jQuery and Google Maps: Adding Maps to Your Website
In today’s digital age, interactive maps have become a ubiquitous feature on websites. Whether you’re running a business, showcasing travel destinations, or simply want to add a dynamic element to your site, integrating Google Maps can be a game-changer. This tutorial will walk you through the process of embedding Google Maps into your website using jQuery, making it easier for you to create interactive and engaging user experiences.
Table of Contents
1. Why Use Google Maps on Your Website?
Before diving into the technical details, let’s explore why adding Google Maps to your website is such a great idea:
1.1. Location Visibility
If your website involves physical locations, such as a store, restaurant, or event venue, displaying a map can help visitors find you effortlessly. It improves location visibility and assists potential customers in planning their visit.
1.2. Enhanced User Experience
Maps provide an interactive element that can significantly enhance the user experience. Users can explore areas of interest, find directions, and interact with the map to gather valuable information.
1.3. Visual Appeal
A well-designed map can make your website visually appealing. It breaks up text and images, adding variety to your content layout.
1.4. Geographic Data Presentation
If you have data that’s tied to geographic locations, such as real estate listings, customer distribution, or travel guides, maps are a powerful tool to present this data in an engaging way.
Now that you understand the benefits, let’s move on to the technical aspect of adding Google Maps to your website using jQuery.
2. Prerequisites
Before you begin, ensure you have the following prerequisites in place:
2.1. Google Maps API Key
To integrate Google Maps into your website, you’ll need an API key. This key authenticates your application to use Google Maps services. Visit the Google Cloud Console to create an API key.
2.2. jQuery Library
Make sure you have jQuery included in your project. You can either download it from the jQuery website or use a Content Delivery Network (CDN) to include it in your HTML.
Now, let’s dive into the step-by-step process of adding Google Maps to your website.
Step 1: Include jQuery and Google Maps JavaScript API
First, make sure to include jQuery and the Google Maps JavaScript API in your HTML file. You can include jQuery by adding the following code within the <head> section of your HTML document:
html <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Next, include the Google Maps JavaScript API by adding the following code below the jQuery script:
html <script src="https://maps.googleapis.com/maps/api/js?key=YOUR_API_KEY&callback=initMap" async defer></script>
Replace YOUR_API_KEY with the API key you obtained from the Google Cloud Console earlier.
Step 2: Create a Container for Your Map
In your HTML file, create a <div> element to serve as the container for your map. Give it an id so that you can easily reference it in your JavaScript code. Here’s an example:
html <div id="map"></div>
Step 3: Style the Map Container
It’s a good practice to set the height and width of your map container to ensure it displays correctly on your website. You can do this via CSS. For example:
css #map { width: 100%; height: 400px; }
Step 4: Initialize the Map with jQuery
Now, let’s write the JavaScript code to initialize the map using jQuery. Create a new JavaScript file or include this code within a <script> tag in your HTML document.
javascript // Initialize the map function initMap() { // Define the coordinates for the center of the map var center = { lat: 37.7749, lng: -122.4194 }; // San Francisco, CA // Create a new map instance var map = new google.maps.Map(document.getElementById('map'), { center: center, zoom: 14, // Adjust the zoom level as needed }); // Add a marker to the map var marker = new google.maps.Marker({ position: center, map: map, title: 'Hello, World!' // Optional, display a tooltip on the marker }); }
In this code:
- We define the initMap function, which will be called when the Google Maps API is loaded.
- We specify the coordinates for the center of the map. You can replace these with the latitude and longitude of your desired location.
- We create a new map instance and set the initial zoom level.
- Finally, we add a marker to the map, marking the specified location.
Step 5: Test Your Map
It’s essential to test your map to ensure everything is working correctly. Open your HTML file in a web browser, and you should see a map centered on the location you specified, with a marker indicating that location.
3. Customizing Your Map
Now that you have a basic map working on your website, you can customize it further to meet your specific needs. Here are some common customizations:
3.1. Custom Markers
You can use custom markers to make your map more visually appealing and informative. To use a custom marker, replace the google.maps.Marker code in the initMap function with the following:
javascript var marker = new google.maps.Marker({ position: center, map: map, icon: 'path/to/your-marker.png', // Replace with the path to your custom marker image title: 'Hello, World!' });
3.2. Info Windows
Info windows allow you to display additional information when a user clicks on a marker. You can add an info window like this:
javascript // Create an info window var infowindow = new google.maps.InfoWindow({ content: 'This is your custom info window content.', }); // Open the info window when the marker is clicked marker.addListener('click', function() { infowindow.open(map, marker); });
3.3. Adding Multiple Markers
If your website needs to display multiple locations on the map, you can create an array of markers and loop through it to add them to the map. Here’s a basic example:
javascript var locations = [ { lat: 37.7749, lng: -122.4194, title: 'Location 1' }, { lat: 37.775, lng: -122.418, title: 'Location 2' }, // Add more locations as needed ]; // Loop through the locations and add markers to the map locations.forEach(function(location) { var marker = new google.maps.Marker({ position: { lat: location.lat, lng: location.lng }, map: map, title: location.title, }); });
This code creates an array of locations and adds markers for each location to the map.
3.4. Styling Your Map
You can also apply custom styles to your map using JSON. Google provides a Map Styling Wizard that makes it easy to generate custom map styles. To apply a custom style, add the following code to your initMap function:
javascript // Apply custom map styles var mapStyles = [ { featureType: 'water', elementType: 'geometry', stylers: [{ color: '#e9e9e9' }], }, // Add more style rules as needed ]; map.setOptions({ styles: mapStyles });
This code applies custom styles to the map, making it match your website’s design.
Conclusion
Integrating Google Maps into your website using jQuery is a powerful way to enhance user experience and provide valuable location-based information. With the steps outlined in this guide, you can get started quickly and customize your maps to suit your specific needs. Experiment with the provided code samples, explore the Google Maps JavaScript API documentation, and let your creativity run wild to create stunning, interactive maps for your website. Happy mapping!
Table of Contents
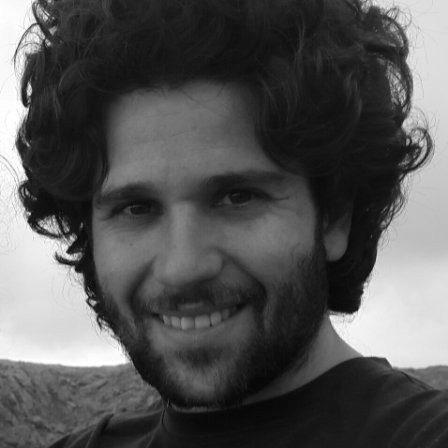
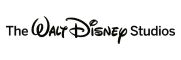