Building Intelligent Chatbots with Natural Language Processing
In today’s digital age, chatbots have become an integral part of various industries, from customer support to e-commerce and beyond. These intelligent conversational agents interact with users, responding to their queries, providing information, and even executing specific tasks. Natural Language Processing (NLP) is the driving force behind the success of modern chatbots. By leveraging NLP techniques, chatbots can understand, interpret, and generate human language, leading to more meaningful and efficient interactions.
In this blog, we’ll dive deep into the world of building intelligent chatbots with Natural Language Processing. We’ll cover the fundamental concepts of NLP, explore the key components of a chatbot, and walk through the steps to create a functional chatbot using Python and some popular NLP libraries.
1. Understanding Natural Language Processing (NLP)
1.1. What is NLP?
Natural Language Processing (NLP) is a field of Artificial Intelligence (AI) that focuses on the interaction between computers and human language. It involves the ability of machines to understand, interpret, and generate human language, including speech and text. NLP plays a pivotal role in enabling chatbots to comprehend user inputs and generate appropriate responses.
2. NLP Techniques
2.1. Tokenization
Tokenization is the process of breaking down a text into individual words or tokens. It forms the foundation of NLP as it allows the chatbot to process each word individually and extract meaningful information.
python # Python code sample for tokenization using NLTK import nltk nltk.download('punkt') from nltk.tokenize import word_tokenize text = "Building intelligent chatbots with NLP." tokens = word_tokenize(text) print(tokens)
2.2. Part-of-Speech (POS) Tagging
POS tagging involves labeling each word in a sentence with its corresponding part of speech, such as noun, verb, adjective, etc. This helps chatbots to understand the grammatical structure of user inputs.
python # Python code sample for POS tagging using NLTK nltk.download('averaged_perceptron_tagger') from nltk import pos_tag pos_tags = pos_tag(tokens) print(pos_tags)
2.3. Named Entity Recognition (NER)
NER identifies and classifies named entities in text, such as names of persons, organizations, locations, etc. This aids chatbots in extracting relevant information from user queries.
python # Python code sample for NER using NLTK nltk.download('words') nltk.download('maxent_ne_chunker') from nltk import ne_chunk ner_tags = ne_chunk(pos_tags) print(ner_tags)
3. Key Components of an Intelligent Chatbot
To build an effective chatbot with NLP capabilities, several key components are essential:
3.1. Natural Language Understanding (NLU)
NLU is responsible for processing and understanding user inputs. It involves tokenization, POS tagging, NER, and other NLP techniques to extract the user’s intent and relevant information from the text.
3.2. Dialogue Management
Dialogue management determines the flow of the conversation between the chatbot and the user. It involves managing context, handling multi-turn conversations, and generating appropriate responses based on the current state of the conversation.
3.3. Natural Language Generation (NLG)
NLG is responsible for generating human-like responses from the chatbot. It uses templates, machine learning algorithms, or other language generation techniques to create coherent and contextually appropriate answers.
3.4. Knowledge Base
A knowledge base is a repository of information that the chatbot can access to provide accurate and relevant responses to user queries. It may include FAQs, product details, or any other structured data.
4. Building an Intelligent Chatbot using Python and NLP Libraries
Now that we understand the core components of an intelligent chatbot, let’s build one using Python and some popular NLP libraries.
Step 1: Install Required Libraries
To get started, make sure you have Python installed on your system. We’ll be using the following libraries:
- nltk: For natural language processing
- tensorflow: For building the NLP model
- flask: For creating a simple web server
python # Python code for installing the required libraries !pip install nltk tensorflow flask
Step 2: Preprocess the Data
The first step in building the chatbot is to preprocess the data. We’ll tokenize the text, convert it to lowercase, and remove any unnecessary characters or stopwords.
python # Python code for data preprocessing import nltk nltk.download('stopwords') from nltk.corpus import stopwords from nltk.tokenize import word_tokenize import string def preprocess_text(text): tokens = word_tokenize(text.lower()) tokens = [token for token in tokens if token.isalpha()] tokens = [token for token in tokens if token not in stopwords.words('english')] return ' '.join(tokens)
Step 3: Implement the Chatbot
Next, we’ll define the NLP model for our chatbot using TensorFlow and train it on our dataset. For simplicity, we’ll use a pre-trained model.
python # Python code for implementing the chatbot using TensorFlow import tensorflow as tf # Define and load the pre-trained model # Create a function to get the chatbot response def get_chatbot_response(user_input): preprocessed_input = preprocess_text(user_input) # Pass the preprocessed_input to the model and get the chatbot's response # ... return chatbot_response
Step 4: Create a Web Interface
To interact with our chatbot, we’ll create a simple web interface using Flask.
python # Python code for creating a web interface from flask import Flask, request, render_template app = Flask(__name__) @app.route('/') def home(): return render_template('index.html') @app.route('/get_response', methods=['POST']) def get_response(): user_input = request.form['user_input'] chatbot_response = get_chatbot_response(user_input) return {'response': chatbot_response} if __name__ == '__main__': app.run(debug=True)
Step 5: Design the Web Interface
Create an HTML template to design the web interface for the chatbot.
html <!-- HTML template for the chatbot web interface (index.html) --> <!DOCTYPE html> <html> <head> <title>Intelligent Chatbot</title> </head> <body> <h1>Welcome to the Intelligent Chatbot</h1> <div> <label for="user_input">You:</label> <input type="text" id="user_input" name="user_input" /> <button onclick="getResponse()">Send</button> </div> <div id="chat_history"></div> <script> function getResponse() { const user_input = document.getElementById("user_input").value; const chat_history = document.getElementById("chat_history"); chat_history.innerHTML += "<p>You: " + user_input + "</p>"; document.getElementById("user_input").value = ""; fetch('/get_response', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ user_input }), }) .then(response => response.json()) .then(data => { const response = data['response']; chat_history.innerHTML += "<p>Chatbot: " + response + "</p>"; }); } </script> </body> </html>
Conclusion
Natural Language Processing has revolutionized the way we interact with machines, and intelligent chatbots are a testament to its power. In this blog, we explored the fundamentals of NLP and its key techniques for building chatbots. We then took a hands-on approach to creating a functional chatbot using Python and popular NLP libraries like NLTK and TensorFlow.
As NLP continues to advance, chatbots will become even more sophisticated, enhancing user experiences, and automating tasks with greater efficiency. By leveraging NLP’s capabilities, businesses can stay ahead in the competitive landscape by providing seamless and intelligent customer interactions.
So, what are you waiting for? Go ahead and build your own intelligent chatbot with NLP, and let the conversations begin!
Table of Contents
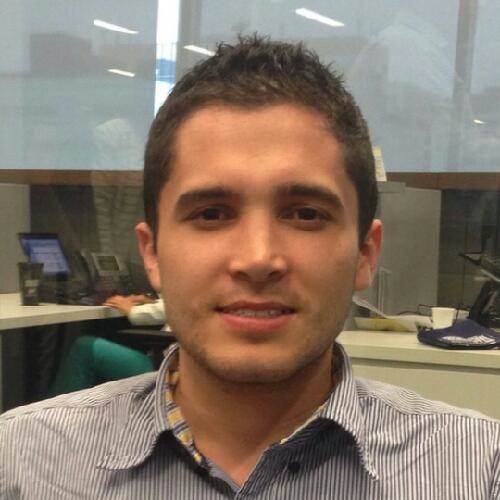
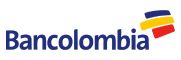