Transforming Business with AI: Applications and Case Studies
In today’s fast-paced and technology-driven world, businesses are constantly seeking innovative ways to stay competitive and efficient. One technology that has been at the forefront of this transformation is Artificial Intelligence (AI). From automating routine tasks to making complex decisions, AI has the potential to reshape industries and revolutionize the way businesses operate. In this article, we will delve into the various applications of AI in business and explore real-world case studies that demonstrate its transformative power.
1. AI-powered Customer Insights
1.1. Enhancing Personalization with Recommendation Systems
Recommendation systems are a prime example of AI’s impact on customer experiences. E-commerce giants like Amazon and streaming platforms like Netflix utilize AI algorithms to analyze user preferences and behaviors, enabling them to offer tailored product suggestions and content recommendations. Let’s take a look at a simple collaborative filtering recommendation system using Python:
python import numpy as np from sklearn.metrics.pairwise import cosine_similarity # User-item matrix (rows: users, columns: items) user_item_matrix = np.array([[1, 0, 3, 4], [2, 1, 0, 3], [0, 4, 2, 0]]) # Calculate cosine similarity item_similarity = cosine_similarity(user_item_matrix.T) # Generate recommendations for a user user_id = 0 user_ratings = user_item_matrix[user_id] recommendations = np.dot(item_similarity, user_ratings) / np.sum(np.abs(item_similarity), axis=1) print("Recommendations:", recommendations)
1.2. Sentiment Analysis for Better Customer Understanding
Understanding customer sentiments is crucial for businesses to improve products and services. Sentiment analysis, a natural language processing technique, helps in gauging public opinions from social media, reviews, and other textual data. Python’s NLTK library can be used to perform sentiment analysis:
python from nltk.sentiment import SentimentIntensityAnalyzer text = "The new product exceeded my expectations. The quality is impressive!" analyzer = SentimentIntensityAnalyzer() sentiment_scores = analyzer.polarity_scores(text) if sentiment_scores['compound'] > 0: sentiment = "positive" elif sentiment_scores['compound'] < 0: sentiment = "negative" else: sentiment = "neutral" print("Sentiment:", sentiment)
2. Operational Efficiency through AI
2.1. Supply Chain Optimization using Predictive Analytics
AI-powered predictive analytics enables businesses to forecast demand, optimize inventory, and enhance supply chain efficiency. This has a significant impact on cost reduction and customer satisfaction. Let’s consider a simplified example of demand forecasting using linear regression:
python import pandas as pd from sklearn.linear_model import LinearRegression from sklearn.model_selection import train_test_split # Load the dataset data = pd.read_csv("sales_data.csv") # Prepare data X = data[['previous_sales', 'marketing_spend']] y = data['current_sales'] # Split data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create and train the model model = LinearRegression() model.fit(X_train, y_train) # Predict sales predicted_sales = model.predict(X_test)
2.2. Streamlining HR Operations with Chatbots
Human Resources departments can benefit from AI-powered chatbots that handle employee queries, provide onboarding assistance, and schedule interviews. These chatbots utilize natural language processing to understand and respond to employee inquiries. Here’s a basic example using the Rasa framework:
python # Install Rasa pip install rasa # Create a simple Rasa chatbot rasa init # Train the chatbot with data rasa train # Start the chatbot rasa shell
3. AI-driven Marketing Strategies
3.1. Precision Targeting through Predictive Analytics
AI enables marketers to segment audiences effectively and personalize marketing campaigns. Predictive analytics can help identify potential high-value customers and optimize marketing strategies accordingly. Let’s use K-means clustering for audience segmentation:
python import pandas as pd from sklearn.cluster import KMeans from sklearn.preprocessing import StandardScaler # Load the dataset data = pd.read_csv("customer_data.csv") # Prepare data X = data[['age', 'income']] scaler = StandardScaler() X_scaled = scaler.fit_transform(X) # Perform K-means clustering num_clusters = 3 kmeans = KMeans(n_clusters=num_clusters, random_state=42) data['cluster'] = kmeans.fit_predict(X_scaled)
3.2. Content Generation with Natural Language Processing
AI-driven content generation tools are revolutionizing marketing efforts. Natural Language Processing models can produce blog posts, social media captions, and even product descriptions. The GPT-3 model from OpenAI is a prime example:
python # Use the OpenAI API for text generation import openai openai.api_key = "your_api_key" prompt = "Write a blog post about the benefits of AI in marketing." response = openai.Completion.create( engine="davinci", prompt=prompt, max_tokens=200 ) generated_text = response.choices[0].text print(generated_text)
4. Financial Decision Making with AI
4.1. Algorithmic Trading in the Stock Market
AI algorithms have revolutionized stock trading by analyzing vast amounts of data to make quick trading decisions. Machine learning models can predict stock price movements and execute trades accordingly. Here’s a simple moving average crossover strategy using Python:
python import pandas as pd import numpy as np import matplotlib.pyplot as plt # Load historical stock data data = pd.read_csv("stock_data.csv") data['Date'] = pd.to_datetime(data['Date']) data.set_index('Date', inplace=True) # Calculate moving averages data['50_MA'] = data['Close'].rolling(window=50).mean() data['200_MA'] = data['Close'].rolling(window=200).mean() # Implement trading strategy data['Signal'] = np.where(data['50_MA'] > data['200_MA'], 1, -1) # Visualize strategy plt.figure(figsize=(12, 6)) plt.plot(data.index, data['Close'], label='Stock Price') plt.plot(data.index, data['50_MA'], label='50-day MA') plt.plot(data.index, data['200_MA'], label='200-day MA') plt.plot(data[data['Signal'] == 1].index, data[data['Signal'] == 1]['Close'], '^', markersize=10, color='g', label='Buy Signal') plt.plot(data[data['Signal'] == -1].index, data[data['Signal'] == -1]['Close'], 'v', markersize=10, color='r', label='Sell Signal') plt.legend() plt.show()
4.2. Fraud Detection and Prevention
Financial institutions employ AI to detect fraudulent activities in real-time. Machine learning models can learn from historical data to identify unusual patterns and behaviors. Let’s consider a basic example of credit card fraud detection using a random forest classifier:
python import pandas as pd from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier from sklearn.metrics import accuracy_score, confusion_matrix # Load the dataset data = pd.read_csv("credit_card_data.csv") # Prepare data X = data.drop('is_fraud', axis=1) y = data['is_fraud'] # Split data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Create and train the model model = RandomForestClassifier(random_state=42) model.fit(X_train, y_train) # Predict fraud predictions = model.predict(X_test) # Evaluate the model accuracy = accuracy_score(y_test, predictions) conf_matrix = confusion_matrix(y_test, predictions)
5. The Healthcare Revolution with AI
5.1. Diagnostics and Medical Imaging Advancements
AI is making significant strides in medical diagnostics, particularly in medical imaging interpretation. Deep learning models can analyze X-rays, MRIs, and CT scans to identify diseases and anomalies. A classic example is using a convolutional neural network (CNN) for image classification:
python import tensorflow as tf from tensorflow.keras.preprocessing.image import ImageDataGenerator from tensorflow.keras.applications import VGG16 from tensorflow.keras.layers import Dense, GlobalAveragePooling2D from tensorflow.keras.models import Model # Load and preprocess image data datagen = ImageDataGenerator(rescale=1.0/255.0, validation_split=0.2) train_generator = datagen.flow_from_directory('medical_images', subset='training', ...) valid_generator = datagen.flow_from_directory('medical_images', subset='validation', ...) # Load pre-trained VGG16 model base_model = VGG16(weights='imagenet', include_top=False) # Add custom layers for classification x = base_model.output x = GlobalAveragePooling2D()(x) x = Dense(512, activation='relu')(x) predictions = Dense(num_classes, activation='softmax')(x) model = Model(inputs=base_model.input, outputs=predictions) # Compile and train the model model.compile(optimizer='adam', loss='categorical_crossentropy', metrics=['accuracy']) model.fit(train_generator, validation_data=valid_generator, ...)
5.2. Drug Discovery and Development Acceleration
AI is also transforming the drug discovery process by analyzing massive datasets and simulating molecular interactions. Machine learning models can predict potential drug candidates and optimize their properties. Here’s a simplified example of using a generative adversarial network (GAN) for molecular design:
python import numpy as np import tensorflow as tf from tensorflow.keras.layers import Dense, Reshape from tensorflow.keras.models import Sequential # Generate random molecular structures def generate_molecules(num_molecules, latent_dim): generator = Sequential([ Dense(128, input_dim=latent_dim, activation='relu'), Dense(256, activation='relu'), Dense(num_features, activation='sigmoid'), Reshape((num_features,)) ]) molecules = generator(np.random.randn(num_molecules, latent_dim)) return molecules num_molecules = 100 latent_dim = 50 num_features = 100 molecules = generate_molecules(num_molecules, latent_dim)
6. Ethical Considerations in AI Adoption
While AI offers immense potential, its adoption raises ethical concerns. Bias in AI algorithms, data privacy, and job displacement are some of the challenges that need careful consideration. Businesses must prioritize responsible AI development and ensure transparency and fairness in their AI applications.
Conclusion
Artificial Intelligence is reshaping the business landscape across various domains, from customer insights and operational efficiency to marketing strategies and financial decision-making. Real-world case studies showcased how AI-powered recommendation systems, sentiment analysis, predictive analytics, chatbots, and content generation are driving innovation and transformation. In fields like healthcare and finance, AI is making groundbreaking advancements, revolutionizing diagnostics, drug discovery, and trading strategies. However, as AI adoption accelerates, ethical considerations remain paramount to harness its benefits responsibly. As businesses continue to embrace AI, staying informed about its potential and its ethical implications will be key to unlocking its true transformative power.
Table of Contents
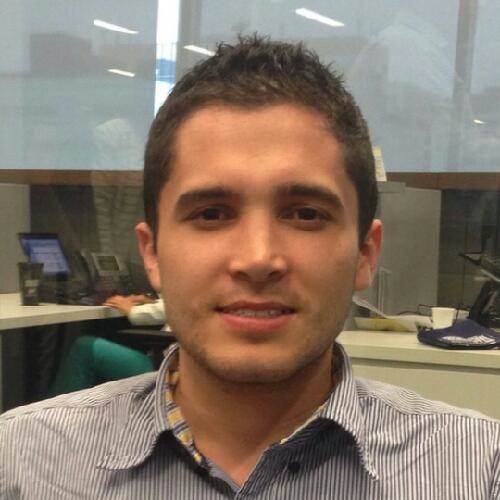
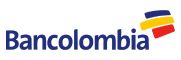