How to implement user authentication in a Django REST framework API?
Implementing user authentication in a Django REST framework (DRF) API is a crucial step to secure your API endpoints and manage user access. DRF offers various authentication methods, including token-based authentication, which is commonly used in RESTful APIs. Here’s a step-by-step guide on how to implement user authentication in a DRF API:
- Install DRF: Ensure you have Django REST framework installed in your project. You can install it using pip:
``` pip install djangorestframework ```
- Configure Authentication: In your project’s `settings.py`, configure DRF authentication settings. Add `’rest_framework.authentication.TokenAuthentication’` to the `DEFAULT_AUTHENTICATION_CLASSES` list. For example:
```python REST_FRAMEWORK = { 'DEFAULT_AUTHENTICATION_CLASSES': ( 'rest_framework.authentication.TokenAuthentication', ), # ... } ```
This setting tells DRF to use token-based authentication for API endpoints.
- Create Tokens: When a user is registered or logs in, create an authentication token for that user. DRF provides a built-in `Token` model for this purpose. You can create tokens manually or use DRF’s `Token` view to generate tokens when users log in.
- Include Authentication: In your API views or viewsets that need authentication, include the `IsAuthenticated` permission class. For example:
```python from rest_framework.permissions import IsAuthenticated class MyApiView(APIView): permission_classes = [IsAuthenticated] def get(self, request): # Your view logic here ```
This ensures that only authenticated users can access the view.
- Include Token in Requests: When making API requests, clients must include the user’s token in the `Authorization` header with the “Token” prefix. For example:
``` Authorization: Token <user_token_here> ```
- Test Authentication: Test your authentication setup by making requests with valid and invalid tokens to ensure that protected views are accessible only to authenticated users.
By following these steps, you can implement user authentication in a Django REST framework API using token-based authentication. This approach provides a secure and straightforward way to manage user access to your API endpoints, ensuring that only authorized users can interact with your API resources.
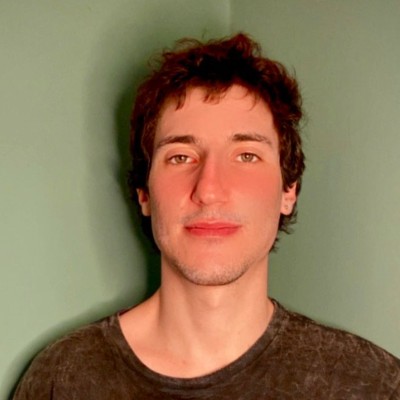
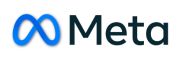