Efficient AI Development with Apache Spark
In the world of artificial intelligence (AI) and machine learning (ML), efficiency is paramount. Developing AI models requires extensive computing power, data processing capabilities, and tools that streamline the development process. Apache Spark, a powerful open-source distributed computing framework, has emerged as a key player in accelerating AI development. In this blog post, we will delve into how Apache Spark can significantly enhance the efficiency of AI development, explore strategies to leverage its capabilities effectively, and provide insightful code examples to illustrate its application.
1. Understanding Apache Spark for AI Development
Apache Spark has gained widespread recognition for its ability to process large-scale data efficiently across distributed computing clusters. While its origins lie in big data processing, Spark’s versatility makes it an excellent choice for AI development as well. Its core strengths lie in its ability to perform in-memory data processing, optimize task scheduling, and support a wide array of data sources, including structured data, unstructured text, graph data, and more. These features directly translate into benefits for AI development:
1.1. Faster Data Processing
AI development heavily relies on data, and the larger the dataset, the more processing power is needed. Spark’s in-memory processing capability accelerates data preparation and feature engineering tasks, leading to faster model iteration cycles. This speed advantage becomes especially crucial when dealing with complex AI algorithms that demand significant computational resources.
1.2. Parallel Computing
Spark’s foundation is built on the concept of parallel computing, enabling it to distribute tasks across a cluster of machines. This parallelism is ideal for AI tasks that involve training and evaluating multiple models simultaneously, significantly reducing the time required for experimentation and hyperparameter tuning.
1.3. Unified Platform
Spark offers a unified platform for various AI-related tasks, including data preprocessing, exploratory data analysis, model training, and deployment. This integrated approach eliminates the need to switch between different tools and environments, streamlining the development pipeline and reducing the chances of compatibility issues.
2. Leveraging Apache Spark for Efficient AI Development
To harness the full potential of Apache Spark for AI development, consider the following strategies:
2.1. Data Preprocessing and Feature Engineering
Efficient AI models hinge on quality data preprocessing and feature engineering. Spark’s DataFrame API simplifies these tasks by providing a high-level abstraction for data manipulation. Let’s look at an example of how Spark can be used for data preprocessing:
python from pyspark.sql import SparkSession # Create a Spark session spark = SparkSession.builder.appName("data_preprocessing").getOrCreate() # Load data into a DataFrame data = spark.read.csv("data.csv", header=True, inferSchema=True) # Clean missing values data = data.na.drop() # Normalize numeric features from pyspark.ml.feature import MinMaxScaler from pyspark.ml import Pipeline feature_cols = ["feature1", "feature2", "feature3"] assembler = VectorAssembler(inputCols=feature_cols, outputCol="features") scaler = MinMaxScaler(inputCol="features", outputCol="scaled_features") pipeline = Pipeline(stages=[assembler, scaler]) data = pipeline.fit(data).transform(data)
2.2. Distributed Model Training
Spark’s MLlib library provides tools for distributed model training. By distributing the training process across a cluster, you can train models on larger datasets and complex architectures. Here’s a simplified example using Spark’s RandomForestClassifier:
python from pyspark.ml.classification import RandomForestClassifier from pyspark.ml.evaluation import BinaryClassificationEvaluator # Split data into training and testing sets train_data, test_data = data.randomSplit([0.8, 0.2], seed=42) # Create a RandomForestClassifier rf = RandomForestClassifier(featuresCol="scaled_features", labelCol="label") # Train the model model = rf.fit(train_data) # Make predictions predictions = model.transform(test_data) # Evaluate the model evaluator = BinaryClassificationEvaluator(labelCol="label") accuracy = evaluator.evaluate(predictions) print("Accuracy:", accuracy)
2.3. Hyperparameter Tuning with Spark
Hyperparameter tuning is a critical part of AI model development. Spark’s MLlib includes tools for performing hyperparameter search in a distributed manner using CrossValidator. Here’s a brief example:
python from pyspark.ml.tuning import ParamGridBuilder, CrossValidator # Define a parameter grid param_grid = ParamGridBuilder() \ .addGrid(rf.maxDepth, [5, 10, 15]) \ .addGrid(rf.numTrees, [50, 100, 150]) \ .build() # Create a CrossValidator crossval = CrossValidator(estimator=rf, estimatorParamMaps=param_grid, evaluator=evaluator, numFolds=3) # Perform cross-validation cv_model = crossval.fit(train_data) best_model = cv_model.bestModel
2.4. Streaming Data for Real-time AI
Real-time AI applications, such as fraud detection or recommendation systems, require continuous processing of streaming data. Spark’s structured streaming capabilities enable developers to build and deploy AI models that handle streaming data seamlessly. Here’s a high-level example:
python from pyspark.sql import SparkSession from pyspark.ml.classification import StreamingRandomForestClassifier # Create a Spark session spark = SparkSession.builder.appName("streaming_ai").getOrCreate() # Load streaming data stream_data = spark.readStream.csv("stream_data/", header=True, inferSchema=True) # Define the model stream_rf = StreamingRandomForestClassifier(featuresCol="features") # Train the streaming model stream_model = stream_rf.fit(stream_data) # Make real-time predictions predictions = stream_model.transform(stream_data)
Conclusion
Apache Spark has proven to be a game-changer in the field of AI development. Its distributed computing capabilities, in-memory data processing, and unified platform make it a versatile tool for accelerating AI model creation. By leveraging Spark for data preprocessing, distributed model training, hyperparameter tuning, and real-time processing, developers can significantly enhance their AI development efficiency. Whether you are working on large-scale machine learning tasks or real-time AI applications, Apache Spark is a powerful asset that can streamline your development workflow and empower you to create more advanced and accurate AI models in less time. So, why not embrace the efficiency and scalability that Apache Spark offers and embark on your journey to AI excellence?
Table of Contents
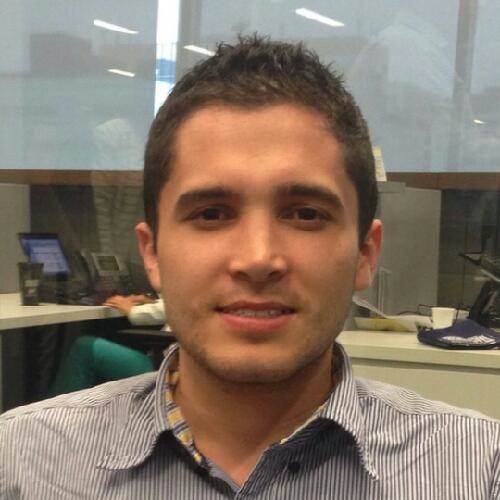
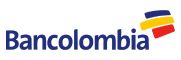