Choosing the Right Programming Language for AI Development
Artificial Intelligence (AI) has rapidly grown into one of the most transformative technologies of the 21st century. From chatbots to autonomous vehicles, AI is reshaping industries and enhancing user experiences across the globe. Behind every AI application lies a programming language that powers its intelligence. With a multitude of options available, choosing the right programming language for AI development can be a daunting task. In this guide, we will explore the factors that influence your choice, popular programming languages for AI, and provide code samples to help you make an informed decision.
Understanding the Factors that Influence Your Choice
Before diving into the realm of programming languages, it’s essential to consider the factors that will influence your decision. Each AI project is unique, and understanding the requirements and constraints will help you choose the most suitable programming language.
1. Project Scope and Complexity
The complexity of your AI project is a critical factor to consider. For smaller projects or prototypes, you may prefer languages that allow for rapid development and ease of implementation. However, for larger and more complex projects, you might need a language that offers better performance and scalability.
2. Library and Framework Support
AI development heavily relies on libraries and frameworks that provide pre-built functions and tools for various tasks. Ensure that the programming language you choose has robust support for AI-related libraries and frameworks, as this can significantly speed up your development process.
3. Community and Resources
A thriving community of developers can be a valuable asset. It means you’ll have access to extensive documentation, tutorials, and online support. Popular programming languages for AI often have larger communities, which can be advantageous when you encounter challenges during your development journey.
4. Performance and Efficiency
AI applications often involve heavy computations, especially in areas like machine learning and deep learning. Opting for a programming language with good performance and efficiency will lead to faster processing times and smoother user experiences.
5. Interoperability
Consider whether your AI project needs to interact with existing systems or applications. If so, choosing a language that supports easy integration with other platforms will be vital.
Popular Programming Languages for AI Development
Now that we understand the factors that play a role in language selection, let’s explore some popular programming languages that are widely used in AI development.
1. Python: The Swiss Army Knife for AI
Python, with its simplicity and versatility, has emerged as the de facto language for AI development. It boasts a rich ecosystem of libraries such as TensorFlow, PyTorch, scikit-learn, and Keras, making it ideal for machine learning and deep learning projects. Let’s take a look at a simple code snippet for image classification using Python and TensorFlow:
python import tensorflow as tf # Load the pre-trained model model = tf.keras.applications.MobileNetV2(weights='imagenet') # Load and preprocess the image img = tf.keras.preprocessing.image.load_img('image.jpg', target_size=(224, 224)) img_array = tf.keras.preprocessing.image.img_to_array(img) img_array = tf.keras.applications.mobilenet_v2.preprocess_input(img_array) img_array = tf.expand_dims(img_array, axis=0) # Make predictions predictions = model.predict(img_array) top_prediction = tf.keras.applications.mobilenet_v2.decode_predictions(predictions, top=1)[0][0] print(f"Predicted object: {top_prediction[1]} with confidence: {top_prediction[2]:.2f}")
2. R: A Statistical Powerhouse
R is a statistical programming language that excels in data analysis, visualization, and statistical modeling. It is widely used in AI research, particularly in areas like data mining, natural language processing, and statistical learning. R’s extensive collection of packages, such as caret and randomForest, makes it a strong contender for AI projects with a focus on data analysis. Here’s an example of a decision tree classification using R:
R # Install required packages install.packages("rpart") install.packages("rpart.plot") # Load the required libraries library(rpart) library(rpart.plot) # Create the dataset (replace with your data) data <- data.frame( feature1 = c(1, 2, 3, 4, 5), feature2 = c(2, 3, 4, 5, 6), target = c("A", "A", "B", "B", "B") ) # Build the decision tree model model <- rpart(target ~ ., data = data) # Plot the decision tree rpart.plot(model)
3. Java: Robust and Scalable
Java, known for its robustness and platform independence, is a preferred language for AI applications that require high performance and scalability. While it might not have the same number of AI-specific libraries as Python, it still offers powerful machine learning frameworks like Deeplearning4j and Weka. Java is an excellent choice for AI projects where performance and reliability are paramount.
java import org.deeplearning4j.datasets.iterator.impl.MnistDataSetIterator; import org.deeplearning4j.nn.api.Model; import org.deeplearning4j.nn.multilayer.MultiLayerNetwork; import org.deeplearning4j.nn.modelimport.keras.trainedmodels.TrainedModels; import org.nd4j.linalg.dataset.api.iterator.DataSetIterator; import org.nd4j.linalg.dataset.api.preprocessor.DataNormalization; import org.nd4j.linalg.dataset.api.preprocessor.ImagePreProcessingScaler; // Load pre-trained Keras model Model model = TrainedModels.VGG16.getMultiLayerNetwork(); // Load and preprocess the image (MNIST example) DataSetIterator dataIterator = new MnistDataSetIterator(1, false, 42); DataNormalization scaler = new ImagePreProcessingScaler(0, 1); scaler.transform(dataIterator.next().getFeatures()); // Make predictions INDArray output = model.output(dataIterator.next().getFeatures()); int predictedLabel = output.argMax(1).getInt(0); System.out.println("Predicted label: " + predictedLabel);
4. Julia: A Language for High-Performance Computing
Julia is gaining popularity in the AI community due to its focus on high-performance computing and ease of use. It combines the best of both worlds, providing a simple syntax similar to Python and performance comparable to C++. For computationally intensive AI projects, Julia can be a game-changer.
julia using Flux using Metalhead # Load pre-trained ResNet model model = Metalhead.ResNet50() # Load and preprocess the image (ImageNet example) img = load("image.jpg") preprocessed_img = Metalhead.preprocess(img) # Make predictions predictions = model(preprocessed_img) top_prediction = argmax(predictions) println("Predicted class: ", Metalhead.IMAGENET_CLASSES[top_prediction])
Conclusion
Selecting the right programming language is a crucial step in AI development. Python remains the go-to choice for its simplicity and the extensive range of AI libraries available. R and Java are solid alternatives, with R excelling in data analysis and statistical modeling, while Java provides robustness and scalability. Julia is emerging as a language for high-performance computing and is worth considering for computationally intensive AI tasks. Ultimately, the best choice depends on your project’s specific requirements and your familiarity with the language. By considering factors like project scope, library support, and community engagement, you can confidently make the right decision and embark on your exciting AI journey.
Table of Contents
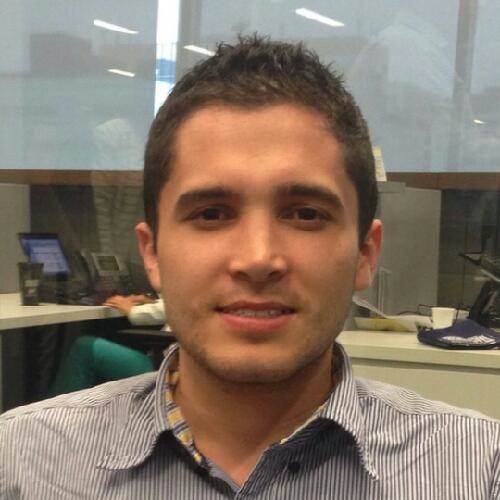
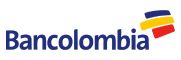