AI Development and Content Recommendation: Personalizing User Experience
In the digital age, content recommendation is a vital aspect of user engagement. AI-driven recommendation systems analyze user behavior and preferences to deliver personalized content, making interactions more relevant and enjoyable. This article explores how AI can be used to develop content recommendation systems and provides practical examples to illustrate the process.
Understanding Content Recommendation Systems
Content recommendation systems use algorithms and data to suggest content that aligns with user preferences. These systems enhance user experience by delivering personalized content, increasing engagement and satisfaction.
Using AI for Content Recommendation
AI, particularly machine learning, plays a crucial role in modern content recommendation systems. By analyzing large datasets, AI can identify patterns and make predictions about user preferences. Below are key aspects of AI development for content recommendation, along with code examples to demonstrate their implementation.
1. Collecting and Preprocessing Data
Data is the foundation of any AI system. To build a recommendation engine, you need to collect and preprocess user interaction data, such as clicks, likes, and views.
Example: Preprocessing User Interaction Data
Assume you have a dataset containing user interactions with various content items. You can use Python’s pandas library to preprocess this data.
```python import pandas as pd # Sample dataset data = { 'user_id': [1, 2, 1, 3, 2], 'content_id': [101, 102, 103, 101, 104], 'interaction': [1, 1, 1, 0, 1] } df = pd.DataFrame(data) # Preprocess data (e.g., normalization, encoding) df['interaction'] = df['interaction'].astype('float32') print(df) ```
2. Building a Recommendation Model
Once the data is ready, you can build a machine learning model to predict which content a user might like. Collaborative filtering and matrix factorization are common techniques used in recommendation systems.
Example: Collaborative Filtering with Surprise
Here’s an example of how you might use the Surprise library to build a collaborative filtering model.
```python from surprise import Dataset, Reader, SVD from surprise.model_selection import train_test_split, accuracy # Load data into Surprise's format reader = Reader(rating_scale=(0, 1)) data = Dataset.load_from_df(df[['user_id', 'content_id', 'interaction']], reader) # Train-test split trainset, testset = train_test_split(data, test_size=0.25) # Train the model algo = SVD() algo.fit(trainset) # Test the model predictions = algo.test(testset) accuracy.rmse(predictions) ```
3. Evaluating and Tuning the Model
Evaluation is essential to ensure the recommendation model is performing well. Metrics like RMSE (Root Mean Square Error) and precision can be used to assess model accuracy.
Example: Evaluating the Recommendation Model
```python from surprise import accuracy # Evaluate the model's predictions accuracy.rmse(predictions) ```
4. Deploying and Scaling the Recommendation System
Once the model is trained and evaluated, you can deploy it to serve recommendations in real-time. Tools like TensorFlow Serving or AWS SageMaker can help in deploying scalable AI models.
Example: Deploying with TensorFlow Serving
```bash # Example of a command to start TensorFlow Serving for a model tensorflow_model_server --rest_api_port=8501 --model_name=recommendation_model --model_base_path=/path/to/model/ ```
5. Improving Recommendations with Deep Learning
Deep learning models like neural collaborative filtering (NCF) can capture complex user-item interactions for better recommendations.
Example: Implementing Neural Collaborative Filtering with Keras
```python from keras.models import Model from keras.layers import Input, Embedding, Flatten, Dot, Dense # Define the model architecture user_input = Input(shape=(1,)) item_input = Input(shape=(1,)) user_embedding = Embedding(input_dim=num_users, output_dim=50)(user_input) item_embedding = Embedding(input_dim=num_items, output_dim=50)(item_input) user_vecs = Flatten()(user_embedding) item_vecs = Flatten()(item_embedding) y = Dot(axes=1)([user_vecs, item_vecs]) y = Dense(1, activation='sigmoid')(y) model = Model(inputs=[user_input, item_input], outputs=y) model.compile(optimizer='adam', loss='binary_crossentropy') # Train the model model.fit([train_user_data, train_item_data], train_labels, epochs=10) ```
Conclusion
AI-powered content recommendation systems are essential tools for delivering personalized user experiences. From data collection to deploying machine learning models, AI offers a comprehensive toolkit for building effective recommendation engines. By harnessing these capabilities, you can create more engaging and personalized content delivery systems, ultimately enhancing user satisfaction and retention.
Further Reading:
Table of Contents
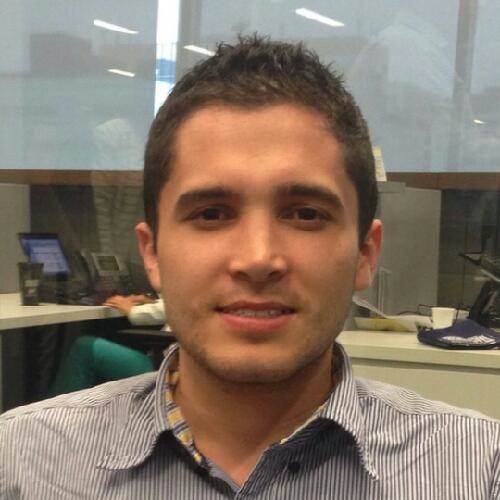
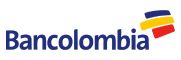