AI Development Made Easy with Scikit-Learn
Artificial Intelligence (AI) has become an essential part of various industries, revolutionizing the way we interact with technology. However, building AI models used to be a daunting task, requiring extensive knowledge of complex algorithms and programming languages. Thanks to libraries like Scikit-Learn, AI development has been made more accessible to both beginners and experts alike.
In this blog, we will delve into the world of Scikit-Learn and explore how it simplifies the AI development process. Whether you are a data scientist, engineer, or enthusiast, Scikit-Learn’s user-friendly interface and powerful capabilities will enable you to create sophisticated AI models effortlessly.
1. What is Scikit-Learn?
Scikit-Learn, also known as sklearn, is an open-source machine learning library built on top of NumPy, SciPy, and Matplotlib. It provides a wide range of machine learning algorithms, data preprocessing techniques, and evaluation metrics in a cohesive and easy-to-use package. Scikit-Learn is designed to be user-friendly, making it an ideal choice for beginners while still offering the flexibility required for advanced applications.
2. Setting up Scikit-Learn:
Before we begin, ensure you have Python and Scikit-Learn installed on your system. If you haven’t installed Scikit-Learn yet, you can do so using pip:
bash pip install scikit-learn
Once installed, you’re ready to dive into the world of AI development with Scikit-Learn.
3. Loading Data:
To work with Scikit-Learn, you need data to train and test your AI models. Scikit-Learn provides several built-in datasets for practice, making it convenient to get started. Let’s load the classic Iris dataset:
python from sklearn.datasets import load_iris iris = load_iris() X, y = iris.data, iris.target
The Iris dataset consists of four features (sepal length, sepal width, petal length, and petal width) and three classes (Iris Setosa, Iris Versicolor, and Iris Virginica).
4. Data Preprocessing:
Data preprocessing is a crucial step in AI development to ensure the data is in the right format and contains no missing values. Scikit-Learn offers various preprocessing functions to handle these tasks.
1) Splitting Data:
Before preprocessing, we split our data into training and testing sets:
python from sklearn.model_selection import train_test_split X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
2) Feature Scaling:
Many machine learning algorithms perform better when features are scaled. Scikit-Learn provides StandardScaler and MinMaxScaler for this purpose:
python from sklearn.preprocessing import StandardScaler scaler = StandardScaler() X_train_scaled = scaler.fit_transform(X_train) X_test_scaled = scaler.transform(X_test)
5. Choosing a Model:
Scikit-Learn offers a vast collection of machine learning algorithms, making it essential to choose the right model for your task. Here, we’ll use a simple yet powerful algorithm, the Support Vector Machine (SVM), for classification:
python from sklearn.svm import SVC svm_classifier = SVC(kernel='linear')
6. Training the Model:
Once the data is prepared, and the model is chosen, we can train the SVM classifier using the training data:
python svm_classifier.fit(X_train_scaled, y_train)
7. Evaluating the Model:
Evaluation is a critical step in the AI development process to understand how well the model performs on unseen data. Scikit-Learn provides various metrics for model evaluation, such as accuracy, precision, recall, and F1-score:
python from sklearn.metrics import accuracy_score, classification_report y_pred = svm_classifier.predict(X_test_scaled) accuracy = accuracy_score(y_test, y_pred) print("Accuracy:", accuracy) classification_rep = classification_report(y_test, y_pred) print("Classification Report:\n", classification_rep)
8. Hyperparameter Tuning:
To maximize the model’s performance, you can tune its hyperparameters. Scikit-Learn offers tools like GridSearchCV to perform an exhaustive search over specified hyperparameter values:
python from sklearn.model_selection import GridSearchCV param_grid = {'C': [0.1, 1, 10], 'kernel': ['linear', 'rbf']} grid_search = GridSearchCV(svm_classifier, param_grid, cv=5) grid_search.fit(X_train_scaled, y_train) best_params = grid_search.best_params_ print("Best Hyperparameters:", best_params)
9. Saving and Loading the Model:
After training the model and finding the best hyperparameters, you might want to save it for future use. Scikit-Learn allows you to do this using the joblib library:
python import joblib joblib.dump(grid_search.best_estimator_, 'svm_classifier.pkl')
Later, you can load the model using:
python loaded_model = joblib.load('svm_classifier.pkl')
10. Building a Pipeline:
Scikit-Learn provides a Pipeline class that allows you to chain multiple processing steps and a final estimator. This makes the process of training and deploying models much more straightforward:
python from sklearn.pipeline import Pipeline pipeline = Pipeline([ ('scaler', StandardScaler()), ('classifier', SVC()) ]) pipeline.fit(X_train, y_train)
11. Handling Imbalanced Data:
In real-world scenarios, datasets often suffer from class imbalances. Scikit-Learn offers techniques to handle such imbalanced datasets, such as resampling:
python from sklearn.utils import resample # Assuming X_train_imbalanced and y_train_imbalanced are imbalanced datasets X_resampled, y_resampled = resample(X_train_imbalanced, y_train_imbalanced, random_state=42)
12. Cross-Validation:
To evaluate your model’s generalization performance, cross-validation is a valuable technique. Scikit-Learn makes it simple with the cross_val_score function:
python from sklearn.model_selection import cross_val_score cv_scores = cross_val_score(svm_classifier, X_train_scaled, y_train, cv=5) average_cv_accuracy = cv_scores.mean() print("Average Cross-Validation Accuracy:", average_cv_accuracy)
Conclusion:
Scikit-Learn is undoubtedly a powerful and user-friendly library that simplifies AI development. With its comprehensive set of tools and algorithms, building AI models has become more accessible than ever. In this blog, we explored the process of developing AI models with Scikit-Learn, from data loading and preprocessing to model training and evaluation.
So, whether you’re a novice or an experienced data scientist, embrace Scikit-Learn and unlock the potential of AI development with ease and efficiency. Happy coding!
Table of Contents
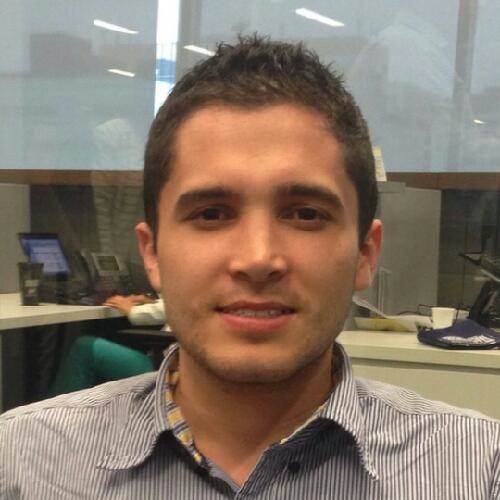
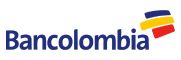