AI Development and Language Translation: Breaking Barriers
Language translation has always been a challenging task, requiring a deep understanding of context, culture, and semantics. However, with advancements in artificial intelligence (AI), we are witnessing a transformative shift in how languages are translated. AI-powered language translation is breaking down barriers, enabling seamless communication across the globe. This blog explores the role of AI in language translation and provides practical examples of its application.
Understanding AI in Language Translation
AI in language translation involves using machine learning models, particularly neural networks, to convert text from one language to another. Unlike traditional translation methods, AI can learn from vast datasets, improving accuracy and context understanding over time. This results in translations that are not only more accurate but also culturally relevant.
Using AI for Language Translation
AI offers powerful tools and frameworks that make language translation more efficient and accessible. Below are some key aspects and code examples demonstrating how AI can be utilized for language translation.
1. Training a Neural Machine Translation Model
The first step in AI-based language translation is training a neural machine translation (NMT) model. Frameworks like TensorFlow and PyTorch provide tools to develop and train these models.
Example: Training a Simple Translation Model with TensorFlow
Here’s a basic example of how to set up and train a translation model using TensorFlow.
```python import tensorflow as tf from tensorflow.keras.layers import Embedding, LSTM, Dense from tensorflow.keras.models import Sequential # Sample data (input and output sequences) input_texts = ["Hello", "How are you?"] target_texts = ["Hola", "¿Cómo estás?"] # Building the model model = Sequential() model.add(Embedding(input_dim=10000, output_dim=64)) model.add(LSTM(64)) model.add(Dense(10000, activation='softmax')) # Compiling the model model.compile(optimizer='adam', loss='sparse_categorical_crossentropy') # Example of model training (with dummy data) # model.fit(input_texts, target_texts, epochs=10) ```
2. Translating Text with Pre-trained Models
For those who may not have the resources to train models from scratch, pre-trained models can be utilized. Libraries like Hugging Face’s Transformers provide access to state-of-the-art models for various language translation tasks.
Example: Translating Text Using a Pre-trained Transformer Model
```python from transformers import MarianMTModel, MarianTokenizer # Load pre-trained model and tokenizer for English to Spanish translation model_name = 'Helsinki-NLP/opus-mt-en-es' model = MarianMTModel.from_pretrained(model_name) tokenizer = MarianTokenizer.from_pretrained(model_name) # Input text text = "Hello, how are you?" # Perform translation translated = model.generate(tokenizer(text, return_tensors="pt", padding=True)) translated_text = [tokenizer.decode(t, skip_special_tokens=True) for t in translated] print(translated_text[0]) # Output: "Hola, ¿cómo estás?" ```
3. Enhancing Translation Quality with Post-Editing
Even with AI, some translations may require human intervention to ensure quality. AI can assist in post-editing by suggesting improvements based on learned patterns.
Example: Implementing a Post-Editing System
```python def post_edit_translation(translation): # Example of simple post-editing rules if "¿cómo estás?" in translation: translation = translation.replace("¿cómo estás?", "¿Cómo estás?") return translation translated_text = "hola, ¿cómo estás?" edited_text = post_edit_translation(translated_text) print(edited_text) # Output: "Hola, ¿Cómo estás?" ```
4. Integrating AI Translation with Applications
AI translation can be integrated into various applications, such as chatbots, content management systems, or mobile apps, to provide real-time translation services.
Example: Integrating Translation in a Chatbot
```python from transformers import pipeline # Load translation pipeline translator = pipeline("translation_en_to_es") def chatbot_response(user_input): # Translate user input translated_input = translator(user_input)[0]['translation_text'] # Example: Send translated input to another service or process it further return f"Translated: {translated_input}" response = chatbot_response("What is your name?") print(response) # Output: "Translated: ¿Cuál es tu nombre?" ```
Conclusion
AI is revolutionizing language translation, making it more accurate, context-aware, and accessible. From training custom models to using pre-trained models and enhancing translations through post-editing, AI provides a range of tools to break down language barriers. Integrating these technologies into your applications can lead to more effective communication across different languages, fostering greater global connectivity.
Further Reading:
Table of Contents
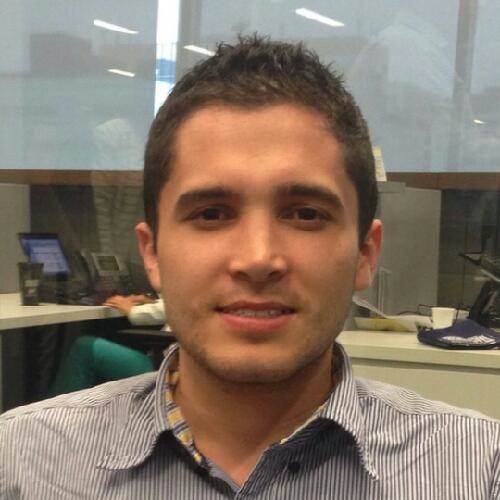
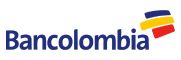