AI Development with MATLAB: From Data Analysis to AI Models
Artificial Intelligence (AI) has become a transformative technology across various industries, driving innovation and automation. MATLAB, a powerful programming environment, provides a comprehensive platform for developing AI solutions. In this blog, we will delve into the exciting journey of AI development with MATLAB, covering the crucial steps from data analysis and preprocessing to building sophisticated AI models.
1. Understanding the Foundation: Data Analysis and Preprocessing
Before diving into AI model development, it’s essential to understand the foundational steps of data analysis and preprocessing. These steps lay the groundwork for building accurate and reliable AI models.
1.1. Importing and Exploring Data
MATLAB offers versatile tools for importing and exploring data. You can read data from various sources, such as CSV files, Excel spreadsheets, or databases, using functions like readtable and xlsread. Once imported, you can visualize and gain insights from your data using plotting functions like plot and scatter.
matlab data = readtable('dataset.csv'); summary(data); scatter(data.Age, data.Income); xlabel('Age'); ylabel('Income'); title('Age vs. Income');
1.2. Data Cleaning and Preprocessing
Data often requires cleaning and preprocessing to address missing values, outliers, and noise. MATLAB provides functions to handle these tasks effectively. For instance, the fillmissing function can impute missing values, and the zscore function can standardize data for modeling.
matlab cleanedData = fillmissing(data, 'linear'); standardizedData = zscore(cleanedData);
2. Building Machine Learning Models
With clean and preprocessed data, you can start building machine learning models using MATLAB’s rich set of functions and toolboxes.
2.1. Supervised Learning: Regression and Classification
For regression tasks, where the goal is to predict a continuous outcome, MATLAB offers algorithms like linear regression, support vector regression, and decision trees. For classification tasks, which involve assigning data to categories, you can use algorithms like logistic regression, support vector machines, and random forests.
matlab % Linear Regression model = fitlm(standardizedData, 'Income ~ Age + Education'); % Support Vector Machine svmModel = fitcsvm(standardizedData(:,1:2), standardizedData.Label, 'KernelFunction', 'rbf'); % Decision Tree Classification treeModel = fitctree(standardizedData(:,1:2), standardizedData.Label);
2.2. Unsupervised Learning: Clustering and Dimensionality Reduction
Unsupervised learning techniques are used when there are no predefined labels in the data. MATLAB supports clustering algorithms such as k-means and hierarchical clustering, as well as dimensionality reduction techniques like principal component analysis (PCA) and t-distributed stochastic neighbor embedding (t-SNE).
matlab % K-Means Clustering idx = kmeans(standardizedData(:,1:2), 3); % Principal Component Analysis coeff = pca(standardizedData); reducedData = standardizedData * coeff(:,1:2); % t-SNE tsneData = tsne(standardizedData);
3. Deep Learning and Neural Networks
As AI evolves, deep learning and neural networks have gained significant attention for their ability to model complex patterns in data. MATLAB provides an extensive deep learning toolbox that simplifies the process of creating and training neural networks.
3.1. Designing Neural Architectures
MATLAB’s deep learning toolbox allows you to design neural architectures using either a high-level approach with the layerGraph object or a simple sequential model using layer functions.
matlab layers = [ imageInputLayer([28 28 1]) convolution2dLayer(3, 16, 'Padding', 'same') reluLayer() maxPooling2dLayer(2, 'Stride', 2) fullyConnectedLayer(10) softmaxLayer() classificationLayer() ];
3.2. Transfer Learning and Pretrained Models
Transfer learning enables you to leverage pretrained neural networks for tasks with limited data. MATLAB supports transfer learning by fine-tuning existing models like AlexNet, ResNet, and VGG.
matlab net = resnet50; % Pretrained ResNet-50 newLayers = [ fullyConnectedLayer(128) reluLayer() fullyConnectedLayer(10) softmaxLayer() classificationLayer() ]; combinedLayers = [net.Layers(1:end-3); newLayers];
3.3. Training and Evaluation
Training a neural network involves feeding it with data and adjusting its weights based on the error. MATLAB provides tools to manage training settings, data augmentation, and validation.
matlab options = trainingOptions('adam', ... 'MaxEpochs', 10, ... 'MiniBatchSize', 64, ... 'ValidationData', valData, ... 'ValidationFrequency', 100, ... 'Plots', 'training-progress'); trainedNet = trainNetwork(trainData, layers, options);
4. Deployment and Beyond
Once you’ve developed and fine-tuned your AI model, it’s time to deploy it for practical use.
4.1. Deployment Options
MATLAB supports various deployment options, including generating standalone executables, deploying to cloud platforms, and integration into mobile and web applications.
matlab % Deploy to MATLAB Production Server compiler = mcc('-m', 'yourScript.m', '-d', 'outputDir'); % Deploy to Cloud deployToCloud(myModel, 'ModelName', 'MyCloudModel'); % Integration with Web Application MATLAB Web App Designer allows seamless integration.
4.2. Continuous Improvement and Monitoring
AI models require continuous improvement and monitoring. MATLAB enables you to monitor model performance, retrain models with new data, and fine-tune hyperparameters to maintain accuracy over time.
Conclusion
MATLAB provides a comprehensive environment for AI development, encompassing data analysis, preprocessing, machine learning, deep learning, deployment, and ongoing improvement. Whether you’re a beginner or an experienced developer, MATLAB’s user-friendly interface and powerful capabilities make it a valuable tool for transforming your AI ideas into reality. Start your journey today and unlock the potential of AI with MATLAB.
In this blog, we explored the journey of AI development with MATLAB, covering key steps from data analysis and preprocessing to building advanced AI models. We looked at code samples and explanations for importing data, cleaning data, building machine learning models, delving into deep learning and neural networks, and deploying AI models for real-world applications. With MATLAB’s versatile tools and rich functionalities, you can embark on an exciting AI development journey and contribute to the forefront of technological innovation.
Table of Contents
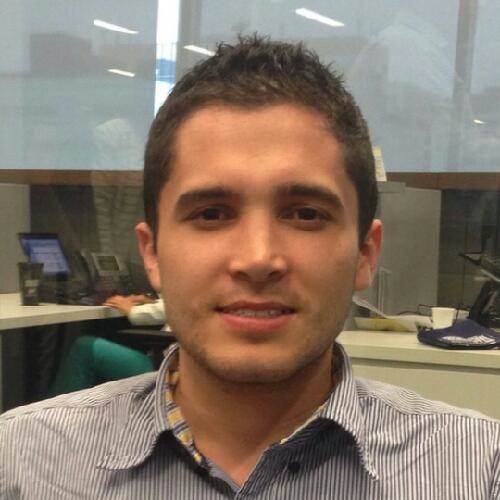
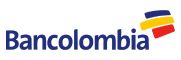