Boost App Performance with Advanced Android Image Handling Strategies
In the age of smartphones, image processing is essential. From social media apps to photo editors, images play a vital role in the user experience. However, as developers, we must ensure images do not compromise an application’s performance or lead to out-of-memory issues. In Android, a common challenge faced is handling large images, especially when loaded from the internet or high-resolution camera captures.
Table of Contents
This blog post will dive into efficient ways to handle image processing in the background and provide examples to optimize image handling.
1. Why Background Image Processing?
Processing images on the main thread can lead to a sluggish user experience or the dreaded `Application Not Responding (ANR)` dialog. To prevent this, it’s crucial to do heavy image processing in the background.
Key reasons:
- Performance: Prevent UI freeze or stutters.
- Responsiveness: Ensure app remains responsive to user actions.
- Resource Management: Optimize memory and CPU usage.
2. Methods for Background Image Processing
2.1. AsyncTask:
Introduced in Android 1.5, `AsyncTask` allows developers to perform background operations and update the UI without handling threads and handlers.
Example:
```java private class ImageLoaderTask extends AsyncTask<String, Void, Bitmap> { protected Bitmap doInBackground(String... urls) { return loadImageFromURL(urls[0]); } protected void onPostExecute(Bitmap result) { imageView.setImageBitmap(result); } } ```
However, remember that `AsyncTask` has its limitations, especially in complex scenarios or long-running operations.
2.2. Executors and Future:
With Java’s `ExecutorService`, you can create a pool of threads to run tasks concurrently. Paired with `Future`, you can obtain results once tasks are complete.
Example:
```java ExecutorService executor = Executors.newSingleThreadExecutor(); Future<Bitmap> future = executor.submit(new Callable<Bitmap>() { public Bitmap call() { return loadImageFromURL(url); } }); Bitmap image = future.get(); imageView.setImageBitmap(image); ```
2.3. RxJava:
An advanced and flexible library for composing asynchronous and event-based programs. `RxJava` can be a bit overwhelming initially but offers vast capabilities.
Example:
```java Observable.fromCallable(() -> loadImageFromURL(url)) .subscribeOn(Schedulers.io()) .observeOn(AndroidSchedulers.mainThread()) .subscribe(bitmap -> imageView.setImageBitmap(bitmap)); ```
2.4. Kotlin Coroutines:
For Kotlin developers, coroutines simplify asynchronous programming by making it more readable and manageable.
Example
GlobalScope.launch(Dispatchers.IO) { val bitmap = loadImageFromURL(url) withContext(Dispatchers.Main) { imageView.setImageBitmap(bitmap) } } ```
3. Optimizing Image Handling
3.1. Use Library:
Libraries like **Glide**, **Picasso**, and **Fresco** abstract the complexities of image loading and caching. For instance:
```java Glide.with(context) .load(url) .into(imageView); ```
3.2. Efficient Bitmap Loading:
If you’re manually handling bitmaps, you need to ensure they’re efficiently loaded, especially when dealing with high-resolution images.
3.3. Tip
Use `BitmapFactory.Options` to scale down images.
```java BitmapFactory.Options options = new BitmapFactory.Options(); options.inJustDecodeBounds = true; BitmapFactory.decodeResource(getResources(), R.id.myimage, options); int imageHeight = options.outHeight; int imageWidth = options.outWidth; String imageType = options.outMimeType; ```
4. Image Caching
Cache images to reduce redundant loading. Libraries like Glide handle this automatically, but if manually doing so, consider using an `LruCache`.
5. Handle Configuration Changes
Ensure image operations persist through configuration changes, like screen rotations. Using ViewModels or retained Fragments can be helpful.
6. Image Compression
Compress images without significantly reducing quality. For instance, JPEG compression:
```java ByteArrayOutputStream stream = new ByteArrayOutputStream(); bitmap.compress(Bitmap.CompressFormat.JPEG, 70, stream); ```
Conclusion
Optimized background image processing in Android not only ensures a buttery-smooth user experience but also efficient resource utilization. Libraries and modern tools like coroutines make this process more straightforward than ever. Always remember to test your image handling on various devices to ensure consistency and performance across the board.
Table of Contents
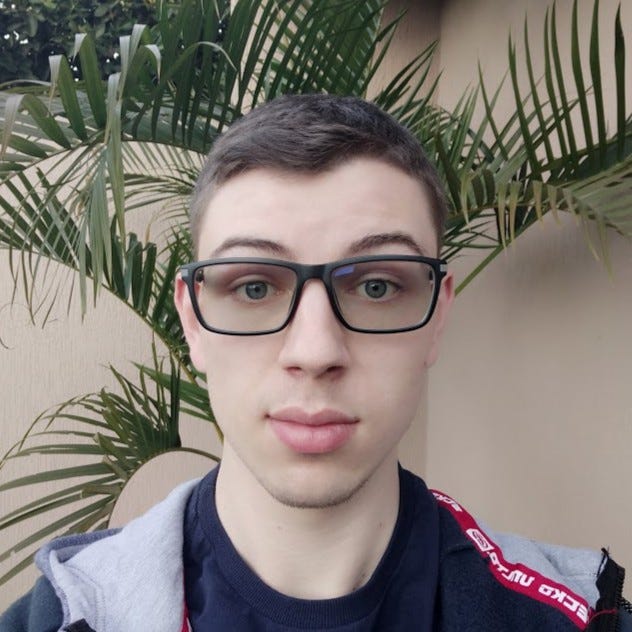
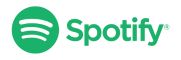