Go Q & A
How do you handle errors returned from concurrent Goroutines in Go?
Handling errors returned from concurrent Goroutines in Go requires careful planning and synchronization mechanisms to ensure that errors are properly captured, propagated, and handled by the calling code. Here’s a systematic approach to handle errors from concurrent Goroutines:
- Error Channels: One common approach is to use channels to propagate errors from Goroutines back to the main routine. You can create a dedicated error channel where Goroutines send any errors they encounter during execution.
- WaitGroup and Error Handling: Use a sync.WaitGroup to wait for all Goroutines to finish their execution. This ensures that the main routine doesn’t exit prematurely before all Goroutines have completed their tasks. Once all Goroutines have finished, you can close the error channel.
- Select Statement: In the main routine, use a select statement to either receive errors from the error channel or proceed with other operations if no errors are encountered. This allows you to handle errors as they occur without blocking the main routine.
- Error Aggregation: Depending on the application’s requirements, you may choose to aggregate errors from multiple Goroutines or handle them individually. Aggregating errors allows you to report all encountered errors at once or take appropriate action based on the severity of errors.
- Error Wrapping: When propagating errors, consider wrapping them with additional context or information to provide better insights into the cause of the error. You can use packages like errors or fmt to wrap errors with additional context before sending them over the error channel.
By following these practices, you can effectively handle errors returned from concurrent Goroutines in Go and ensure robust error handling in your applications.
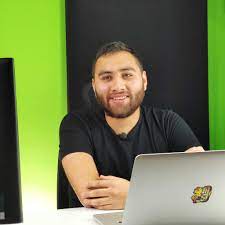
Previously at
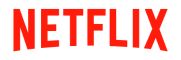
Over 5 years of experience in Golang. Led the design and implementation of a distributed system and platform for building conversational chatbots.