of various tools and frameworks can lead to exceptional results. When it comes to building interactive and responsive websites, jQuery and Bootstrap stand as two of the most influential players. In this guide, we’ll delve into the synergy between jQuery and Bootstrap, exploring how their collaboration can supercharge your web projects and elevate your development game.
Table of Contents
1. Understanding jQuery and Bootstrap
1.1. What is jQuery?
jQuery is a fast, small, and feature-rich JavaScript library that simplifies HTML document traversal and manipulation, event handling, animation, and more. It abstracts many complex functionalities, providing developers with a streamlined way to interact with the Document Object Model (DOM) and create dynamic web experiences. With jQuery, tasks that would require significant lines of code can often be accomplished with just a few concise statements.
1.2. What is Bootstrap?
Bootstrap, on the other hand, is a widely used open-source front-end framework that aids in designing responsive and visually appealing web interfaces. It offers a plethora of pre-built CSS and JavaScript components, allowing developers to create consistent and modern layouts with minimal effort. From responsive grids to navigation bars, modals to carousels, Bootstrap provides a treasure trove of components that cater to various web development needs.
2. The Power of Integration
2.1. Benefits of Using jQuery with Bootstrap
The combination of jQuery and Bootstrap opens up a world of possibilities for web developers. The two frameworks complement each other beautifully, each contributing its unique strengths to the development process:
- Efficient Development: jQuery’s simplified syntax and powerful DOM manipulation capabilities make it easier to create interactive elements and handle events.
- Rich User Experience: Bootstrap’s ready-to-use components and responsive design tools help create visually appealing interfaces that adapt seamlessly to different screen sizes.
- Rapid Prototyping: The synergy between jQuery and Bootstrap allows for quick prototyping of complex UI elements and dynamic content, enabling faster iteration during the design phase.
- Cross-browser Compatibility: Both frameworks are well-tested across various browsers, ensuring a consistent experience for users regardless of their browser preference.
2.2. Seamless Integration
Integrating jQuery with Bootstrap is straightforward. To start, include the jQuery library in your HTML document using a <script> tag before including the Bootstrap JavaScript files. This ensures that jQuery is available before any Bootstrap components that might depend on it.
html <!DOCTYPE html> <html> <head> <!-- Your head content here --> <!-- Include jQuery --> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <!-- Include Bootstrap CSS and JS --> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css"> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.5.2/js/bootstrap.min.js"></script> </head> <body> <!-- Your body content here --> </body> </html>
3. Getting Started
3.1. Linking jQuery and Bootstrap
Before diving into the coding specifics, it’s essential to ensure that you have access to the latest versions of both jQuery and Bootstrap. Utilizing content delivery networks (CDNs) is a popular method, as demonstrated in the previous code snippet. Alternatively, you can download the libraries and host them locally if needed.
3.2. Your First jQuery-Bootstrap Project
Let’s kick things off by creating a simple project that combines jQuery and Bootstrap. Imagine you want to create a button that changes its color when clicked.
First, create a button element in your HTML:
html <button id="colorButton" class="btn btn-primary">Click me</button>
Next, add a <script> tag to your HTML file to include a custom JavaScript file:
html <script src="path/to/your/script.js"></script>
In your script.js file, add the following jQuery code to change the button’s color when clicked:
javascript $(document).ready(function() { $('#colorButton').click(function() { $(this).toggleClass('btn-primary btn-danger'); }); });
In this example, we’re using jQuery’s event handling capabilities to toggle between the btn-primary and btn-danger Bootstrap classes, effectively changing the button’s color.
4. Enhancing User Interaction
4.1. Creating Dynamic Content with jQuery
jQuery’s power lies in its ability to dynamically manipulate the DOM. Suppose you’re building a blog with comments. You can use jQuery to add new comments without refreshing the page.
javascript $(document).ready(function() { $('#addCommentButton').click(function() { var commentText = $('#commentInput').val(); var newComment = $('<div class="comment">' + commentText + '</div>'); $('#commentContainer').append(newComment); $('#commentInput').val(''); }); });
In this example, we’re extracting the text from an input field, creating a new div element with the comment’s content, and appending it to a comment container.
4.2. Utilizing Bootstrap Components
Bootstrap’s components enhance user experience and save development time. For instance, creating a modal dialog with Bootstrap and then triggering it with jQuery is remarkably simple:
html <!-- Button to trigger the modal --> <button id="myModalButton" class="btn btn-primary" data-toggle="modal" data-target="#myModal">Open Modal</button> <!-- Modal --> <div class="modal fade" id="myModal"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <h4 class="modal-title">Modal Title</h4> <button type="button" class="close" data-dismiss="modal">×</button> </div> <div class="modal-body"> <p>This is a modal dialog powered by Bootstrap and jQuery!</p> </div> <div class="modal-footer"> <button type="button" class="btn btn-secondary" data-dismiss="modal">Close</button> </div> </div> </div> </div>
In this example, we’ve used Bootstrap’s modal component and jQuery’s data-toggle and data-target attributes to control the modal’s appearance.
5. Responsive Design Made Easy
5.1. Bootstrap’s Responsive Grid System
Bootstrap’s grid system allows you to create responsive layouts with ease. Columns automatically adjust based on screen size, making your website look great on various devices.
html <div class="container"> <div class="row"> <div class="col-md-6"> <!-- Content for the first column --> </div> <div class="col-md-6"> <!-- Content for the second column --> </div> </div> </div>
In this example, the two columns will stack on top of each other on smaller screens, ensuring optimal readability.
5.2. Media Queries with jQuery
You can use jQuery to enhance responsive behavior even further. For instance, you might want to change an element’s style when the screen width falls below a certain threshold:
javascript $(window).resize(function() { if ($(window).width() < 768) { $('#myElement').css('font-size', '14px'); } else { $('#myElement').css('font-size', '18px'); } });
In this example, we’re using the resize event to adjust the font size of an element based on the screen width.
6. Advanced Techniques
6.1. Custom Animations and Effects
jQuery provides a wide range of animation methods that can be seamlessly integrated with Bootstrap components to create engaging user experiences. For instance, you can fade in an element when a user scrolls down to it:
javascript $(window).scroll(function() { if ($(this).scrollTop() > 300) { $('#fadeInElement').fadeIn(); } });
6.2. AJAX-powered Functionality
jQuery’s AJAX capabilities are a game-changer when it comes to loading data dynamically without page refreshes. You can combine this with Bootstrap’s loading spinners to provide visual feedback to users:
javascript $('#loadDataButton').click(function() { $('#loadingSpinner').show(); $.ajax({ url: 'data.json', method: 'GET', success: function(response) { // Process the response data $('#loadingSpinner').hide(); } }); });
7. Optimization and Best Practices
7.1. Minifying and Bundling
To optimize performance, it’s recommended to minify and bundle your jQuery and Bootstrap files before deploying to production. Tools like Grunt, Gulp, or Webpack can automate this process.
7.2. Ensuring Compatibility
Always ensure that you’re using compatible versions of jQuery and Bootstrap. Libraries may have dependencies on specific versions, and using incompatible versions could lead to unexpected behavior.
8. Future Trends
As web development continues to evolve, the collaboration between jQuery and Bootstrap remains relevant and impactful. New features, enhancements, and improved performance are likely on the horizon for both frameworks. By mastering the integration of jQuery and Bootstrap, you equip yourself with a versatile toolkit to create captivating and responsive web projects that stand out in the digital landscape.
Conclusion
In conclusion, the marriage of jQuery and Bootstrap can be likened to a symphony where each instrument plays a unique role, culminating in a harmonious composition. The combination of jQuery’s dynamic capabilities and Bootstrap’s elegant components empowers developers to create web experiences that are not only functional but also visually impressive and user-friendly. Whether you’re a seasoned developer or just embarking on your coding journey, harnessing the power of jQuery with Bootstrap is an essential skill that will undoubtedly elevate your web projects to new heights. So dive in, experiment, and watch your web creations flourish!
Table of Contents
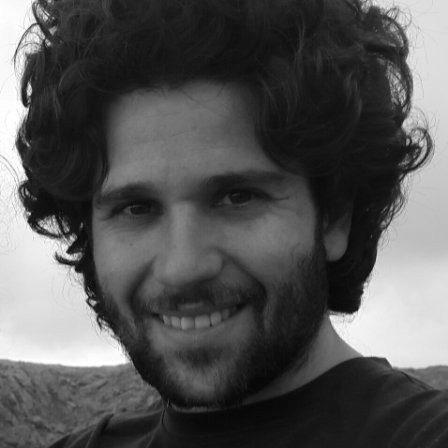
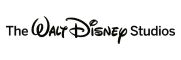