AI Development and Predictive Maintenance: Maximizing Equipment Lifespan
Predictive maintenance uses advanced analytics and machine learning to predict when equipment will fail, allowing organizations to perform maintenance just in time to avoid unexpected breakdowns. By integrating AI into predictive maintenance strategies, businesses can significantly extend the lifespan of their equipment and improve operational efficiency. This blog explores how AI can be applied to predictive maintenance, with practical examples of analyzing and forecasting equipment performance.
Understanding Predictive Maintenance
Predictive maintenance involves monitoring equipment conditions and using data-driven insights to forecast potential failures before they occur. This proactive approach contrasts with reactive maintenance (fixing equipment after it fails) and preventive maintenance (performing maintenance at regular intervals). The goal is to optimize maintenance schedules, reduce downtime, and extend the equipment’s operational lifespan.
Using AI for Predictive Maintenance
AI excels in analyzing large volumes of data to uncover patterns and make accurate predictions. Machine learning algorithms, in particular, can process historical data and sensor readings to identify indicators of equipment wear and potential failure. Below are key aspects and code examples demonstrating how AI can be utilized for predictive maintenance.
1. Collecting and Preprocessing Equipment Data
Effective predictive maintenance starts with collecting and preprocessing data from various sources, such as sensors, logs, and historical records. AI models require clean and relevant data to make accurate predictions.
Example: Preprocessing Sensor Data for Analysis
Assume you have sensor data in CSV format. You can use Python with libraries like `pandas` for data preprocessing.
```python import pandas as pd # Load sensor data data = pd.read_csv('sensor_data.csv') # Drop missing values and normalize data data = data.dropna() data['normalized_value'] = (data['sensor_value'] - data['sensor_value'].mean()) / data['sensor_value'].std() # Save preprocessed data data.to_csv('preprocessed_sensor_data.csv', index=False) ```
2. Training a Machine Learning Model for Failure Prediction
Machine learning models can be trained to predict equipment failures based on historical data. Algorithms like Random Forests, Support Vector Machines, or Neural Networks can be used.
Example: Training a Random Forest Model
Here’s how you might train a Random Forest classifier using Python and `scikit-learn` to predict equipment failures.
```python from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import accuracy_score import pandas as pd # Load preprocessed data data = pd.read_csv('preprocessed_sensor_data.csv') X = data[['normalized_value']] y = data['failure'] # Split data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42) # Train Random Forest model model = RandomForestClassifier() model.fit(X_train, y_train) # Make predictions and evaluate y_pred = model.predict(X_test) accuracy = accuracy_score(y_test, y_pred) print(f'Accuracy: {accuracy:.2f}') ```
3. Implementing Real-Time Failure Prediction
For real-time applications, AI models can be deployed to monitor equipment continuously and provide predictions based on live data.
Example: Real-Time Prediction with a Deployed Model
Assuming you have a trained model saved, you can load it and use it for real-time predictions.
```python import joblib import pandas as pd # Load trained model model = joblib.load('random_forest_model.pkl') # Function to make real-time predictions def predict_failure(sensor_value): data = pd.DataFrame({'sensor_value': [sensor_value]}) data['normalized_value'] = (data['sensor_value'] - data['sensor_value'].mean()) / data['sensor_value'].std() prediction = model.predict(data[['normalized_value']]) return prediction[0] # Example usage sensor_value = 0.5 prediction = predict_failure(sensor_value) print(f'Failure Prediction: {"Yes" if prediction == 1 else "No"}') ```
4. Visualizing and Interpreting Predictive Maintenance Data
Visualization helps in understanding the predictive maintenance results and trends. Libraries such as `matplotlib` or `Plotly` can be used to create insightful charts.
Example: Visualizing Predictive Maintenance Trends
Here’s how you might visualize predicted failure probabilities over time.
```python import matplotlib.pyplot as plt import pandas as pd # Load prediction results results = pd.read_csv('prediction_results.csv') timestamps = pd.to_datetime(results['timestamp']) probabilities = results['failure_probability'] # Plot results plt.figure(figsize=(10, 6)) plt.plot(timestamps, probabilities, label='Failure Probability') plt.xlabel('Time') plt.ylabel('Probability') plt.title('Predictive Maintenance Trends') plt.legend() plt.show() ```
Conclusion
AI enhances predictive maintenance by analyzing equipment data, predicting failures, and optimizing maintenance schedules. Implementing AI-driven predictive maintenance can lead to significant cost savings, reduced downtime, and longer equipment lifespan. Leveraging these technologies effectively ensures a more proactive and efficient approach to maintaining your equipment.
Further Reading:
Table of Contents
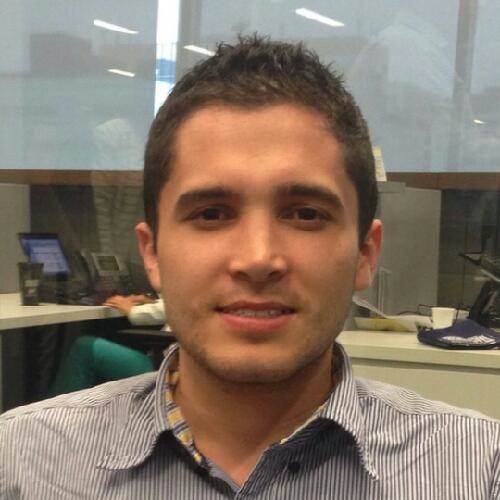
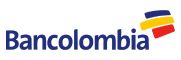