Android App Widgets: The Ultimate Tool for a Personalized Home Screen Experience
The Android operating system offers a plethora of customization options, among which App Widgets occupy a significant space. Android widgets are not just an icon on the home screen; they are interactive miniature application views that can be embedded within the Android home screen. These widgets provide quick access to the app’s main features and allow users to engage with the app without necessarily launching it.
Table of Contents
In this blog post, we’ll delve deep into the world of Android app widgets, offering insights into their creation and providing real-world examples.
1. Basics of App Widgets
App Widgets in Android are, in essence, broadcast receivers that react to broadcast messages, coupled with a user interface that can be updated at regular intervals or in response to user actions or notifications. These can be designed using layout files just like any other Android user interface.
2. Configuring the Widget Metadata
Before you start creating the widget, you need to define the widget’s metadata. This is usually stored in an XML resource in the `res/xml/` directory.
For example, if we are creating a simple clock widget:
```xml <appwidget-provider xmlns:android="http://schemas.android.com/apk/res/android" android:minWidth="146dip" android:minHeight="146dip" android:updatePeriodMillis="86400000" android:initialLayout="@layout/widget_layout" android:resizeMode="horizontal|vertical" android:widgetCategory="home_screen"> </appwidget-provider> ```
Here, `updatePeriodMillis` specifies the update interval and `initialLayout` defines the layout of the widget.
3. Creating the Widget Layout
A widget’s layout is not different from the layout of an activity or fragment. For our clock widget, it might look something like this:
```xml <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:padding="8dp"> <TextView android:id="@+id/txtClock" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:textSize="24sp" android:text="12:00"/> </RelativeLayout> ```
4. Developing the Widget Provider
The `AppWidgetProvider` is a subclass of `BroadcastReceiver`. It receives broadcasts when the widget needs to be updated.
For the clock widget:
```java public class ClockWidgetProvider extends AppWidgetProvider { @Override public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) { final int N = appWidgetIds.length; for (int i = 0; i < N; i++) { int appWidgetId = appWidgetIds[i]; RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.widget_layout); // Logic to update the TextView with the current time. appWidgetManager.updateAppWidget(appWidgetId, views); } } } ```
5. Reacting to User Actions
Widgets are often interactive. For our clock, let’s say we want to open the clock app when the user taps on it:
```java RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.widget_layout); Intent intent = new Intent(AlarmClock.ACTION_SHOW_ALARMS); PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, intent, 0); views.setOnClickPendingIntent(R.id.txtClock, pendingIntent); ```
6. Testing your Widget
Once you’ve created your widget, it’s vital to test it on different devices and screen sizes to ensure consistent behavior and appearance.
- Emulators: Use Android Studio’s emulator suite to simulate different devices and screen sizes.
- Real Devices: Deploy your widget to real Android devices.
- Battery and Performance: Ensure that your widget does not drain battery or hog system resources.
7. Advanced Widgets: Stack and Collection Views
For displaying collections of data, Android widgets support structures like `ListView`, `GridView`, and `StackView`. This allows for a more complex and interactive widget experience, like an email inbox preview or a news feed.
Conclusion
Android widgets offer an enriched home screen experience, allowing users to interact with their favorite apps at a glance. Whether you’re creating a simple clock widget or a more complex news feed, the process of creating and deploying widgets is both rewarding and engaging.
With the advent of newer tools and frameworks, widget development is becoming even more streamlined. By following the principles outlined in this guide and keeping a keen eye on performance, usability, and design, you can craft beautiful widget experiences for your users.
Table of Contents
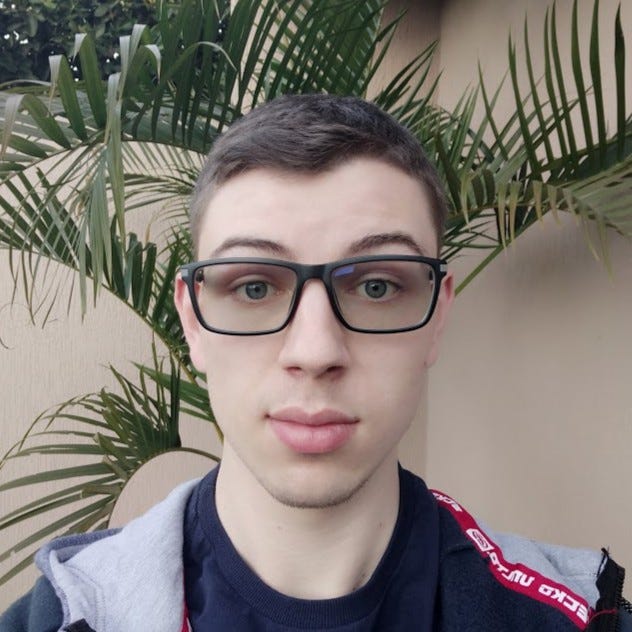
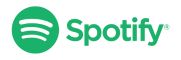