Building Real-Time Notifications with jQuery and Pusher
In today’s fast-paced digital world, real-time notifications have become an essential part of web applications. Whether it’s notifying users about new messages, updates, or important events, providing real-time information keeps users engaged and informed. In this tutorial, we will explore how to build real-time notifications using jQuery and Pusher, a popular WebSocket service. By the end of this guide, you’ll be able to implement real-time notifications in your web application, enhancing the user experience.
Table of Contents
1. Prerequisites
Before we dive into the tutorial, let’s make sure you have the following prerequisites in place:
- Basic knowledge of HTML, CSS, and JavaScript.
- A code editor of your choice (e.g., Visual Studio Code, Sublime Text).
- Node.js and npm (Node Package Manager) installed on your system.
- A Pusher account (sign up at pusher.com).
2. What is Pusher?
Pusher is a cloud-based service that simplifies the implementation of real-time features in web and mobile applications. It provides WebSocket functionality, making it easy to send and receive messages instantly. Pusher offers libraries and SDKs for various programming languages and frameworks, including JavaScript, which we will use in this tutorial.
3. Setting Up Pusher
To get started, you need to set up a Pusher application:
- Sign in or Create an Account: If you don’t already have a Pusher account, sign up at pusher.com. Once you’re logged in, create a new application.
- Create a New App: Click on the “Create a new app” button and follow the steps to configure your app. You’ll need to choose a name, cluster, and other settings.
- Get Your App Keys: After creating your app, you’ll receive your Pusher keys (App ID, Key, and Secret). Keep these keys secure, as they will be used to authenticate and communicate with Pusher.
4. Setting Up the Project
Let’s start by setting up a basic project structure for our real-time notifications application. Open your terminal, create a new project directory, and navigate to it:
bash mkdir real-time-notifications-jquery-pusher cd real-time-notifications-jquery-pusher
Next, initialize a new Node.js project by running:
bash npm init -y
This command will generate a package.json file, which we’ll use to manage our project dependencies. Now, let’s install the necessary packages:
bash npm install express pusher body-parser
Here’s a brief overview of these packages:
- express: A minimal and flexible Node.js web application framework that we’ll use to create a simple server.
- pusher: The Pusher Node.js library that allows us to interact with the Pusher service.
- body-parser: Middleware for parsing HTTP request bodies, which will be useful when working with form data.
5. Creating the Server
Now that we have our project and dependencies set up, let’s create a simple Express server to handle incoming requests and serve our web application.
Create a file named server.js in your project directory and add the following code:
javascript const express = require('express'); const bodyParser = require('body-parser'); const Pusher = require('pusher'); const app = express(); // Middleware app.use(bodyParser.urlencoded({ extended: false })); app.use(bodyParser.json()); // Configure Pusher const pusher = new Pusher({ appId: 'YOUR_APP_ID', key: 'YOUR_APP_KEY', secret: 'YOUR_APP_SECRET', cluster: 'YOUR_APP_CLUSTER', useTLS: true, }); // Serve static files app.use(express.static('public')); // Start the server const port = process.env.PORT || 3000; app.listen(port, () => { console.log(`Server is running on port ${port}`); });
Replace ‘YOUR_APP_ID’, ‘YOUR_APP_KEY’, ‘YOUR_APP_SECRET’, and ‘YOUR_APP_CLUSTER’ with the values from your Pusher application settings.
In this code:
- We import the necessary packages: express, body-parser, and pusher.
- We configure the body-parser middleware to parse JSON and URL-encoded data.
- We set up Pusher using the credentials from your Pusher application.
- We serve static files from a public directory, which we’ll create shortly.
- The server listens on port 3000 (or the PORT environment variable if set).
6. Creating the Frontend
With our server in place, let’s create the frontend of our real-time notifications application. We’ll start by setting up the HTML structure and the jQuery code to handle notifications.
6.1. HTML Structure
Inside your project directory, create a public directory and add an index.html file with the following structure:
html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Real-Time Notifications</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="container"> <h1>Real-Time Notifications</h1> <div class="notification-list"> <ul id="notifications"> <!-- Notifications will appear here --> </ul> </div> <form id="notification-form"> <input type="text" id="notification-text" placeholder="Enter a notification..."> <button type="submit">Send</button> </form> </div> <script src="https://code.jquery.com/jquery-3.6.0.min.js"></script> <script src="app.js"></script> </body> </html>
This HTML structure creates a simple webpage with a heading, a list to display notifications, an input field to enter new notifications, and a button to send them. We’ve also linked to an external styles.css file and two JavaScript files: jquery-3.6.0.min.js (jQuery) and app.js.
6.2. Styling with CSS
Inside the public directory, create a styles.css file to style your webpage. Here’s a basic CSS setup:
css /* Add your styles here */ body { font-family: Arial, sans-serif; background-color: #f5f5f5; margin: 0; padding: 0; display: flex; justify-content: center; align-items: center; min-height: 100vh; } .container { background-color: #fff; border-radius: 8px; box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1); padding: 20px; width: 320px; text-align: center; } h1 { font-size: 24px; } .notification-list { margin-top: 20px; } ul { list-style: none; padding: 0; } li { background-color: #f0f0f0; border-radius: 4px; margin: 4px 0; padding: 8px; text-align: left; font-size: 14px; } form { margin-top: 20px; display: flex; align-items: center; } input[type="text"] { flex-grow: 1; padding: 8px; border: 1px solid #ccc; border-radius: 4px; font-size: 14px; } button { background-color: #007bff; color: #fff; border: none; border-radius: 4px; padding: 8px 16px; margin-left: 8px; font-size: 14px; cursor: pointer; } button:hover { background-color: #0056b3; }
This CSS code defines some basic styles for our notification app, ensuring it looks presentable and user-friendly.
7. Handling Notifications with jQuery
Now, let’s create the JavaScript code to handle notifications using jQuery. Inside your public directory, create an app.js file and add the following code:
javascript $(document).ready(function () { const notificationsList = $('#notifications'); const notificationForm = $('#notification-form'); const notificationText = $('#notification-text'); // Function to append a new notification to the list function appendNotification(text) { const listItem = $('<li></li>').text(text); notificationsList.append(listItem); } // Event listener for the notification form submission notificationForm.submit(function (e) { e.preventDefault(); const text = notificationText.val().trim(); if (text !== '') { // Send the notification to the server $.post('/send-notification', { text }, function (data) { // Clear the input field notificationText.val(''); }); } }); // Initialize Pusher const pusher = new Pusher('YOUR_APP_KEY', { cluster: 'YOUR_APP_CLUSTER', }); // Subscribe to the "notifications" channel const channel = pusher.subscribe('notifications'); // Bind to the "new-notification" event channel.bind('new-notification', function (data) { const text = data.text; appendNotification(text); }); });
Make sure to replace ‘YOUR_APP_KEY’ and ‘YOUR_APP_CLUSTER’ with your Pusher app credentials.
In this JavaScript code:
- We use jQuery to select and cache elements from the HTML, such as the notifications list, the notification form, and the input field.
- The appendNotification function appends a new notification item to the list when called.
- We add an event listener to the notification form’s submission. When the form is submitted, it sends the notification text to the server using a POST request to /send-notification. After sending the notification, it clears the input field.
- We initialize Pusher with your app key and cluster.
- We subscribe to the notifications channel and bind to the new-notification event. When a new notification is received from the server, it’s added to the list of notifications on the frontend.
8. Creating the Real-Time Server
Now, let’s create the server-side logic for handling and broadcasting notifications in real-time.
Create a new file named routes.js in your project directory and add the following code:
javascript const express = require('express'); const Pusher = require('pusher'); const router = express.Router(); // Configure Pusher const pusher = new Pusher({ appId: 'YOUR_APP_ID', key: 'YOUR_APP_KEY', secret: 'YOUR_APP_SECRET', cluster: 'YOUR_APP_CLUSTER', useTLS: true, }); // Route to handle incoming notifications router.post('/send-notification', (req, res) => { const { text } = req.body; // Trigger the "new-notification" event on the "notifications" channel pusher.trigger('notifications', 'new-notification', { text, }); res.sendStatus(200); }); module.exports = router;
Ensure that you replace ‘YOUR_APP_ID’, ‘YOUR_APP_KEY’, ‘YOUR_APP_SECRET’, and ‘YOUR_APP_CLUSTER’ with your Pusher app credentials.
In this code:
- We configure Pusher again, just like we did in the server.js file.
- We create a POST route at /send-notification that listens for incoming notification data.
- When a notification is received, we trigger the new-notification event on the notifications channel, broadcasting the notification text to all connected clients.
9. Routing and Middleware
Next, let’s set up routing and middleware for our Express application. We’ll modify the server.js file to include these changes.
Replace the content of server.js with the following:
javascript const express = require('express'); const bodyParser = require('body-parser'); const Pusher = require('pusher'); const app = express(); // Middleware app.use(bodyParser.urlencoded({ extended: false })); app.use(bodyParser.json()); // Configure Pusher const pusher = new Pusher({ appId: 'YOUR_APP_ID', key: 'YOUR_APP_KEY', secret: 'YOUR_APP_SECRET', cluster: 'YOUR_APP_CLUSTER', useTLS: true, }); // Serve static files app.use(express.static('public')); // Routes const routes = require('./routes'); app.use('/', routes); // Start the server const port = process.env.PORT || 3000; app.listen(port, () => { console.log(`Server is running on port ${port}`); });
Again, replace the Pusher credentials with your own.
In this updated code:
- We import the routes.js file and define the / route to use the routes defined in that file. This separation of concerns makes our code more organized.
10. Testing the Application
Now that we have everything set up, let’s test our real-time notifications application.
- Start your server by running node server.js in your project directory.
- Open a web browser and navigate to http://localhost:3000.
- You should see the Real-Time Notifications page with an input field to enter notifications and a list to display them.
- Enter a notification in the input field and click the “Send” button.
- You should see the notification appear in the list instantly, without needing to refresh the page.
Congratulations! You’ve successfully built a real-time notifications system using jQuery and Pusher. Users of your web application can now receive notifications in real-time, enhancing their overall experience.
Conclusion
Real-time notifications are a powerful way to keep users engaged and informed within your web applications. In this tutorial, we’ve learned how to implement real-time notifications using jQuery and Pusher. We’ve set up a simple server, created the frontend interface, and established the necessary connections to make real-time updates possible. You can now take this knowledge and expand upon it to build more complex real-time features in your own web applications. Stay connected, and keep your users in the loop with real-time notifications!
Table of Contents
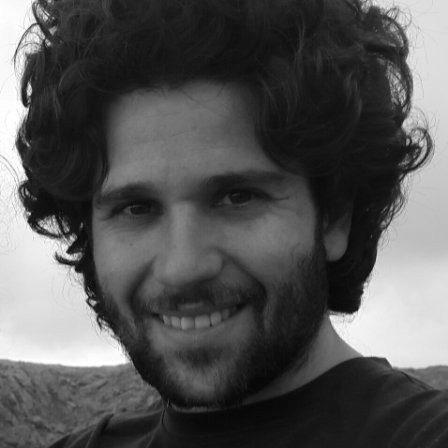
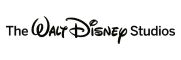