Building Recommendation Systems with AI and Python
In today’s digital age, personalized experiences are more important than ever. Whether you’re browsing your favorite streaming platform, shopping online, or using a content aggregator, you’re likely to encounter recommendation systems at work. These systems, powered by artificial intelligence and implemented using Python, play a pivotal role in enhancing user experiences by suggesting items that align with users’ preferences and behaviors. In this article, we’ll delve into the world of recommendation systems, exploring their importance, different approaches, and how to build one using Python.
1. Understanding Recommendation Systems
1.1. What are Recommendation Systems?
Recommendation systems, often referred to as recommender systems, are AI-driven tools designed to provide users with personalized suggestions. These suggestions can be in the form of movies, products, music, articles, or any other content relevant to the user’s interests. These systems have become integral to various online platforms, helping users discover new content while boosting engagement and customer satisfaction.
1.2. Importance of Recommendation Systems
- Personalization: One-size-fits-all approaches are outdated. Users expect tailored experiences that cater to their preferences. Recommendation systems leverage AI to understand user behavior and preferences, offering content that aligns with their tastes.
- Increased Engagement: By presenting users with content they’re likely to enjoy, recommendation systems keep users engaged and encourage longer interactions with the platform.
- Discoverability: Users might not be aware of all the content available to them. Recommendation systems introduce users to new and relevant items, expanding their horizons.
2. Types of Recommendation Systems
Recommendation systems can be broadly categorized into the following types:
2.1. Collaborative Filtering
Collaborative Filtering (CF) is one of the most popular recommendation techniques. It’s based on the idea that users who have agreed on preferences in the past will continue to do so in the future. CF can be further divided into two sub-types:
- User-Based Collaborative Filtering: This approach recommends items based on the preferences of users with similar tastes. For instance, if User A and User B have similar movie preferences, the system might recommend a movie that User A has watched but User B hasn’t.
- Item-Based Collaborative Filtering: Instead of focusing on user similarity, this approach recommends items similar to those a user has already shown interest in. If a user likes Movie X, the system will recommend movies that are similar to Movie X.
2.2. Content-Based Filtering
Content-Based Filtering recommends items to users based on the features of the items themselves and the user’s historical preferences. For example, if a user has shown interest in action movies in the past, the system might recommend other action movies.
2.3. Hybrid Methods
Hybrid methods combine multiple recommendation techniques to improve accuracy and overcome limitations. For instance, a hybrid approach might blend collaborative filtering and content-based filtering to provide more accurate and diverse recommendations.
3. Building a Simple Movie Recommendation System
Let’s dive into building a basic movie recommendation system using Python. We’ll use a collaborative filtering approach for simplicity.
3.1. Collecting Data
First, we need data to work with. We’ll use a small dataset containing movie ratings. Each row will represent a user and their rating for a specific movie.
python import pandas as pd data = { 'user_id': [1, 1, 1, 2, 2], 'movie_id': [101, 102, 103, 101, 104], 'rating': [5, 4, 3, 5, 2] } df = pd.DataFrame(data)
3.2. Calculating Similarities
Next, we’ll calculate the similarity between users using a similarity metric like Pearson correlation.
python user_similarities = df.pivot_table(index='user_id', columns='movie_id', values='rating').corr()
3.3. Making Recommendations
Now, let’s make recommendations for a specific user. We’ll find movies that similar users liked but the target user hasn’t watched yet.
python def get_movie_recommendations(user_id, num_recommendations=5): user_ratings = df[df['user_id'] == user_id].set_index('movie_id')['rating'] similar_users = user_similarities[user_id].drop(user_id).dropna().sort_values(ascending=False) recommendations = [] for similar_user, similarity_score in similar_users.iteritems(): similar_user_ratings = df[df['user_id'] == similar_user].set_index('movie_id')['rating'] unseen_movies = similar_user_ratings.index.difference(user_ratings.index) similar_user_top_movies = similar_user_ratings.loc[unseen_movies].sort_values(ascending=False)[:num_recommendations] recommendations.extend(similar_user_top_movies.index) return recommendations[:num_recommendations] user_id = 1 recommendations = get_movie_recommendations(user_id) print(f"Recommended movies for user {user_id}:") for movie_id in recommendations: print(movie_id)
This simple example demonstrates the core concepts of collaborative filtering recommendation systems.
4. Taking Recommendation Systems Further with AI
While the example above gives you a taste of building a recommendation system, real-world systems are more complex and involve additional considerations:
- Scalability: Handling large datasets and ensuring quick response times is crucial.
- Matrix Factorization: Advanced techniques like matrix factorization enhance the accuracy of recommendations.
- Deep Learning: Neural networks can capture intricate patterns and improve the quality of suggestions.
Incorporating these elements requires a deep understanding of AI, machine learning, and Python programming. However, libraries like TensorFlow and scikit-learn provide tools to simplify the process.
Conclusion
Recommendation systems have revolutionized how users interact with digital platforms, making experiences more enjoyable and tailored. By utilizing AI and Python, you can create recommendation systems that not only provide value to users but also enhance engagement and satisfaction. Whether you’re diving into collaborative filtering or exploring more advanced techniques, the world of recommendation systems is ripe for exploration. So why not embark on this journey and start building your own personalized recommendation system today?
Table of Contents
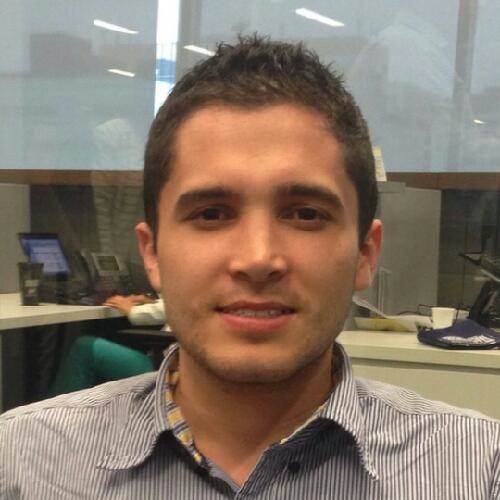
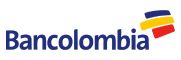