Simplifying AI Development with Microsoft’s Cognitive Toolkit
Artificial Intelligence (AI) has emerged as a transformative technology, revolutionizing industries and empowering businesses to deliver innovative solutions. However, developing AI applications can be a daunting task, requiring specialized knowledge and complex algorithms. Microsoft’s Cognitive Toolkit (CNTK) comes to the rescue, offering a comprehensive framework that simplifies AI development. In this blog, we’ll explore how Microsoft’s Cognitive Toolkit can be a game-changer in the world of AI, its key features, and provide code samples to demonstrate its capabilities.
I. What is Microsoft’s Cognitive Toolkit (CNTK)?
Microsoft’s Cognitive Toolkit, also known as CNTK, is an open-source deep learning framework designed to facilitate AI development. Initially developed by Microsoft Research, CNTK has grown into a versatile and efficient tool for building various types of neural networks. Whether you’re working on natural language processing, computer vision, speech recognition, or any other AI-related task, CNTK provides a rich set of tools and functionalities.
2. Advantages of Microsoft’s Cognitive Toolkit
2.1 High Performance and Scalability:
CNTK stands out for its impressive performance and scalability. It takes advantage of multi-GPU and multi-machine configurations, allowing you to train large-scale models efficiently. This performance edge is crucial, especially when dealing with extensive datasets or complex AI models.
2.2 Flexibility and Customization:
With CNTK, developers enjoy a high degree of flexibility. It offers low-level control over the neural network architecture, enabling customization of every aspect. Additionally, CNTK supports various data types, activation functions, and optimization algorithms, giving you the freedom to experiment and fine-tune your models.
3. Getting Started with Microsoft’s Cognitive Toolkit
To begin harnessing the potential of CNTK, follow these simple steps:
3.1 Installation:
CNTK supports various operating systems, including Windows, Linux, and macOS. You can choose to install it via pip, Docker, or from the source code available on GitHub. Detailed installation instructions can be found on the official CNTK website.
3.2 Dataset Preparation:
Before training an AI model, you need a well-prepared dataset. CNTK supports popular data formats like text, images, and numerical data. Ensure your data is correctly formatted and preprocessed to achieve better results.
4. Building Neural Networks with CNTK
4.1 Define the Network:
CNTK follows a symbolic approach, where you define the neural network as a symbolic graph. This graph represents the flow of data through different layers of the network. Let’s see an example of building a simple feedforward neural network using CNTK:
python import cntk as C # Define input and output dimensions input_dim = 784 output_dim = 10 # Define the input and output variables input_var = C.input_variable(input_dim) output_var = C.input_variable(output_dim) # Define the neural network model def simple_feedforward(input_var): hidden_layer = C.layers.Dense(64, activation=C.relu)(input_var) output_layer = C.layers.Dense(output_dim, activation=None)(hidden_layer) return output_layer # Create the model model = simple_feedforward(input_var)
In this code sample, we created a feedforward neural network with one hidden layer having 64 neurons and an output layer with 10 neurons representing different classes in the dataset.
4.2 Training the Model:
Once the network is defined, the next step is to train it using your dataset. CNTK provides a straightforward API to define the loss function, optimization algorithm, and evaluation metrics.
python # Define the loss and the evaluation function loss = C.cross_entropy_with_softmax(model, output_var) metric = C.classification_error(model, output_var) # Define the optimizer learning_rate = 0.01 lr_schedule = C.learning_rate_schedule(learning_rate, C.UnitType.minibatch) learner = C.sgd(model.parameters, lr_schedule) # Create a Trainer trainer = C.Trainer(model, (loss, metric), [learner]) # Train the model num_epochs = 10 batch_size = 64 for epoch in range(num_epochs): for batch in range(0, len(training_data), batch_size): input_batch = training_data[batch:batch + batch_size] output_batch = training_labels[batch:batch + batch_size] trainer.train_minibatch({input_var: input_batch, output_var: output_batch})
4.3 Evaluation and Testing:
After training, it’s essential to evaluate the model’s performance on a separate test dataset to assess its accuracy and generalization capabilities.
python # Evaluate the model test_accuracy = 0 num_test_batches = len(test_data) // batch_size for batch in range(num_test_batches): input_batch = test_data[batch:batch + batch_size] output_batch = test_labels[batch:batch + batch_size] # Compute the evaluation metric evaluation = trainer.test_minibatch({input_var: input_batch, output_var: output_batch}) test_accuracy += evaluation[metric] test_accuracy /= num_test_batches print("Test Accuracy: {:.2f}%".format((1 - test_accuracy) * 100))
5. Advanced Features of Microsoft’s Cognitive Toolkit
5.1 Pre-trained Models and Transfer Learning:
CNTK comes with pre-trained models for various tasks, such as image classification and language understanding. Leveraging these models and applying transfer learning can significantly speed up your AI development process.
5.2 Distributed Training:
As your AI projects scale, CNTK’s distributed training capabilities become invaluable. You can distribute training across multiple machines or GPUs, reducing the overall training time for complex models.
Conclusion
Microsoft’s Cognitive Toolkit (CNTK) has emerged as a powerful and flexible deep learning framework, making AI development more accessible than ever. Its performance, scalability, and ease of use make it a preferred choice for AI researchers and developers alike. With CNTK’s intuitive APIs and extensive documentation, you can effortlessly create cutting-edge AI applications, empowering your business to thrive in the era of artificial intelligence. So why wait? Dive into the world of CNTK and unlock the true potential of AI!
Table of Contents
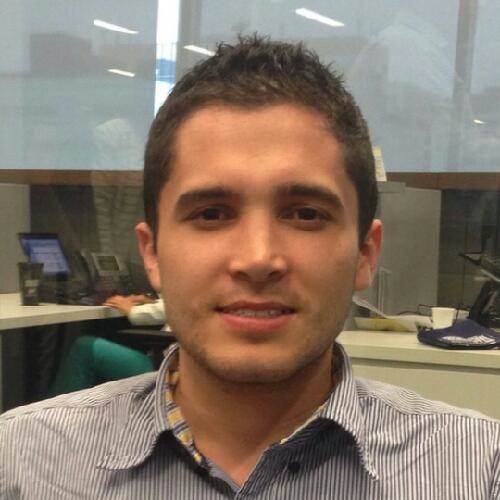
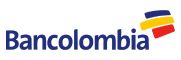