AI Development and Financial Forecasting: Making Accurate Predictions
Financial forecasting is a critical aspect of strategic planning for businesses and investors. Accurate predictions can drive better decision-making and risk management. With advancements in AI and machine learning, financial forecasting has become more precise and insightful. This article explores how AI can be used to enhance financial forecasting and provides practical examples of implementing AI techniques for accurate predictions.
Understanding Financial Forecasting
Financial forecasting involves predicting future financial trends based on historical data and various influencing factors. Effective forecasting helps organizations plan their budgets, manage risks, and make informed investment decisions.
Using AI for Financial Forecasting
AI offers powerful tools and techniques for improving financial forecasting accuracy. By analyzing large volumes of data and identifying complex patterns, AI can provide more reliable predictions. Below are some key aspects and code examples demonstrating how AI can be employed for financial forecasting.
1. Data Collection and Preparation
The first step in financial forecasting is collecting and preparing data. AI models require high-quality data to make accurate predictions. This data can include historical financial metrics, market trends, and economic indicators.
Example: Loading and Preparing Financial Data
Here’s an example of how you might load and prepare financial data using Python and the Pandas library.
```python import pandas as pd # Load financial data from a CSV file data = pd.read_csv('financial_data.csv') # Convert date column to datetime data['Date'] = pd.to_datetime(data['Date']) # Set date as index data.set_index('Date', inplace=True) # Display the first few rows of the dataset print(data.head()) ```
2. Building Predictive Models
AI can be used to build predictive models that forecast future financial trends. Common techniques include time series analysis, regression models, and deep learning.
Example: Time Series Forecasting with ARIMA
Here’s how you might use the ARIMA model from the `statsmodels` library to forecast future financial data.
```python from statsmodels.tsa.arima_model import ARIMA import pandas as pd # Load and prepare the data data = pd.read_csv('financial_data.csv', parse_dates=['Date'], index_col='Date') # Fit ARIMA model model = ARIMA(data['Value'], order=(5, 1, 0)) model_fit = model.fit(disp=0) # Make predictions forecast = model_fit.forecast(steps=12) print(forecast) ```
3. Evaluating Model Performance
Evaluating the performance of your AI models is crucial for ensuring accuracy. Metrics such as Mean Absolute Error (MAE) and Root Mean Squared Error (RMSE) can help assess the quality of predictions.
Example: Calculating Model Performance Metrics
Here’s how you might calculate MAE and RMSE for your forecasting model.
```python from sklearn.metrics import mean_absolute_error, mean_squared_error import numpy as np # True values and predicted values true_values = [100, 150, 200] predicted_values = [110, 140, 190] # Calculate MAE and RMSE mae = mean_absolute_error(true_values, predicted_values) rmse = np.sqrt(mean_squared_error(true_values, predicted_values)) print(f"MAE: {mae}, RMSE: {rmse}") ```
4. Visualizing Forecast Results
Visualizing forecasting results can help in understanding trends and making data-driven decisions. Tools like Matplotlib and Seaborn can be used to create charts and graphs.
Example: Plotting Forecast Results
Here’s how you might visualize forecast results using Matplotlib.
```python import matplotlib.pyplot as plt # Example data dates = pd.date_range(start='2023-01-01', periods=12, freq='M') forecast_values = [110, 140, 190, 220, 250, 280, 310, 340, 370, 400, 430, 460] # Plotting plt.figure(figsize=(10, 6)) plt.plot(dates, forecast_values, label='Forecast', marker='o') plt.xlabel('Date') plt.ylabel('Value') plt.title('Financial Forecast') plt.legend() plt.grid(True) plt.show() ```
5. Integrating with Financial Systems
AI models can be integrated into financial systems for real-time forecasting and decision support. APIs and automated data pipelines can streamline this process.
Example: Fetching Real-Time Financial Data
Here’s how you might fetch real-time financial data using an API.
```python import requests # Define the API endpoint url = "https://api.financialdata.com/latest" # Fetch data from the API response = requests.get(url) data = response.json() # Display the fetched data print(data) ```
Conclusion
AI development offers significant advantages for financial forecasting, including improved accuracy and deeper insights. By leveraging AI techniques such as predictive modeling, performance evaluation, and data visualization, organizations can enhance their forecasting capabilities and make more informed decisions. Embracing these technologies will lead to more effective financial strategies and better risk management.
Further Reading:
Table of Contents
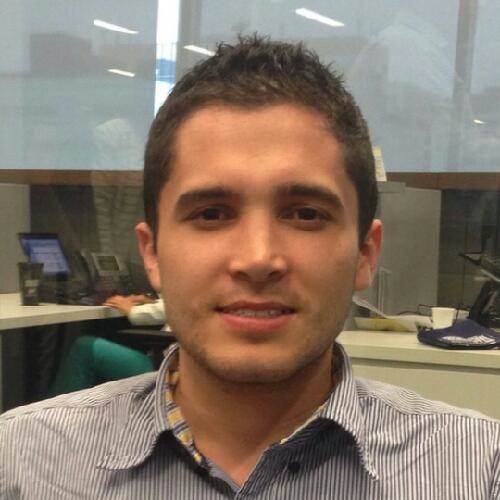
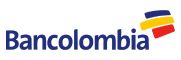