AI Development and Predictive Maintenance in Manufacturing
Predictive maintenance is a critical aspect of modern manufacturing, aimed at predicting equipment failures before they occur to minimize downtime and reduce costs. Artificial Intelligence (AI) plays a pivotal role in this process by analyzing data from various sources to forecast maintenance needs. This blog delves into how AI can be utilized for predictive maintenance in manufacturing, providing practical examples and approaches for integrating AI into your maintenance strategies.
Understanding Predictive Maintenance
Predictive maintenance involves using data analysis and machine learning to predict when equipment is likely to fail, allowing for timely maintenance interventions. By leveraging AI, manufacturers can optimize maintenance schedules, extend equipment lifespan, and reduce unexpected breakdowns, ultimately enhancing operational efficiency.
Using AI for Predictive Maintenance
AI technologies, such as machine learning algorithms and data analytics, are essential for developing predictive maintenance solutions. Below are key aspects and code examples illustrating how AI can be applied to predictive maintenance in manufacturing.
1. Data Collection and Preparation
The first step in implementing AI for predictive maintenance is collecting and preparing data from equipment sensors, logs, and other sources.
Example: Collecting Sensor Data
Assuming you have sensor data stored in a CSV file, you can use Python’s pandas library to load and preprocess this data for analysis:
```python import pandas as pd # Load sensor data data = pd.read_csv('sensor_data.csv') # Display the first few rows of the dataset print(data.head()) ```
2. Building Predictive Models
Machine learning models can be trained to predict equipment failures based on historical data. Common models used in predictive maintenance include regression models, classification algorithms, and time series forecasting.
Example: Predictive Maintenance with Random Forest
Here’s how you might build a predictive model using the Random Forest algorithm to classify equipment failure:
```python from sklearn.ensemble import RandomForestClassifier from sklearn.model_selection import train_test_split from sklearn.metrics import accuracy_score # Prepare the data X = data.drop('failure', axis=1) # Features y = data['failure'] # Target variable # Split the data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42) # Train the model model = RandomForestClassifier() model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test) # Evaluate the model accuracy = accuracy_score(y_test, predictions) print(f'Accuracy: {accuracy}') ```
3. Analyzing and Interpreting Results
Interpreting the results of your predictive models is crucial for making informed maintenance decisions. AI can help identify patterns and anomalies that indicate potential equipment failures.
Example: Analyzing Feature Importances
Here’s how you can analyze feature importances to understand which factors most influence equipment failure:
```python import matplotlib.pyplot as plt # Get feature importances importances = model.feature_importances_ # Plot feature importances plt.figure(figsize=(10, 6)) plt.barh(X.columns, importances) plt.xlabel('Importance') plt.title('Feature Importances') plt.show() ```
4. Implementing Real-Time Monitoring
AI can be used for real-time monitoring of equipment to predict failures as they occur. This involves integrating AI models with live sensor data streams.
Example: Real-Time Anomaly Detection
Here’s how you might use an AI model to detect anomalies in real-time sensor data:
```python import numpy as np # Simulate real-time sensor data new_data = np.array([sensor_reading1, sensor_reading2, ...]).reshape(1, -1) # Predict failure failure_prediction = model.predict(new_data) print(f'Failure Prediction: {failure_prediction}') ```
5. Visualizing Predictive Maintenance Insights
Visualization tools can help present insights from predictive maintenance analyses effectively. AI can assist in generating visual reports and dashboards to track equipment health and maintenance schedules.
Example: Creating a Maintenance Dashboard
Here’s how you might use Python’s Plotly library to create an interactive dashboard for monitoring equipment status:
```python import plotly.graph_objects as go # Sample data equipment_ids = ['Equipment A', 'Equipment B', 'Equipment C'] failure_probabilities = [0.1, 0.5, 0.3] # Create bar chart fig = go.Figure(data=[go.Bar(x=equipment_ids, y=failure_probabilities)]) fig.update_layout(title='Equipment Failure Probabilities', xaxis_title='Equipment', yaxis_title='Failure Probability') fig.show() ```
Conclusion
AI offers powerful capabilities for predictive maintenance in manufacturing, enabling organizations to anticipate equipment failures, optimize maintenance schedules, and improve operational efficiency. By leveraging AI for data collection, model building, real-time monitoring, and visualization, manufacturers can enhance their maintenance strategies and reduce unexpected downtimes.
Further Reading:
Table of Contents
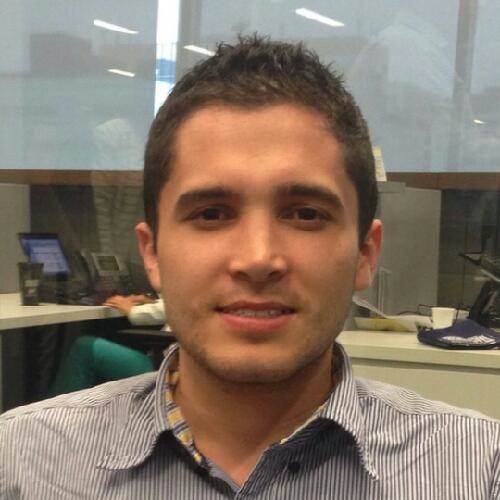
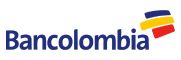