AI Development and Smart Retail: Transforming the Shopping Experience
Understanding Smart Retail
Smart retail leverages advanced technologies like AI, IoT, and big data to enhance the shopping experience. By integrating AI into retail operations, businesses can better understand customer behavior, streamline processes, and offer personalized experiences. This article explores the role of AI in smart retail and provides practical examples of how AI is transforming the shopping experience.
AI-Powered Customer Personalization
AI enables retailers to analyze vast amounts of customer data to create personalized shopping experiences. By understanding individual preferences and behaviors, AI can recommend products, tailor promotions, and enhance customer satisfaction.
Example: Personalized Product Recommendations
Imagine a customer browsing an online store. AI algorithms can analyze the customer’s browsing history, purchase patterns, and preferences to suggest products they are likely to purchase.
```csharp using System; using System.Collections.Generic; using System.Linq; class ProductRecommender { public class Product { public string Name { get; set; } public string Category { get; set; } public double Rating { get; set; } } public static List<Product> RecommendProducts(List<Product> products, string customerPreference) { return products .Where(p => p.Category.Equals(customerPreference, StringComparison.OrdinalIgnoreCase)) .OrderByDescending(p => p.Rating) .Take(5) .ToList(); } static void Main() { var products = new List<Product> { new Product { Name = "Smartphone", Category = "Electronics", Rating = 4.8 }, new Product { Name = "Laptop", Category = "Electronics", Rating = 4.7 }, new Product { Name = "Sneakers", Category = "Fashion", Rating = 4.5 }, new Product { Name = "Jeans", Category = "Fashion", Rating = 4.3 }, }; var recommendedProducts = RecommendProducts(products, "Electronics"); foreach (var product in recommendedProducts) { Console.WriteLine($"Recommended: {product.Name}, Rating: {product.Rating}"); } } } ```
Enhancing Supply Chain Efficiency with AI
AI can optimize supply chains by predicting demand, managing inventory, and reducing waste. This leads to cost savings and ensures that products are available when customers need them.
Example: Predicting Product Demand
Retailers can use AI to forecast demand for specific products, helping them to maintain optimal inventory levels and avoid stockouts or overstock situations.
```csharp using System; using System.Collections.Generic; using System.Linq; class DemandPredictor { public class SalesData { public DateTime Date { get; set; } public int QuantitySold { get; set; } } public static double PredictNextWeekSales(List<SalesData> salesHistory) { // Simple moving average prediction return salesHistory .OrderByDescending(s => s.Date) .Take(7) .Average(s => s.QuantitySold); } static void Main() { var salesHistory = new List<SalesData> { new SalesData { Date = DateTime.Now.AddDays(-1), QuantitySold = 100 }, new SalesData { Date = DateTime.Now.AddDays(-2), QuantitySold = 150 }, new SalesData { Date = DateTime.Now.AddDays(-3), QuantitySold = 120 }, }; var predictedSales = PredictNextWeekSales(salesHistory); Console.WriteLine($"Predicted sales for next week: {predictedSales}"); } } ```
AI-Driven Customer Engagement
AI chatbots and virtual assistants are becoming essential tools in retail. They provide instant support, answer queries, and guide customers through their shopping journey, significantly enhancing customer engagement.
Example: Implementing a Basic AI Chatbot
A simple AI chatbot can be developed to assist customers with common queries, such as product information, order status, and return policies.
```csharp using System; class Chatbot { public static string RespondToCustomer(string query) { if (query.Contains("order status", StringComparison.OrdinalIgnoreCase)) { return "Your order is being processed and will be delivered soon."; } else if (query.Contains("return policy", StringComparison.OrdinalIgnoreCase)) { return "You can return any item within 30 days of purchase."; } else { return "I'm here to help with any questions you have."; } } static void Main() { Console.WriteLine("Customer: What's the order status?"); Console.WriteLine("Chatbot: " + RespondToCustomer("What's the order status?")); Console.WriteLine("Customer: What's your return policy?"); Console.WriteLine("Chatbot: " + RespondToCustomer("What's your return policy?")); } } ```
Visualizing Retail Data with AI
Visualizing sales trends, customer behavior, and other data points is essential for making informed decisions. AI can be combined with visualization tools to present data in an easily understandable format.
Example: Creating a Sales Trend Chart
By integrating AI with data visualization libraries, retailers can create charts that illustrate sales trends over time, helping them make strategic decisions.
```csharp using OxyPlot; using OxyPlot.Series; using System; using System.Collections.Generic; class Program { static void Main() { var plotModel = new PlotModel { Title = "Weekly Sales Trends" }; var series = new LineSeries { Title = "Sales" }; // Sample data var data = new List<KeyValuePair<DateTime, int>> { new KeyValuePair<DateTime, int>(DateTime.Now.AddDays(-7), 120), new KeyValuePair<DateTime, int>(DateTime.Now.AddDays(-6), 150), new KeyValuePair<DateTime, int>(DateTime.Now.AddDays(-5), 100), }; foreach (var point in data) { series.Points.Add(new DataPoint(DateTimeAxis.ToDouble(point.Key), point.Value)); } plotModel.Series.Add(series); // Code to render the plot would go here } } ```
Integrating AI with Retail Analytics
Retailers can enhance their analytics capabilities by integrating AI solutions that analyze customer behavior, predict trends, and optimize marketing strategies.
Example: Analyzing Customer Sentiment
AI can be used to analyze customer reviews and feedback, providing insights into customer sentiment and helping retailers to improve their offerings.
```csharp using System; using System.Collections.Generic; using System.Linq; class SentimentAnalyzer { public static string AnalyzeSentiment(string review) { if (review.Contains("love", StringComparison.OrdinalIgnoreCase) || review.Contains("great", StringComparison.OrdinalIgnoreCase)) { return "Positive"; } else if (review.Contains("hate", StringComparison.OrdinalIgnoreCase) || review.Contains("bad", StringComparison.OrdinalIgnoreCase)) { return "Negative"; } else { return "Neutral"; } } static void Main() { var reviews = new List<string> { "I love this product!", "This is the worst shopping experience I've ever had.", "It's okay, but could be better." }; foreach (var review in reviews) { Console.WriteLine($"Review: {review}, Sentiment: {AnalyzeSentiment(review)}"); } } } ```
Conclusion
AI is playing a pivotal role in transforming the retail industry, from enhancing customer personalization to optimizing supply chains and improving customer engagement. By leveraging AI-driven solutions, retailers can offer a more seamless and personalized shopping experience, ultimately leading to increased customer satisfaction and loyalty.
Further Reading:
Table of Contents
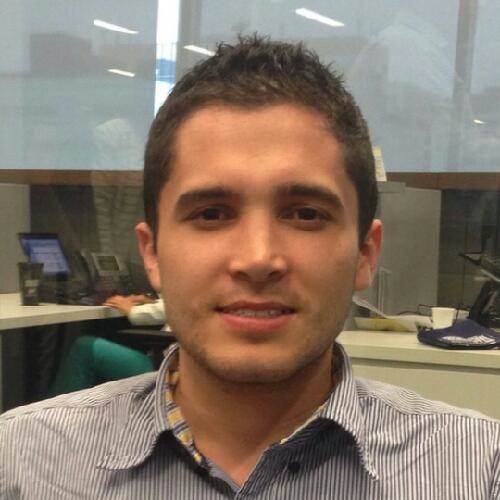
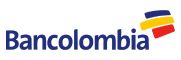