AI Development and Social Media Analytics: Understanding User Behavior
Social media has become a vital part of modern life, generating vast amounts of data that can provide deep insights into user behavior and trends. Artificial Intelligence (AI) plays a crucial role in analyzing this data, helping businesses and organizations make data-driven decisions. In this blog, we’ll explore how AI can be used to analyze social media data, understand user behavior, and provide actionable insights. We’ll cover practical examples and approaches for integrating AI into social media analytics.
Understanding Social Media Analytics
Social media analytics involves examining data from social media platforms to gain insights into user behavior, preferences, and trends. By leveraging AI, organizations can automate the analysis of large datasets, identify patterns, and derive actionable insights to enhance their marketing strategies, customer engagement, and overall business performance.
Using AI for Social Media Data Analysis
AI technologies, including machine learning and natural language processing (NLP), are essential for analyzing social media data effectively. Below are some key aspects and code examples demonstrating how AI can be utilized for social media analytics.
-
Collecting and Preprocessing Social Media Data
The first step in social media analytics is collecting and preprocessing data. AI tools can help in gathering data from various social media platforms and preparing it for analysis.
Example: Collecting Tweets Using Tweepy
Assume you want to collect tweets related to a specific topic using Python and the Tweepy library. Here’s a basic example:
```python import tweepy # Twitter API credentials consumer_key = 'YOUR_CONSUMER_KEY' consumer_secret = 'YOUR_CONSUMER_SECRET' access_token = 'YOUR_ACCESS_TOKEN' access_token_secret = 'YOUR_ACCESS_TOKEN_SECRET' # Authenticate to Twitter auth = tweepy.OAuthHandler(consumer_key, consumer_secret) auth.set_access_token(access_token, access_token_secret) api = tweepy.API(auth) # Collect tweets query = 'AI technology' tweets = api.search(q=query, count=100) # Print tweet texts for tweet in tweets: print(tweet.text) ```
-
Analyzing Sentiment
Sentiment analysis helps in understanding user sentiments and opinions expressed on social media. AI models can classify text as positive, negative, or neutral.
Example: Sentiment Analysis Using VADER
Here’s how you might analyze tweet sentiments using the VADER sentiment analysis tool in Python:
```python from vaderSentiment.vaderSentiment import SentimentIntensityAnalyzer # Initialize VADER sentiment analyzer analyzer = SentimentIntensityAnalyzer() # Analyze sentiment of a tweet tweet_text = "AI is transforming the tech industry!" sentiment_score = analyzer.polarity_scores(tweet_text) print(f"Sentiment Score: {sentiment_score}") ```
-
Identifying Trends and Patterns
AI can help identify trends and patterns in social media conversations by analyzing large volumes of data. This includes detecting emerging topics and user behavior trends.
Example: Topic Modeling Using LDA
Here’s a simple example of topic modeling using Latent Dirichlet Allocation (LDA) to discover topics in a collection of tweets:
```python from sklearn.feature_extraction.text import CountVectorizer from sklearn.decomposition import LatentDirichletAllocation # Sample tweet texts tweets = ["AI is the future of technology.", "Machine learning is evolving rapidly.", "Deep learning algorithms are powerful."] # Convert text to bag-of-words model vectorizer = CountVectorizer(stop_words='english') X = vectorizer.fit_transform(tweets) # Apply LDA lda = LatentDirichletAllocation(n_components=2, random_state=0) lda.fit(X) # Print topics for idx, topic in enumerate(lda.components_): print(f"Topic {idx}:") print([vectorizer.get_feature_names_out()[i] for i in topic.argsort()[-5:]]) ```
-
Visualizing Social Media Insights
Visualization tools help in presenting social media data insights effectively. AI can assist in generating visual representations of trends and patterns.
Example: Creating a Word Cloud
Here’s how you can create a word cloud to visualize frequently used words in social media posts:
```python from wordcloud import WordCloud import matplotlib.pyplot as plt # Sample text text = "AI machine learning deep learning artificial intelligence technology" # Generate word cloud wordcloud = WordCloud(width=800, height=400, background_color='white').generate(text) # Display word cloud plt.figure(figsize=(10, 5)) plt.imshow(wordcloud, interpolation='bilinear') plt.axis('off') plt.show() ```
-
Integrating with Social Media APIs
AI can be integrated with social media APIs to fetch real-time data and analyze it continuously.
Example: Fetching Data from a Social Media API
Here’s how you might fetch and analyze data from a social media API (e.g., Twitter API) using Python:
```python import requests # Twitter API endpoint url = 'https://api.twitter.com/2/tweets/search/recent' params = {'query': 'AI technology'} headers = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'}
Conclusion
AI offers powerful tools for analyzing social media data, helping organizations gain valuable insights into user behavior and trends. From collecting and preprocessing data to performing sentiment analysis, identifying trends, and visualizing insights, AI can significantly enhance social media analytics capabilities. Leveraging these technologies will lead to more informed decision-making and effective strategies in the ever-evolving digital landscape.
Further Reading:
Table of Contents
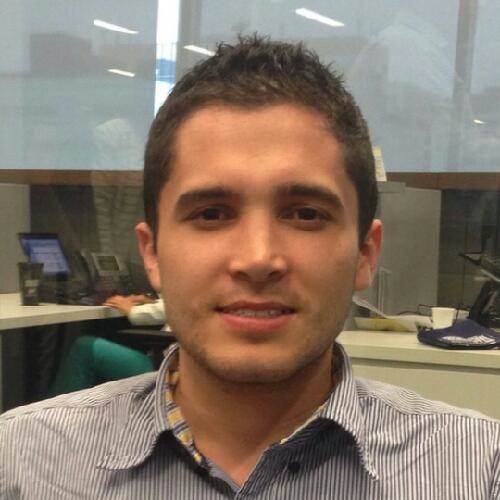
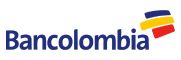