Essential Android Animation Techniques for a More Appealing UI
In the realm of Android application development, a field where skilled Android developers are highly sought after, animation stands as a vital element that adds a layer of sophistication, interactivity, and an overall elevated user experience. It not only adds visual appeal to your app but also provides fluid feedback and clear navigational guidance. If you’re looking to hire Android developers, understanding their proficiency in these techniques can be crucial. In this blog post, we will discuss various Android animation techniques, their importance, and walk through some practical examples to apply these animations to your application.
Table of Contents
1. The Importance of Android Animation
There’s a famous saying in the world of UI/UX design, “Good design is obvious. Great design is transparent.” When we apply this concept to Android applications, animations are a crucial part of “transparency.”
Animations can serve multiple purposes, such as:
- Creating a narrative: Animations can guide users through the functionalities of the application, making it intuitive and user-friendly.
- Providing feedback: They can offer visual cues to users about interactions. For instance, a button changing color or vibrating slightly when pressed.
- Enhancing visual appeal: Well-designed animations can make your app more engaging and attractive.
2. Understanding Android Animation Types
Android offers various ways to create animations. Primarily, they fall into two categories: View Animation and Property Animation.
2.1. View Animation
View Animation, also known as Tweened Animation, allows you to move, rotate, scale, and fade views. The four subclasses under this are RotateAnimation, TranslateAnimation, ScaleAnimation, and AlphaAnimation.
Example: RotateAnimation
Imagine an application where you want a button to rotate when clicked. Here’s how you might implement this using RotateAnimation:
```java Button rotateButton = findViewById(R.id.rotate_button); RotateAnimation rotateAnimation = new RotateAnimation(0f, 360f, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f); rotateAnimation.setDuration(1000); rotateAnimation.setInterpolator(new LinearInterpolator()); rotateButton.setOnClickListener(v -> v.startAnimation(rotateAnimation)); ```
In the above code, we create a RotateAnimation that rotates the view from 0 to 360 degrees around the center of the button (`0.5f, 0.5f` refer to the pivot points). It lasts for 1000 milliseconds (or 1 second) and applies a LinearInterpolator, meaning the animation speed is consistent throughout its duration.
2.2. Property Animation
The Property Animation system is a more flexible and powerful mechanism for animating objects. It allows you to animate any property of any object, not just views, over time.
Example: ObjectAnimator
Let’s say you want to fade out an image over a certain period. Here’s how to do it using ObjectAnimator:
```java ImageView imageView = findViewById(R.id.image_view); ObjectAnimator fadeOut = ObjectAnimator.ofFloat(imageView, "alpha", 1f, 0f); fadeOut.setDuration(2000); fadeOut.start(); ```
In this example, we create an ObjectAnimator that animates the “alpha” property (opacity) of an ImageView from 1 (fully visible) to 0 (fully transparent) over 2 seconds. The result is a fade-out effect.
3. Leveraging Advanced Animation Libraries
Apart from the built-in animations, numerous libraries can help you create more complex and visually appealing animations. A popular one among these is Lottie.
3.1. Lottie
Lottie is a library developed by Airbnb that parses Adobe After Effects animations exported as JSON and renders them natively on the app.
Example: Lottie Animation
To implement a Lottie animation, first, add the Lottie dependency to your `build.gradle`:
```gradle dependencies { implementation 'com.airbnb.android:lottie:3.4.0' } ```
Next, add a LottieAnimationView to your layout:
```xml <com.airbnb.lottie.LottieAnimationView android:id="@+id/lottie_animation_view" android:layout_width="wrap_content" android:layout_height="wrap_content" app:lottie_fileName="animation.json"/> ```
In this case, `animation.json` is the After Effects animation exported as a JSON file. This file should be placed in your app’s `assets` folder.
4. The Power of Animated Transitions
Another great way to add life to your UI is by using animated transitions between activities or fragments.
4.1. Transition Framework
The Transition Framework enables you to define enter and exit transitions for your activities and fragments.
Example: Shared Element Transition
Assume you have an image in Activity A, and you want it to transition to Activity B. Here’s how you can achieve this:
In `res/values/styles.xml`, enable window content transitions:
```xml <style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar"> <item name="android:windowContentTransitions">true</item> </style> ```
In Activity A, specify the transition name of the image:
```xml <ImageView android:id="@+id/image" android:transitionName="sharedImage" ... /> ```
In Activity B, specify the same transition name for the destination image:
```xml <ImageView android:id="@+id/image" android:transitionName="sharedImage" ... /> ```
Finally, when starting Activity B from Activity A, create an `ActivityOptions` bundle and pass it to `startActivity()`:
```java Intent intent = new Intent(this, ActivityB.class); ActivityOptions options = ActivityOptions.makeSceneTransitionAnimation(this, imageView, imageView.getTransitionName()); startActivity(intent, options.toBundle()); ```
The result is a seamless animated transition of the image from Activity A to Activity B.
Conclusion
In the bustling world of app development, it’s the small details that set apart good apps from great ones. This is where the expertise of those who hire Android developers really shines. Adding animations to your Android application brings a level of polish and responsiveness that users not only appreciate but expect. With the examples above, you, or the Android developers you hire, can start adding some life to your app’s user interface and ensure a memorable user experience. Animation indeed adds the ‘motion‘ to the ’emotion‘ of using an application.
To keep learning, explore the Android documentation, experiment with different animations, and learn how to use advanced animation libraries like Lottie. Whether you’re an aspiring Android developer or looking to hire Android developers, remember that the best way to learn is by doing, so don’t hesitate to get your hands dirty and start coding.
Table of Contents
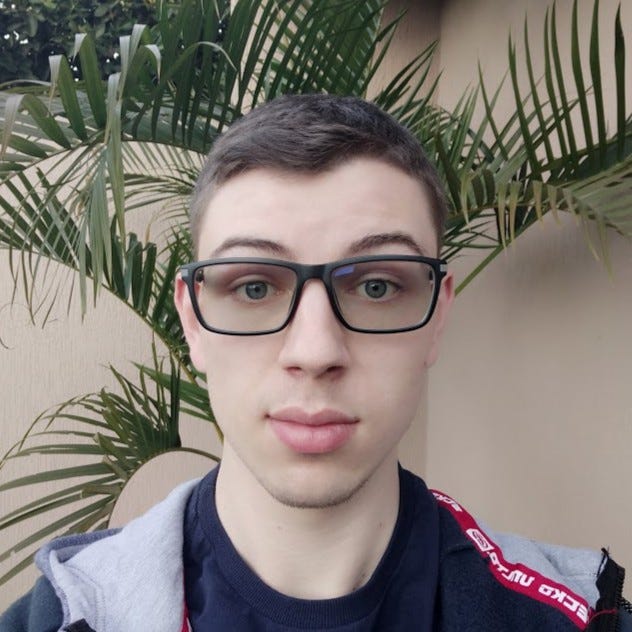
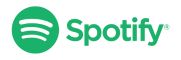