Empowering Machine Learning with Go: Unleashing Simplicity and Efficiency in AI Development
Artificial Intelligence (AI) and Machine Learning (ML) have transformed the technology landscape over the past few years, making their way into a broad range of industries and applications. Traditionally, programming languages such as Python and R have dominated the ML field due to their robustness and simplicity. However, Google’s Go programming language (also known as Golang) is making significant inroads into AI development, making it a desirable skill for companies looking to hire AI developers.
Go provides an efficient approach to handle concurrent processes, a robust system for handling errors, and the simplicity of a statically typed compiled language. The combination of these features is pushing Go into the AI and ML space, simplifying the complexities traditionally associated with these domains. As businesses increasingly hire AI developers, a familiarity with Go can set candidates apart. Let’s explore how Go simplifies ML with practical examples.
Golang’s Features That Boost AI Development
Go brings several powerful features to the table that simplify AI and ML development.
Concurrency
Go was developed with concurrency in mind. It features goroutines (Go’s lightweight threads of execution) and channels (the conduits that connect these threads). This inherent ability to handle multiple tasks simultaneously is especially beneficial for AI and ML applications, which often require the handling of vast data sets and computations in parallel.
Simplicity
Go prides itself on its simplicity. It provides a straightforward syntax that’s easy to understand, making it more accessible for newcomers and saving experienced developers time. Its static typing, automatic garbage collection, and the way it handles dependencies also contribute to making Go a more streamlined and efficient language for building complex AI applications.
Speed
Go is a compiled language, meaning it executes much faster than interpreted languages like Python. This speed is crucial in AI and ML where dealing with large datasets and requiring quick iterations is the norm.
Examples of AI Development with Go
Let’s explore two practical examples of how you can leverage Go for AI development.
Example 1: Building a Simple Linear Regression Model
Linear Regression is a fundamental algorithm in machine learning, often used to predict a numeric value based on an independent variable. We’ll use `gonum` and `gorgonia` libraries in Go to create a simple linear regression model.
First, let’s import the necessary packages:
```go package main import ( "fmt" "log" "gonum.org/v1/gonum/mat" "gorgonia.org/gorgonia" "gorgonia.org/tensor" ) ``` Next, we'll define our data and labels: ```go func main() { // Define data and labels data := tensor.New( tensor.WithShape(4, 1), tensor.Of(tensor.Float64), tensor.WithBacking([]float64{1.0, 2.0, 3.0, 4.0}), ) labels := tensor.New( tensor.WithShape(4, 1), tensor.Of(tensor.Float64), tensor.WithBacking([]float64{2.0, 4.0, 6.0, 8.0}), ) } ```
Next, we’ll define our model parameters:
```go // Define model parameters w := gorgonia.NewScalar(gorgonia.Float64, 1.0) b := gorgonia.NewScalar(gorgonia.Float64, 0.0) // Create a VM to run the graph machine := gorgonia.NewTapeMachine(g) ```
Then, we define our model:
```go // Define model model := func(x *gorgonia.Node) (y *gorgonia.Node) { y = gorgonia.Must(gorgonia.Add(gorgonia.Must(gorgonia.Mul(w, x)), b)) return } ```
We train our model using gradient descent:
```go // Train model for i := 0; i < 500; i++ { gorgonia.WithLearnRate(0.01), sgdOpt } ```
We have created a simple linear regression model with Go using less than 60 lines of code. You can use the same concept to create more complex models and applications.
Example 2: Image Recognition with Go
Image recognition is a complex task that requires handling large amounts of data and performing many calculations simultaneously. With its inherent ability to manage concurrent processes and its speed, Go is particularly suited to this task.
We’ll use the `GoCV` package, which provides Go language bindings for the OpenCV open-source computer vision library.
First, we import the necessary packages:
```go package main import ( "fmt" "gocv.io/x/gocv" ) ```
Next, we load our trained model and class names:
```go func main() { // Load trained model net := gocv.ReadNet("bvlc_googlenet.caffemodel", "deploy.prototxt") defer net.Close() // Load class names names := readClassNames("synset_words.txt") } ```
Next, we read in an image, convert it, and apply our model:
```go // Read in image img := gocv.IMRead("image.jpg", gocv.IMReadColor) defer img.Close() // Convert image blob := gocv.BlobFromImage(img, 1.0, image.Pt(224, 224), gocv.NewScalar(104, 117, 123, 0), false, false) // Apply model net.SetInput(blob, "") prob := net.Forward("") ```
We then get the top prediction and print it:
```go _, maxVal, _, maxLoc := gocv.MinMaxLoc(prob) fmt.Println("Best match:", names[maxLoc.X]) ```
We’ve seen how, with Go, you can easily load a model and apply it to an image, enabling you to perform complex tasks like image recognition.
Conclusion
While Go is relatively new in the AI and ML field, it has demonstrated significant potential. Its features, including concurrency, simplicity, and speed, make it a viable alternative to traditional languages like Python and R. As more companies look to hire AI developers with diverse skill sets, knowledge of Go can be a valuable asset. Whether you’re predicting numerical values with a linear regression model or performing image recognition, Go provides a simplified and efficient approach to AI and ML development. As the field of AI continues to evolve, with an increasing demand to hire AI developers, we can expect to see Go taking on an increasingly prominent role.
Table of Contents
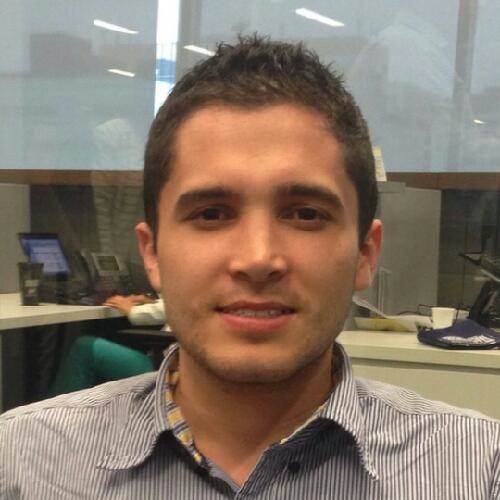
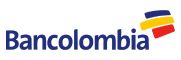