Improve Your Android App’s Performance with Threads, Handlers, and AsyncTask
Background processing is the core of any robust and efficient mobile application. Android, as a versatile platform, offers various ways to handle tasks in the background. Whether it’s downloading large files, updating the user interface, or computing heavy tasks, Android’s background processing tools ensure that these operations don’t interrupt the user experience. This is why when you hire Android developers, their expertise in these areas is a significant consideration. In this blog post, we will dive into three critical mechanisms: Threads, Handlers, and AsyncTask. These tools are part of the repertoire of any skilled Android developer. By learning how to use these tools, developers can create applications that are responsive, efficient, and most importantly, user-friendly. So, whether you’re an aspiring developer or looking to hire Android developers, understanding these background processing techniques is invaluable.
Table of Contents
1. Threads
A thread is the smallest unit of processing that can be scheduled by an operating system. In Android, threads play a pivotal role in making an application run smoothly.
1.1. Working with Threads in Android
By default, when an Android application starts, it runs on a single thread known as the ‘main thread’ or ‘UI thread.’ But when we need to perform heavy or time-consuming operations, running these tasks on the main thread can result in a non-responsive UI, leading to an “Application Not Responding” (ANR) error.
To prevent this, we use worker threads, which run in the background and handle heavy operations, keeping the main thread free for UI updates.
Here’s a basic example of how to create a new thread in Android:
```java new Thread(new Runnable() { @Override public void run() { // Task to be performed in the background } }).start(); ```
Although threads are a powerful tool, they have their limitations. The most notable is the inability to update the UI from a background thread. Android doesn’t allow UI updates from a non-UI thread, leading us to another critical tool: Handlers.
2. Handlers
A Handler is a powerful component that can schedule code to run at some point in the future, post delayed actions to be executed, and even process long-running computations without affecting the UI thread.
2.1. Working with Handlers in Android
Here’s an example of how you can use a Handler to update the UI from a background thread:
```java final Handler handler = new Handler(Looper.getMainLooper()); new Thread(new Runnable() { @Override public void run() { // Task to be performed in the background handler.post(new Runnable() { @Override public void run() { // UI updates go here } }); } }).start(); ```
In the above code, the `Handler` is associated with the main thread’s `Looper`. The `post()` method queues the Runnable to be run on the main thread, allowing UI updates.
However, while Handlers make it possible to communicate between threads, they require careful management to avoid memory leaks or crashes. This is where the AsyncTask comes in.
3. AsyncTask
AsyncTask is a helper class that simplifies the use of Threads and Handlers. It allows you to perform background operations and publish results on the UI thread without having to manipulate threads or handlers.
3.1. Working with AsyncTask in Android
Here’s an example of how you can use an AsyncTask to download a file in the background:
```java private class DownloadFileTask extends AsyncTask<String, Void, Void> { @Override protected Void doInBackground(String... urls) { // Perform file download here return null; } @Override protected void onPostExecute(Void result) { // Update UI after download is complete } } // Execute the AsyncTask with the URL of the file to be downloaded new DownloadFileTask().execute("http://example.com/file.pdf"); ```
In the `doInBackground()` method, you perform the background operation (like downloading a file). You can then override the `onPostExecute()` method to update the UI after the background operation is complete. It’s a neat and concise way to handle background operations.
Conclusion
Android provides robust tools to handle background processing, an aspect pivotal for creating applications that are responsive and ensure a seamless user experience. This understanding is particularly important when you look to hire Android developers, as mastery over Threads, Handlers, and AsyncTask allows them to effectively manage background tasks without freezing the UI or causing ANR errors.
However, it’s crucial to keep in mind that each of these tools has its unique strengths and limitations. Threads, although powerful, cannot update the UI. Handlers can bridge this gap, but they require careful management. AsyncTask offers a simplified process but lacks the flexibility of directly using Threads and Handlers.
As you hire Android developers, also note that AsyncTask has been deprecated since API level 30. The Android team now advocates for other solutions like Coroutines, LiveData, and WorkManager for background work. However, for simpler tasks or maintaining legacy apps, the tools discussed in this post are still highly relevant and commonly employed.
Table of Contents
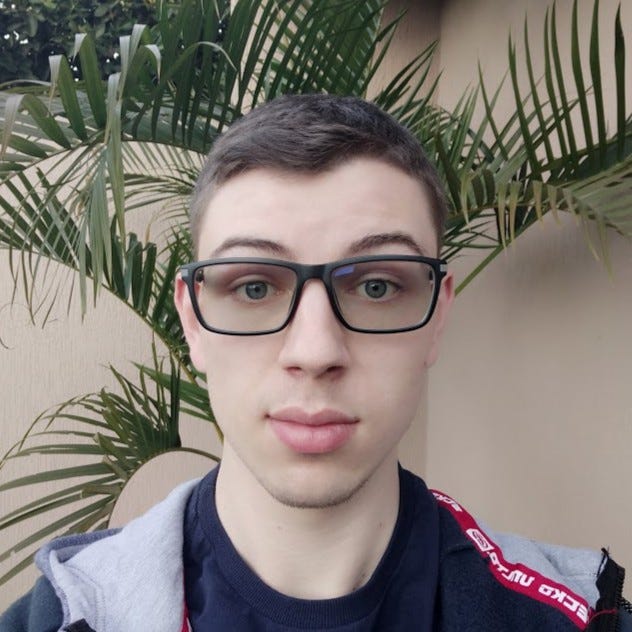
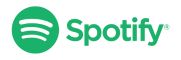