AI Development and Smart Homes: Automating Daily Life
The integration of artificial intelligence (AI) into smart homes is transforming the way we live by automating daily tasks and enhancing our living environment. AI-driven smart home systems can manage everything from lighting and temperature to security and energy consumption, making homes more efficient and user-friendly. This article explores how AI can be used in smart home technology, providing practical examples and insights into developing intelligent automation solutions.
Understanding Smart Homes
Smart homes utilize advanced technology to automate and control various household systems. By incorporating AI, these systems can learn user preferences, predict needs, and optimize home management. AI enables devices to communicate and make decisions based on data, leading to a more intuitive and responsive living experience.
Using AI for Smart Home Automation
AI offers several advantages in smart home automation, including improved efficiency, enhanced security, and personalized experiences. Below are key aspects and examples demonstrating how AI can be utilized to automate and optimize smart home systems.
1. Voice-Activated Assistants
Voice-activated assistants like Amazon Alexa and Google Assistant leverage AI to interpret and respond to user commands. These systems can control various smart devices, such as lights, thermostats, and appliances, through natural language processing.
Example: Developing a Voice Assistant Skill
Here’s an example of creating a simple voice skill for controlling smart lights using the Amazon Alexa Skills Kit (ASK).
```python from ask_sdk_core.skill_builder import SkillBuilder from ask_sdk_core.dispatch_components import AbstractRequestHandler from ask_sdk_core.utils import is_request_type from ask_sdk_model import Response class TurnOnLightsHandler(AbstractRequestHandler): def can_handle(self, handler_input): return is_request_type("IntentRequest")(handler_input) and \ handler_input.request_envelope.request.intent.name == "TurnOnLightsIntent" def handle(self, handler_input): speech_text = "Turning on the lights." # Code to interface with smart home API to turn on lights return handler_input.response_builder.speak(speech_text).response sb = SkillBuilder() sb.add_request_handler(TurnOnLightsHandler()) lambda_handler = sb.lambda_handler() ```
2. Predictive Energy Management
AI can analyze usage patterns to optimize energy consumption and reduce costs. Smart thermostats and lighting systems can adjust settings based on user behavior and environmental conditions.
Example: Energy Consumption Prediction
Here’s a basic example of using a machine learning model to predict energy consumption based on historical data.
```python import numpy as np from sklearn.linear_model import LinearRegression # Sample data: hours of usage and corresponding energy consumption X = np.array([[1], [2], [3], [4], [5]]) y = np.array([10, 15, 20, 25, 30]) model = LinearRegression() model.fit(X, y) # Predict energy consumption for 6 hours of usage predicted_consumption = model.predict([[6]]) print(f"Predicted Energy Consumption: {predicted_consumption[0]}") ```
3. Intelligent Security Systems
AI-powered security systems can detect unusual activity, recognize faces, and send alerts in real-time. Machine learning algorithms can be trained to identify patterns and distinguish between normal and suspicious behavior.
Example: Face Recognition for Security
Here’s a simplified example of using a pre-trained model for face recognition.
```python import cv2 from deepface import DeepFace def recognize_face(image_path): result = DeepFace.verify(img1_path=image_path, img2_path="known_person.jpg") if result['verified']: print("Face recognized!") else: print("Face not recognized.") recognize_face("new_person.jpg") ```
4. Automated Routine Management
AI can help automate daily routines by scheduling tasks, reminders, and notifications based on user preferences and habits. This can include adjusting lighting, setting reminders, or managing household chores.
Example: Creating a Daily Routine Scheduler
Here’s an example of a simple scheduler for daily tasks.
```python import schedule import time def morning_routine(): print("Good morning! Starting your daily routine.") def evening_routine(): print("Good evening! Wrapping up your day.") schedule.every().day.at("07:00").do(morning_routine) schedule.every().day.at("20:00").do(evening_routine) while True: schedule.run_pending() time.sleep(1) ```
Conclusion
AI has the potential to revolutionize smart homes by automating daily tasks, enhancing security, and improving energy efficiency. By integrating AI technologies, homeowners can enjoy a more convenient, secure, and efficient living environment. Leveraging AI for smart home automation can lead to a more intuitive and responsive home that adapts to your needs and preferences.
Further Reading:
Table of Contents
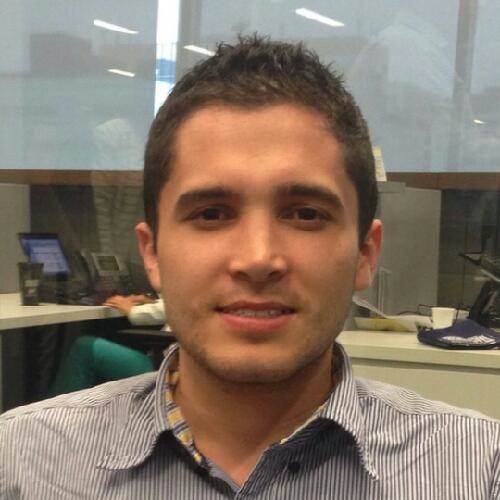
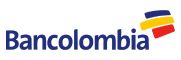