Android Audio and Video Playback: Building Multimedia Apps
In today’s world, multimedia applications have become an integral part of the Android ecosystem. From music and video streaming services to interactive gaming experiences, developers constantly strive to create captivating apps that provide seamless audio and video playback. Building such multimedia apps requires a deep understanding of Android’s audio and video APIs, along with practical implementation techniques.
In this blog, we will explore the fundamentals of Android audio and video playback, step-by-step guidelines to build multimedia apps, and code samples to illustrate key concepts. Whether you are a beginner or an experienced Android developer, this guide will help you master the art of creating engaging multimedia experiences for your users.
1. Understanding Android Audio Playback
Before diving into the development process, let’s first understand the core concepts of Android audio playback. Android provides a robust set of APIs to handle audio playback efficiently. Here are the essential components you need to know:
1.1. MediaPlayer API
The MediaPlayer class in Android allows you to play audio and video files from various sources like local storage, URLs, or raw resources. It provides methods to control playback, pause, stop, seek, and more. Below is a sample code snippet demonstrating how to play an audio file using the MediaPlayer API:
java // Initialize MediaPlayer MediaPlayer mediaPlayer = new MediaPlayer(); // Set the audio source String audioUrl = "https://example.com/audio/sample.mp3"; try { mediaPlayer.setDataSource(audioUrl); mediaPlayer.prepare(); } catch (IOException e) { e.printStackTrace(); } // Start playback mediaPlayer.start();
1.2. AudioManager API
The AudioManager class allows you to manage audio settings on the device, such as volume control, audio mode, and routing. Here’s a brief example of adjusting the media volume:
java AudioManager audioManager = (AudioManager) getSystemService(Context.AUDIO_SERVICE); audioManager.adjustStreamVolume(AudioManager.STREAM_MUSIC, AudioManager.ADJUST_RAISE, AudioManager.FLAG_SHOW_UI);
2. Building Android Audio Playback App
Now that we have a basic understanding of Android audio playback, let’s proceed to build a simple audio player app. This app will enable users to play audio from their local storage.
Step 1: Create a New Project
Open Android Studio and create a new Android project with an appropriate name and package. Choose an empty activity template to start with a clean slate.
Step 2: Design the User Interface
Design a straightforward user interface with necessary components like Play, Pause, Stop buttons, and a SeekBar to display audio progress. You can use XML or programmatically create the layout. Here’s a sample XML layout:
xml <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <Button android:id="@+id/btnPlay" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Play" /> <Button android:id="@+id/btnPause" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Pause" /> <Button android:id="@+id/btnStop" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Stop" /> <SeekBar android:id="@+id/seekBar" android:layout_width="match_parent" android:layout_height="wrap_content" /> </LinearLayout>
Step 3: Implement Audio Playback
In the activity’s Java file, initialize the MediaPlayer instance and implement the playback functionality for the Play, Pause, and Stop buttons. Also, update the SeekBar progress during playback. Here’s a snippet:
java public class AudioPlayerActivity extends AppCompatActivity { private MediaPlayer mediaPlayer; private Button btnPlay, btnPause, btnStop; private SeekBar seekBar; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_audio_player); // Initialize views btnPlay = findViewById(R.id.btnPlay); btnPause = findViewById(R.id.btnPause); btnStop = findViewById(R.id.btnStop); seekBar = findViewById(R.id.seekBar); // Initialize MediaPlayer mediaPlayer = MediaPlayer.create(this, R.raw.sample_audio); // Set up the SeekBar seekBar.setMax(mediaPlayer.getDuration()); seekBar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() { @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { if (fromUser) { mediaPlayer.seekTo(progress); } } @Override public void onStartTrackingTouch(SeekBar seekBar) {} @Override public void onStopTrackingTouch(SeekBar seekBar) {} }); // Set click listeners for buttons btnPlay.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { mediaPlayer.start(); } }); btnPause.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (mediaPlayer.isPlaying()) { mediaPlayer.pause(); } } }); btnStop.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { mediaPlayer.stop(); mediaPlayer.prepareAsync(); } }); } }
Step 4: Test the Audio Player App
Run the app on an Android device or emulator and test the audio playback functionalities. You should be able to play, pause, stop, and seek the audio using the SeekBar.
3. Understanding Android Video Playback
In addition to audio, Android also provides powerful APIs to handle video playback. The fundamental component for video playback is the VideoView, which simplifies the process of displaying videos in your app.
3.1. VideoView API
The VideoView class is a UI component in Android that can be used to play videos from various sources, such as local storage or remote URLs. It handles video playback, buffering, and display seamlessly. Below is a code snippet demonstrating how to play a video using the VideoView:
java VideoView videoView = findViewById(R.id.videoView); String videoUrl = "https://example.com/video/sample.mp4"; videoView.setVideoURI(Uri.parse(videoUrl)); videoView.start();
3.2. MediaController API
The MediaController class is used to create an overlay on top of the VideoView to provide playback controls like play, pause, seek, and volume adjustment. It provides a better user experience when interacting with videos. Here’s how to add a MediaController to the VideoView:
java MediaController mediaController = new MediaController(this); mediaController.setAnchorView(videoView); videoView.setMediaController(mediaController);
4. Building Android Video Playback App
Now, let’s move on to building a simple video player app using the VideoView and MediaController APIs.
Step 1: Create a New Project
Open Android Studio and create a new Android project with an appropriate name and package. Choose an empty activity template to begin.
Step 2: Design the User Interface
Design a clean and straightforward user interface with a VideoView and the necessary playback controls (e.g., play, pause, seek). You can use XML or create the layout programmatically. Here’s a sample XML layout:
xml <RelativeLayout android:layout_width="match_parent" android:layout_height="match_parent"> <VideoView android:id="@+id/videoView" android:layout_width="match_parent" android:layout_height="wrap_content" /> <MediaController android:id="@+id/mediaController" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignBottom="@id/videoView" android:layout_gravity="center_horizontal" /> </RelativeLayout>
Step 3: Implement Video Playback
In the activity’s Java file, initialize the VideoView and MediaController instances, and set the video source and media controller to the VideoView. Here’s a snippet:
java public class VideoPlayerActivity extends AppCompatActivity { private VideoView videoView; private MediaController mediaController; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_video_player); // Initialize views videoView = findViewById(R.id.videoView); mediaController = new MediaController(this); // Set up the MediaController mediaController.setAnchorView(videoView); videoView.setMediaController(mediaController); // Set the video source String videoUrl = "https://example.com/video/sample.mp4"; videoView.setVideoURI(Uri.parse(videoUrl)); // Start playback videoView.start(); } }
Step 4: Test the Video Player App
Run the app on an Android device or emulator and test the video playback functionalities. You should be able to play, pause, seek, and control the volume of the video using the MediaController.
Conclusion
Congratulations! You’ve learned the fundamentals of Android audio and video playback and built simple multimedia apps with both audio and video functionalities. With this knowledge, you can now explore and create more advanced multimedia applications, incorporating various media formats, streaming capabilities, and custom playback controls. Keep experimenting and enhancing your skills to deliver captivating multimedia experiences to your users.
In conclusion, the world of Android multimedia apps is vast and exciting, and as a developer, you have the power to create immersive and engaging experiences that can delight users worldwide. Happy coding!
Table of Contents
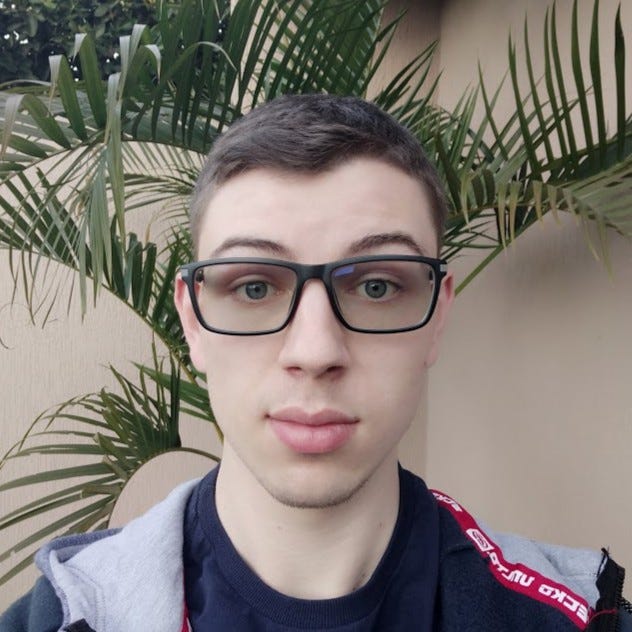
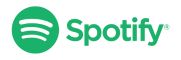