AI Development and Autonomous Vehicles: Advancements in Transportation
The rise of autonomous vehicles marks a significant milestone in the transportation industry. Driven by advancements in artificial intelligence (AI), these self-driving cars promise to enhance safety, efficiency, and convenience. This blog explores the role of AI in the development of autonomous vehicles and highlights key innovations and practical applications in this rapidly evolving field.
Understanding Autonomous Vehicles
Autonomous vehicles (AVs) are equipped with AI systems that allow them to navigate and operate without human intervention. These systems rely on a combination of sensors, machine learning algorithms, and real-time data processing to make driving decisions and adapt to dynamic environments.
Using AI for Autonomous Vehicle Development
AI plays a crucial role in the development and functioning of autonomous vehicles. Here are some key areas where AI is applied, along with practical examples and code snippets to illustrate these applications.
1. Perception and Object Detection
Perception is the ability of an autonomous vehicle to understand its surroundings. AI-driven object detection algorithms help identify and classify objects such as pedestrians, other vehicles, and traffic signs.
Example: Implementing Object Detection with TensorFlow
Here’s a basic example of how you might use TensorFlow to perform object detection on images.
```csharp using System; using TensorFlow; class Program { static void Main() { // Load a pre-trained model var model = TFModel.Load("path/to/model"); // Load an image var image = LoadImage("path/to/image.jpg"); // Perform object detection var results = model.Predict(image); // Process and display results foreach (var result in results) { Console.WriteLine($"Detected {result.Label} with confidence {result.Confidence}"); } } static Tensor LoadImage(string path) { // Implement image loading and preprocessing here } } ```
2. Path Planning and Navigation
Path planning involves determining the optimal route for an autonomous vehicle to follow while avoiding obstacles and adhering to traffic rules. AI algorithms such as A* and Dijkstra’s algorithm are commonly used for this purpose.
Example: Path Planning with A* Algorithm
Here’s an example of implementing the A* algorithm for path planning.
```csharp using System; using System.Collections.Generic; class AStarPathfinding { // Define Node class and A* algorithm implementation here static void Main() { // Set up the grid and nodes var grid = new Grid(10, 10); var start = grid.GetNode(0, 0); var goal = grid.GetNode(9, 9); // Run A* algorithm var path = AStar.FindPath(grid, start, goal); // Output the path foreach (var node in path) { Console.WriteLine($"Path Node: {node.X}, {node.Y}"); } } } ```
3. Decision Making and Control Systems
AI-driven decision-making systems are responsible for making real-time driving decisions, such as when to accelerate, brake, or steer. Reinforcement learning is often used to train these systems to optimize driving behavior.
Example: Reinforcement Learning for Driving Policy
Here’s a simplified example of using reinforcement learning to train a driving policy.
```csharp using System; class ReinforcementLearning { static void Main() { // Define environment and agent var environment = new DrivingEnvironment(); var agent = new DrivingAgent(); // Train the agent for (int episode = 0; episode < 1000; episode++) { var state = environment.Reset(); var done = false; while (!done) { var action = agent.ChooseAction(state); var (nextState, reward, done) = environment.Step(action); agent.UpdatePolicy(state, action, reward, nextState); state = nextState; } } // Evaluate the trained agent agent.Evaluate(); } } ```
4. Simulation and Testing
Simulation is essential for testing autonomous vehicles in a controlled environment before real-world deployment. AI-driven simulators can model various scenarios and assess the vehicle’s performance under different conditions.
Example: Simulating Driving Scenarios
Here’s an example of setting up a simple simulation environment.
```csharp using System; class Simulation { static void Main() { var simulator = new DrivingSimulator(); simulator.LoadScenario("urban_traffic"); // Run the simulation simulator.Start(); // Monitor and analyze the results var results = simulator.GetResults(); Console.WriteLine($"Simulation Results: {results}"); } } ```
Conclusion
AI development is at the forefront of advancements in autonomous vehicle technology. From perception and object detection to path planning, decision making, and simulation, AI is transforming the way self-driving cars operate and interact with their environment. Embracing these technologies will lead to safer, more efficient, and intelligent transportation solutions.
Further Reading
Table of Contents
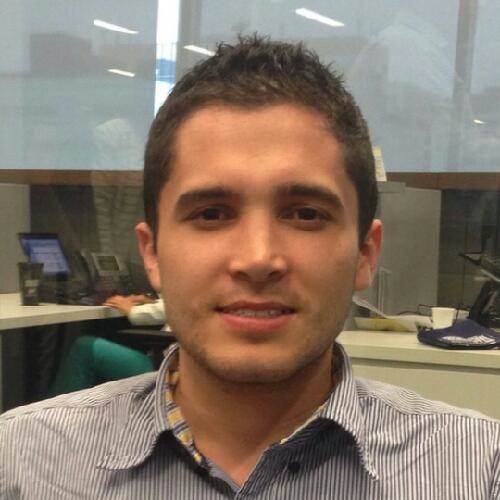
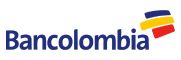