AI Development and Fraud Detection in Insurance: Identifying Suspicious Patterns
Fraud detection is a significant concern in the insurance industry, where fraudulent claims can lead to substantial financial losses. AI technologies offer powerful solutions for identifying suspicious patterns and enhancing fraud detection processes. This article explores how AI can be employed to detect insurance fraud and provides practical examples of how to implement these techniques.
Understanding Fraud Detection in Insurance
Fraud detection involves identifying and preventing fraudulent activities within insurance claims. Effective fraud detection systems help insurance companies minimize losses and ensure the integrity of their operations. AI technologies, with their ability to analyze large datasets and recognize complex patterns, play a crucial role in enhancing these systems.
Using AI for Fraud Detection
AI offers several techniques and algorithms for detecting fraudulent activities. Below are key aspects and code examples demonstrating how AI can be utilized for fraud detection in insurance.
1. Data Collection and Preprocessing
The first step in fraud detection is to collect and preprocess data. AI algorithms require clean and well-structured data to perform effectively.
Example: Preprocessing Claim Data
Assume you have a dataset of insurance claims. You can use Python’s `pandas` library for preprocessing this data.
```python import pandas as pd # Load dataset data = pd.read_csv('insurance_claims.csv') # Data cleaning data.dropna(inplace=True) # Remove missing values data['ClaimAmount'] = data['ClaimAmount'].apply(lambda x: float(x.replace('
2. Building Fraud Detection Models
AI models can be trained to detect fraudulent patterns. Machine learning algorithms like decision trees, random forests, and neural networks are commonly used.
Example: Training a Random Forest Model
Here’s how you might train a random forest classifier to detect fraudulent claims using `scikit-learn`.
```python from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import classification_report # Features and target variable X = data[['ClaimAmount', 'ClaimYear']] y = data['IsFraudulent'] # Train-test split X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42) # Model training model = RandomForestClassifier(n_estimators=100, random_state=42) model.fit(X_train, y_train) # Model evaluation y_pred = model.predict(X_test) print(classification_report(y_test, y_pred)) ```
3. Detecting Suspicious Patterns
AI models can help detect patterns indicative of fraud, such as unusual claim amounts or inconsistent data.
Example: Analyzing Claim Amounts
Here’s a Python script to analyze claim amounts and identify outliers.
```python import numpy as np # Detecting outliers threshold = 3 # Z-score threshold for outliers data['ZScore'] = (data['ClaimAmount'] - data['ClaimAmount'].mean()) / data['ClaimAmount'].std() outliers = data[np.abs(data['ZScore']) > threshold] print("Suspicious Claims Detected:") print(outliers) ```
4. Integrating AI with Fraud Detection Systems
AI can be integrated with existing fraud detection systems to provide real-time alerts and analysis.
Example: Real-Time Fraud Detection
Using a REST API, you can integrate the AI model with a fraud detection system to evaluate new claims in real time.
```python from flask import Flask, request, jsonify app = Flask(__name__) @app.route('/predict', methods=['POST']) def predict(): data = request.json claim_amount = data['ClaimAmount'] claim_year = data['ClaimYear'] # Predict fraud prediction = model.predict([[claim_amount, claim_year]]) result = 'Fraudulent' if prediction[0] == 1 else 'Not Fraudulent' return jsonify({'prediction': result}) if __name__ == '__main__': app.run(debug=True) ```
Conclusion
AI technologies offer robust solutions for detecting fraud in the insurance industry. From preprocessing and analyzing data to training models and integrating real-time systems, AI can significantly enhance fraud detection capabilities. Implementing these AI-driven approaches will lead to more accurate and efficient fraud prevention strategies, ultimately safeguarding your organization.
Further Reading:
, '').replace(',', ''))) # Feature engineering data['ClaimDate'] = pd.to_datetime(data['ClaimDate']) data['ClaimYear'] = data['ClaimDate'].dt.year ```
2. Building Fraud Detection Models
AI models can be trained to detect fraudulent patterns. Machine learning algorithms like decision trees, random forests, and neural networks are commonly used.
Example: Training a Random Forest Model
Here’s how you might train a random forest classifier to detect fraudulent claims using `scikit-learn`.
3. Detecting Suspicious Patterns
AI models can help detect patterns indicative of fraud, such as unusual claim amounts or inconsistent data.
Example: Analyzing Claim Amounts
Here’s a Python script to analyze claim amounts and identify outliers.
4. Integrating AI with Fraud Detection Systems
AI can be integrated with existing fraud detection systems to provide real-time alerts and analysis.
Example: Real-Time Fraud Detection
Using a REST API, you can integrate the AI model with a fraud detection system to evaluate new claims in real time.
Conclusion
AI technologies offer robust solutions for detecting fraud in the insurance industry. From preprocessing and analyzing data to training models and integrating real-time systems, AI can significantly enhance fraud detection capabilities. Implementing these AI-driven approaches will lead to more accurate and efficient fraud prevention strategies, ultimately safeguarding your organization.
Further Reading:
Table of Contents
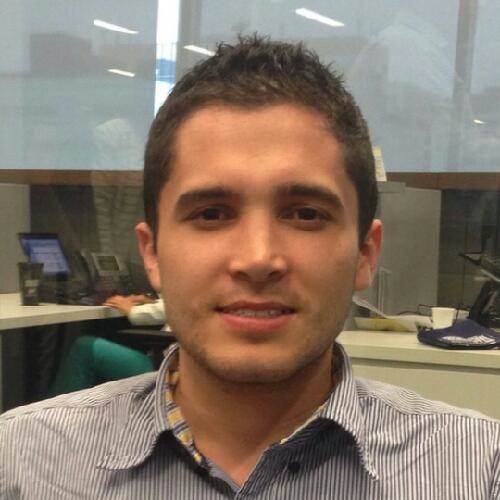
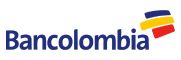