Improve Your Android Architecture with ViewModel and LiveData
Android Architecture Components were introduced to simplify app development and manage the codebase efficiently. As these components, particularly ViewModel and LiveData, have become essential in an Android developer’s toolkit, businesses looking to optimize their apps should consider hiring Android developers well-versed in these tools. This blog post will explore ViewModel and LiveData in depth, providing practical examples to illustrate their use and benefits in the app development process.
Table of Contents
1. Understanding ViewModel
The ViewModel is part of the Android Architecture Components library that manages UI-related data in a lifecycle-conscious way. It survives configuration changes such as screen rotations, enabling data to be preserved. It follows the “separation of concerns” principle by removing the UI data from the Activity or Fragment.
Example of ViewModel
Consider the case of an app that fetches user information from a server. We’ll create a ViewModel to manage the user data.
First, define the ViewModel:
```kotlin class UserViewModel : ViewModel() { private lateinit var user: User fun getUser(): User { return user } fun fetchUserFromServer(userId: String) { // Code to fetch user from server // After fetching, assign the user to the local user variable this.user = server.fetch(userId) } } ```
The ViewModel is created and attached to the lifecycle in an Activity or Fragment:
```kotlin class UserActivity : AppCompatActivity() { // Create a ViewModel the first time the system calls an activity's onCreate() method. // Re-created activities receive the same MyViewModel instance created by the first activity. val model: UserViewModel by viewModels() override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) // Fetch the user when the activity is created model.fetchUserFromServer("userId") } } ```
Now the data remains intact even after configuration changes.
2. Understanding LiveData
LiveData is an observable data holder class that is lifecycle-aware. This means it respects the lifecycle of other components, such as activities, fragments, or services, updating them when data changes. LiveData ensures your UI matches your data state.
Example of LiveData
We will continue with the UserViewModel example. Suppose you want to observe the changes in the User instance in your activities or fragments.
First, convert the user to MutableLiveData, which is a type of LiveData that can be modified:
```kotlin class UserViewModel : ViewModel() { private val _user = MutableLiveData<User>() val user: LiveData<User> get() = _user fun fetchUserFromServer(userId: String) { // Code to fetch user from server // After fetching, assign the user to the local user variable _user.value = server.fetch(userId) } } ```
Now, observe this LiveData in your Activity:
```kotlin class UserActivity : AppCompatActivity() { val model: UserViewModel by viewModels() override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) // Observe the user LiveData model.user.observe(this, Observer { user -> // update UI }) // Fetch the user when the activity is created model.fetchUserFromServer("userId") } } ```
Now, whenever the user data changes, all observers will be notified, and UI elements can be updated accordingly.
3. Combining ViewModel and LiveData
Combining ViewModel and LiveData can make your code more structured and robust. The ViewModel holds and manages the LiveData, ensuring data is preserved during configuration changes while still being able to update the UI efficiently.
The previous examples of ViewModel and LiveData combined effectively illustrate this. Fetching a user from a server, holding it in a ViewModel, observing it in an Activity using LiveData – all of this ensures a good separation of concerns and an efficient way of updating UI.
Conclusion
Android Architecture Components, specifically ViewModel and LiveData, play a crucial role in building robust and manageable Android applications. Understanding these components is an essential skill for anyone who wants to hire Android developers, as ViewModel ensures UI data survives configuration changes for a smoother user experience, and LiveData enables data changes to be observed, ensuring the UI is always correctly updated.
Whether you’re a business looking to hire Android developers, a beginner just starting with Android development, or an experienced developer aiming to improve your codebase, using ViewModel and LiveData should be a priority. With these tools in your Android arsenal, you’re well-equipped to build efficient, reliable, and robust apps.
Table of Contents
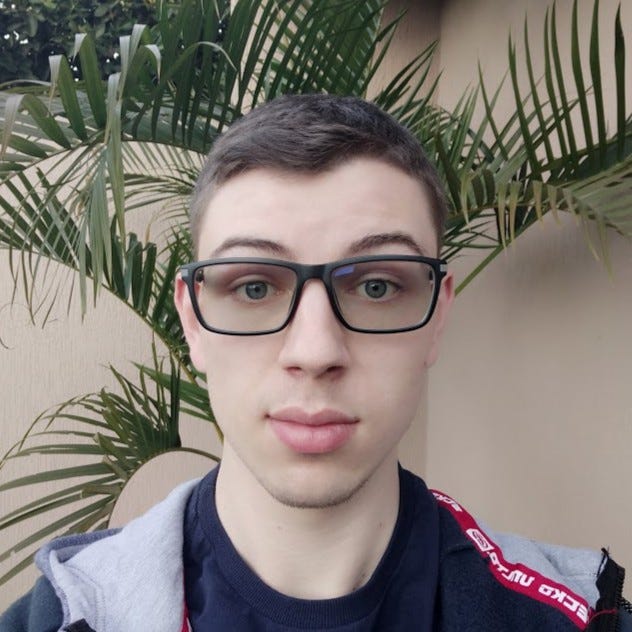
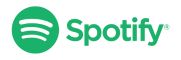