Android App Performance Monitoring: Diagnosing Bottlenecks
In today’s fast-paced digital world, Android apps have become an integral part of our daily lives. As an Android app developer, ensuring a smooth and responsive user experience is crucial to the success of your application. Performance bottlenecks can lead to frustrated users, negative reviews, and, worst of all, decreased app usage. To mitigate these issues, it is essential to implement effective Android App Performance Monitoring techniques.
In this blog, we will explore the importance of monitoring app performance and delve into diagnosing bottlenecks that may hinder your app’s efficiency. We will cover various tools and strategies to optimize your app’s performance, ensuring it runs smoothly across a wide range of devices. So, let’s dive in and discover how to unlock the full potential of your Android app!
1. Why Android App Performance Monitoring Matters?
When users interact with your Android app, they expect a seamless experience, with fast load times and smooth navigation. However, various factors can impact your app’s performance, such as device specifications, network conditions, and resource-intensive operations. By monitoring your app’s performance, you gain valuable insights into potential bottlenecks and areas for improvement.
1.1. The Impact of Poor Performance on User Experience
When an Android app is slow to load or responds sluggishly, users are likely to abandon it and switch to competitors. A recent study by Google revealed that 53% of mobile users will abandon a site if it takes longer than three seconds to load. The same principle applies to mobile apps. Poor performance can lead to:
- Reduced User Engagement: Users are less likely to engage with your app if it’s slow or unresponsive.
- Negative Reviews and Ratings: Dissatisfied users may leave negative reviews, damaging your app’s reputation.
- Decreased Retention Rates: Users are more likely to uninstall an app that consistently performs poorly.
- Lower Conversion Rates: Slow app performance can negatively impact in-app purchases and conversions.
1.2. Benefits of Android App Performance Monitoring
Implementing robust performance monitoring provides several advantages for both developers and end-users:
- Proactive Issue Detection: Performance monitoring allows you to detect and address issues before they escalate, improving user satisfaction.
- Data-Driven Optimization: Access to performance data helps you make informed decisions to optimize your app’s efficiency.
- Enhanced User Experience: By identifying and resolving bottlenecks, you deliver a smoother and more enjoyable user experience.
- Improved App Store Rankings: Apps with better performance and positive reviews are more likely to rank higher on app stores.
2. Diagnosing Performance Bottlenecks in Android Apps
Now that we understand the importance of monitoring app performance, let’s explore how to diagnose performance bottlenecks effectively.
2.1. Identifying CPU and Memory Usage
One of the primary contributors to app slowdowns is excessive CPU and memory usage. Monitoring these metrics can help pinpoint the root cause of performance issues.
java // Code Sample 1: Monitoring CPU Usage public class MyActivity extends AppCompatActivity { @Override protected void onResume() { super.onResume(); android.os.Debug.startMethodTracing("myAppTrace"); } @Override protected void onPause() { super.onPause(); android.os.Debug.stopMethodTracing(); } }
The above code snippet demonstrates how to monitor CPU usage during the execution of an activity. The data collected can be analyzed using tools like Android Profiler or Android Device Monitor.
java // Code Sample 2: Monitoring Memory Usage public class MyApplication extends Application { @Override public void onCreate() { super.onCreate(); // Get memory info ActivityManager activityManager = (ActivityManager) getSystemService(Context.ACTIVITY_SERVICE); ActivityManager.MemoryInfo memoryInfo = new ActivityManager.MemoryInfo(); activityManager.getMemoryInfo(memoryInfo); // Total available memory in MB long totalMemory = memoryInfo.totalMem / (1024 * 1024); // Memory currently available in MB long availableMemory = memoryInfo.availMem / (1024 * 1024); // Log the memory info Log.d("MemoryInfo", "Total Memory: " + totalMemory + " MB"); Log.d("MemoryInfo", "Available Memory: " + availableMemory + " MB"); } }
The code above demonstrates how to monitor memory usage in your Android application. This can help identify potential memory leaks or excessive memory consumption.
2.2. Network Performance Monitoring
In today’s interconnected world, many apps rely on network calls to fetch data. Sluggish network performance can significantly impact the overall user experience. Monitoring network performance is crucial to ensure smooth data retrieval and transmission.
java // Code Sample 3: Monitoring Network Calls public class NetworkMonitor { public static void logNetworkRequest(String url, long startTime, long endTime) { long duration = endTime - startTime; Log.d("NetworkMonitor", "URL: " + url + ", Duration: " + duration + " ms"); } }
You can use the above code to log network request durations. Implement this in your network layer to keep track of the time taken by each network call.
2.3. UI Rendering Performance
Slow UI rendering is a common cause of frustration for users. It’s crucial to monitor UI rendering to ensure smooth animations and interactions.
java // Code Sample 4: Tracing UI Rendering public class MyActivity extends AppCompatActivity { @Override protected void onResume() { super.onResume(); View rootView = getWindow().getDecorView().getRootView(); rootView.getViewTreeObserver().addOnPreDrawListener(() -> { long startTime = System.currentTimeMillis(); rootView.getViewTreeObserver().removeOnPreDrawListener(this); long endTime = System.currentTimeMillis(); long renderingDuration = endTime - startTime; Log.d("UIRendering", "UI Rendering Duration: " + renderingDuration + " ms"); return true; }); } }
The above code traces UI rendering time in an activity. By analyzing these durations, you can identify potential UI bottlenecks and take corrective actions.
3. Tools for Effective Android App Performance Monitoring
3.1. Android Profiler
Android Profiler is an essential tool for monitoring app performance. It provides real-time insights into CPU, memory, and network usage. With Android Profiler, you can:
- Monitor CPU usage and method traces to identify performance bottlenecks.
- Track memory allocations and heap usage to detect memory-related issues.
- Analyze network activity and optimize network calls for improved efficiency.
3.2. Firebase Performance Monitoring
Firebase Performance Monitoring is a powerful tool from Google that allows you to gain deeper insights into your app’s performance. It offers:
- Detailed traces for specific app functions, allowing you to measure performance metrics accurately.
- Monitoring of network requests and their durations to optimize API calls.
- Crash reporting and monitoring for faster issue resolution.
3.3. LeakCanary
Memory leaks are a common cause of app slowdowns and crashes. LeakCanary is a memory leak detection library that helps you detect and fix memory leaks in your app. It offers:
- Automatic memory leak detection during app runtime.
- Easy integration with your app to quickly identify leaky objects.
- Detailed reports to help you pinpoint the source of the memory leak.
4. Best Practices for Optimizing Android App Performance
4.1. Optimize UI Elements
Efficiently managing UI elements is crucial for a smooth user experience. Implement the following practices:
- Use RecyclerView instead of ListView for efficient item recycling.
- Employ ConstraintLayout to create flexible and responsive UIs.
- Optimize image loading with tools like Glide or Picasso.
4.2. Background Tasks and Threading
Performing resource-intensive tasks on the main thread can lead to unresponsive UIs. Consider these best practices:
- Offload heavy tasks to background threads or AsyncTask.
- Utilize the Android JobScheduler or WorkManager for background processing.
- Employ RxJava or Kotlin Coroutines for efficient and concise threading.
4.3. Data Caching and Offline Support
Optimizing data retrieval and caching can significantly improve app performance:
- Implement data caching to reduce network calls and improve data retrieval speeds.
- Offer offline support for essential app features to enhance user experience during connectivity issues.
Conclusion
Mastering Android App Performance Monitoring is essential for delivering a top-notch user experience. By proactively diagnosing bottlenecks, you can optimize your app’s performance and avoid user dissatisfaction. Utilizing tools like Android Profiler, Firebase Performance Monitoring, and LeakCanary, along with implementing best practices, will ensure your Android app runs seamlessly across diverse devices and network conditions. So, embark on this journey of performance optimization, and unlock the true potential of your Android app!
Table of Contents
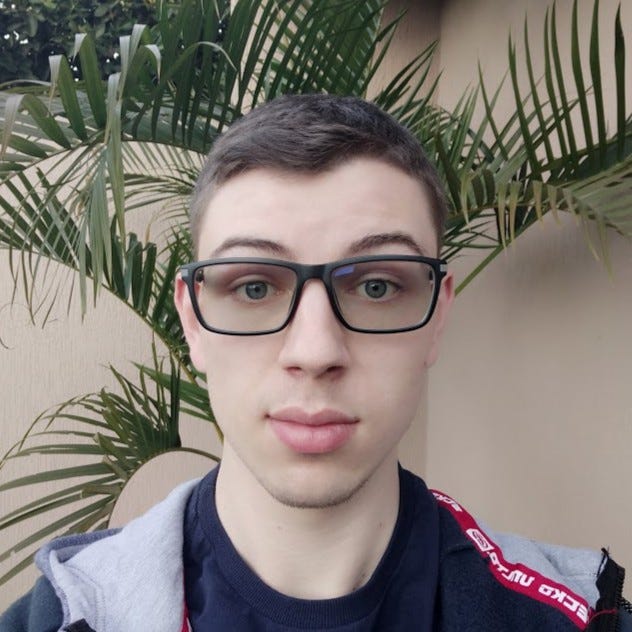
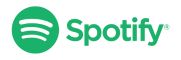