Empowering AI Innovations: Building Robust and Intelligent Applications with Ruby
Artificial Intelligence (AI) is no longer just a concept confined to the pages of science fiction novels. It’s a reality that is transforming various industries, from healthcare to transportation. An increasing number of developers, including those looking to hire AI developers, are turning to various languages for AI applications, one of them being Ruby. Ruby, known for its elegance, simplicity, and readability, stands out as a potent tool in the hands of AI developers.
While Python is traditionally the go-to language for AI, other languages, including Ruby, are increasingly being used. This is due to Ruby’s simple syntax and a large number of libraries that make it suitable for prototyping AI models and developing intelligent applications. This adaptability makes it a sought-after skill for businesses aiming to hire AI developers.
So, let’s dive into how we can leverage Ruby for AI development. We’ll explore various libraries and provide examples of AI applications built using Ruby, shedding light on why Ruby can be a competitive edge for organizations looking to hire AI developers.
Libraries for AI in Ruby
Several libraries and gems (Ruby libraries are often called gems) have been developed to facilitate AI in Ruby. These include:
- Ruby-fann: This library is a binding to the Fast Artificial Neural Network (FANN) library and is often used to create, train, and execute artificial neural networks.
- Ai4r: Short for Artificial Intelligence for Ruby, Ai4r provides a collection of algorithms for machine learning, data mining, and clustering.
- rb-libsvm: This library is a Ruby binding to the LIBSVM library, used for support vector machines, a type of supervised learning model for classification and regression analysis.
- CardMagic-Classifier: This gem uses a Bayesian and Latent Semantic Analysis (LSA) approach to classify data, primarily used in Natural Language Processing.
- Treat: The Text REtrieval and Annotation Toolkit, or Treat, is a toolkit for natural language processing and computational linguistics.
Now that we have a good understanding of the available tools, let’s delve into a few examples of AI applications developed using Ruby.
Example 1: Text Classification with Ai4r
The Ai4r gem has a variety of machine learning algorithms that can be utilized for tasks like classification, clustering, and association. For this example, we’ll use the Naive Bayes classifier for text classification.
```ruby require 'ai4r' include Ai4r::Classifiers include Ai4r::Data data_set = DataSet.new.load_csv_with_labels "mydata.csv" naive_bayes = NaiveBayes.new.build data_set naive_bayes.eval ["Some", "text", "for", "classification"] ```
In the code above, we first include the necessary modules, load our labeled data from a CSV file, build our Naive Bayes classifier with this data, and then classify a new piece of text.
Example 2: Neural Networks with Ruby-fann
Neural networks are the cornerstone of most AI applications, and with Ruby-fann, we can build and train neural networks for tasks such as image recognition or natural language processing.
```ruby require 'ruby-fann' # Data train = RubyFann::TrainData.new(:filename=>'train.data') # Create a network with 2 input neurons, 1 hidden layer with 3 neurons, and 2 output neurons fann = RubyFann::Standard.new(:num_inputs=>2, :hidden_neurons=>[3], :num_outputs=>2) # Train the network fann.train_on_data(train, 1000, 10, 0.1) # 1000 max_epochs, 10 errors between reports, desired error=0.1 ```
In this example, we’re creating a network with 2 input neurons, 3 hidden neurons, and 2 output neurons. We then train this network with our training data.
Example 3: Natural Language Processing with Treat
The Treat gem is a comprehensive toolkit for natural language processing and computational linguistics in Ruby. It can be used to perform tasks like tokenization, part-of-speech tagging, chunking, and named entity recognition. These features not only enhance the capabilities of AI in Ruby but also increase the demand for businesses to hire AI developers who are proficient in utilizing such comprehensive toolkits. By leveraging the power of the Treat gem, AI developers can build more intelligent and complex applications, making them valuable assets for any team looking to hire AI developers.
```ruby require 'treat' include Treat::Core::DSL d = document("This is a sentence. And this is another one.") d.apply(:chunk, :segment, :tokenize, :parse) d.sentences.each do |sentence| sentence.each do |token| puts "#{token} (#{token.class})" end end ```
In this example, we’re applying several NLP techniques to a document containing two sentences. We then iterate over each sentence and print each token and its type.
Example 4: Support Vector Machines with rb-libsvm
Support Vector Machines (SVMs) are supervised learning models typically used for classification and regression analysis. The rb-libsvm library provides a binding to the LIBSVM library and can be used to create SVM models.
```ruby require 'libsvm' include Libsvm problem = Problem.new parameter = SvmParameter.new parameter.cache_size = 1 # in megabytes parameter.eps = 0.001 parameter.c = 10 examples = [[1, 0, 1], [-1, 0, -1]] labels = [1, -1] problem.set_examples(labels, examples) model = Model.train(problem, parameter) pred = model.predict([1, 1, 1]) ```
In this example, we first set the SVM parameters, then create our problem and load our examples and corresponding labels. After that, we train our SVM model. Finally, we predict the class of a new example.
Example 5: Decision Trees with Ai4r
Decision trees are machine learning models that predict the value of a target variable by learning simple decision rules inferred from the data features. They’re easy to understand and interpret, making them very useful for exploratory data analysis. Here’s how we can use Ai4r to create a decision tree:
```ruby require 'ai4r' include Ai4r::Data include Ai4r::Classifiers data_set = DataSet.new.load_csv_with_labels "mydata.csv" decision_tree = Id3.build data_set decision_tree.get_rules ```
In this example, we’re loading our data, building our decision tree, and printing the rules of the tree.
Example 6: Bayesian Classifiers with Classifier-Reborn
Classifier-Reborn is a Ruby gem that implements both a Bayesian classifier and a Latent Semantic Indexer (LSI), useful for content-based categorization and recommendation. Here’s an example of how we can use it for text classification:
```ruby require 'classifier-reborn' # Initialize a new Bayesian classifier classifier = ClassifierReborn::Bayes.new 'Positive', 'Negative' # Train the classifier classifier.train_positive "good, great, fantastic, superb, excellent" classifier.train_negative "bad, worse, worst, terrible, dreadful" # Classify a new sentence classifier.classify "This has been a terrible day" ```
In this example, we’re training our Bayesian classifier on positive and negative words, and then using it to classify a new sentence.
Example 7: K-Means Clustering with Ai4r
K-means clustering is an unsupervised machine learning algorithm that groups similar data points together. It’s often used for exploratory data analysis, customer segmentation, and image segmentation. Here’s how we can implement k-means clustering with Ai4r:
```ruby require 'ai4r' include Ai4r::Data include Ai4r::Clusterers data_set = DataSet.new.load_csv_with_labels "mydata.csv" kmeans = KMeans.new.build(data_set, 3) # Use 3 as the number of clusters kmeans.clusters ```
In this example, we’re loading our data, running k-means clustering with a specified number of clusters (3), and then printing the clusters.
Conclusion
Artificial Intelligence development in Ruby is a rapidly evolving field. The language’s simplicity and readability, combined with its versatile libraries, make it an attractive choice for developers, including those looking to hire AI developers.
While Ruby might not be the first choice for high-performance AI applications—given languages like Python offer more robust support and performance optimization for AI—it still emerges as an excellent choice for prototyping, exploring AI concepts, and building intelligent web applications. This makes Ruby a key skill for AI developers.
The examples provided demonstrate just a few ways to implement AI in Ruby. As AI continues to evolve and mature, and more libraries and tools become available, the potential for AI development in Ruby is sure to expand. Consequently, businesses looking to hire AI developers will likely benefit from including Ruby in their list of desired skills.
Table of Contents
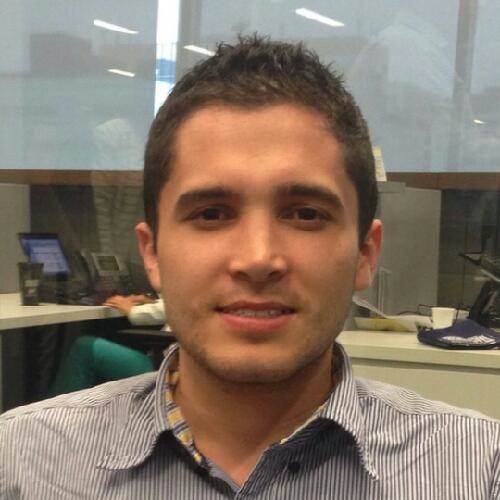
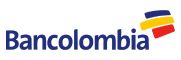