Essential Tips for Designing Interactive Android Home Screen Elements
Android’s home screen is a dynamic canvas that allows developers to offer interactive widgets, ensuring a user’s most crucial information is always at their fingertips. If you’re looking to harness this potential, it might be time to hire Android developers. Widgets, as mini-applications, offer a glimpse of the application’s key functionalities directly from the home screen. Whether it’s the weather forecast, your latest emails, or a music player, widgets give users instant access and a smoother user experience. In this post, we will delve deep into the creation of Android App Widgets and illustrate it with examples.
Table of Contents
1. Basic Components of App Widgets
Android App Widgets are made up of a few core components:
– Layouts: Defines the widget’s UI, using XML layout files.
– AppWidgetProvider: A broadcast receiver that manages widget data updates.
– Metadata: Defined in XML, specifying essential properties like update frequency and layout dimensions.
2. Creating a Simple Clock Widget
2.1. Define the Layout
Start by creating a layout XML file for the widget. In this example, we’ll create a simple digital clock.
```xml <!-- res/layout/widget_clock.xml --> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:padding="8dp" android:background="@drawable/widget_background"> <TextClock android:id="@+id/digitalClock" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:textSize="24sp" android:format12Hour="hh:mm:ss a" android:timeZone="Etc/UTC" /> </RelativeLayout> ```
2.2 Implement the AppWidgetProvider
Here, we create a BroadcastReceiver that manages widget updates.
```java public class ClockWidgetProvider extends AppWidgetProvider { @Override public void onUpdate(Context context, AppWidgetManager appWidgetManager, int[] appWidgetIds) { for (int appWidgetId : appWidgetIds) { RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.widget_clock); appWidgetManager.updateAppWidget(appWidgetId, views); } } } ```
2.3 Define the Widget Metadata
This is an XML resource in `res/xml/`.
```xml <!-- res/xml/clock_widget_info.xml --> <appwidget-provider xmlns:android="http://schemas.android.com/apk/res/android" android:minWidth="146dip" android:minHeight="72dip" android:updatePeriodMillis="60000" android:initialLayout="@layout/widget_clock" android:resizeMode="horizontal|vertical" android:widgetCategory="home_screen"> </appwidget-provider> ```
2.4. Register the Widget in the Manifest
```xml <receiver android:name=".ClockWidgetProvider" > <intent-filter> <action android:name="android.appwidget.action.APPWIDGET_UPDATE" /> </intent-filter> <meta-data android:name="android.appwidget.provider" android:resource="@xml/clock_widget_info" /> </receiver> ```
Upon completion, this widget can be added to the home screen, and it will display a simple digital clock.
3. Making Widgets Interactive
Widgets aren’t just static displays; they can interact with user touches. For example, you might want the clock widget to open the Clock app when tapped.
You can achieve this using `PendingIntent`:
```java Intent intent = new Intent(context, YourTargetActivity.class); PendingIntent pendingIntent = PendingIntent.getActivity(context, 0, intent, 0); RemoteViews views = new RemoteViews(context.getPackageName(), R.layout.widget_clock); views.setOnClickPendingIntent(R.id.digitalClock, pendingIntent); ```
Now, when users tap on the clock widget, they’ll be directed to the designated activity.
4. Updating Widgets Efficiently
Frequent widget updates can drain battery life. Thus, consider the necessity of real-time updates. In our clock example, updates every minute suffice. However, if you’re showcasing a battery widget, you only need to update when there’s a significant change in battery levels.
For infrequent updates or updates based on triggers, consider using `AlarmManager` or `WorkManager` to manage update intervals.
Conclusion
Android App Widgets provide an excellent opportunity to increase user engagement, offering direct access to an app’s essential functionalities. For businesses aiming to harness this potential, it’s a strategic move to hire Android developers. Widgets are versatile and can range from simple displays (like our clock) to complex interactive elements integrated with services, databases, and even the web. The sky’s the limit when it comes to widget design and functionality. As a developer, you hold the power to create widgets that can make users’ lives easier and more productive.
Table of Contents
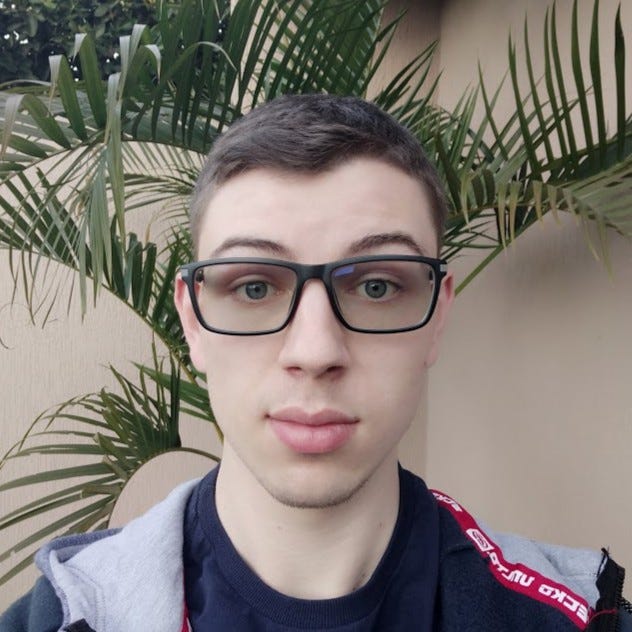
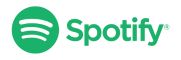