Android Background Services: Running Tasks in the Background
In the world of Android app development, providing a seamless user experience is paramount. However, some tasks, such as downloading large files, processing data, or fetching content from servers, can take a considerable amount of time, leading to a poor user experience if performed on the main thread. To overcome this challenge, Android offers background services, allowing developers to run tasks in the background without hindering the app’s responsiveness. In this blog, we’ll explore the importance of Android background services and learn how to implement them effectively to ensure smooth user interactions.
1. Understanding Background Services:
Background services in Android are components that execute tasks outside the scope of an activity’s lifecycle. These services allow tasks to continue even when the app is not in the foreground, improving user experience and resource management. There are two types of background services:
1.1. Started Services:
Started services are initiated explicitly by an app component, such as an activity or another service. They continue running until they complete their task or are stopped programmatically.
1.2. Bound Services:
Bound services provide a client-server interface, allowing components to bind to the service and interact with it. Once all clients unbind, the service is destroyed.
2. Implementing Background Services:
Let’s dive into the steps to implement background services in Android.
2.1. Creating a Background Service:
To create a background service, you need to extend the Service class and override its lifecycle methods. First, define your service in the AndroidManifest.xml file:
xml <service android:name=".MyBackgroundService" />
Now, create the MyBackgroundService class:
java public class MyBackgroundService extends Service { @Override public IBinder onBind(Intent intent) { return null; } @Override public int onStartCommand(Intent intent, int flags, int startId) { // Task to be performed in the background performBackgroundTask(); return START_STICKY; } private void performBackgroundTask() { // Your background task implementation goes here } @Override public void onDestroy() { super.onDestroy(); // Cleanup resources if needed } }
2.2. Starting a Background Service:
To start a background service, you can use the startService() method with an Intent. Here’s an example of starting MyBackgroundService from an activity:
java Intent serviceIntent = new Intent(this, MyBackgroundService.class); startService(serviceIntent);
2.3. Stopping a Background Service:
As started services do not stop automatically once their task is complete, you need to stop them explicitly when they are no longer needed. To stop the service, use the stopService() method:
java Intent serviceIntent = new Intent(this, MyBackgroundService.class); stopService(serviceIntent);
3. Managing Background Service Lifecycle:
Understanding the lifecycle of a background service is crucial for proper management and resource utilization.
3.1. onCreate():
This method is called when the service is created, allowing you to perform one-time setup tasks, such as initializing variables or creating notification channels.
3.2. onStartCommand():
As discussed earlier, this method is called when the service is started using startService(). Here, you can perform background tasks that might take some time to complete.
3.3. onBind():
If your service is a bound service, this method returns an IBinder interface that clients can use to interact with the service.
3.4. onDestroy():
This method is called when the service is about to be destroyed. Use it to release any resources held by the service.
4. Running Tasks in the Foreground:
In some cases, you may need to run a background task in the foreground to ensure it isn’t killed by the system in low-memory situations and to provide a persistent notification to the user.
4.1. Creating a Foreground Service:
To convert a background service into a foreground service, you need to call startForeground() within onStartCommand(). This method requires a notification that will be shown to the user while the service is running.
java private static final int NOTIFICATION_ID = 1; private static final String CHANNEL_ID = "my_channel"; private void createNotificationChannel() { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) { NotificationChannel channel = new NotificationChannel(CHANNEL_ID, "My Channel", NotificationManager.IMPORTANCE_DEFAULT); NotificationManager manager = getSystemService(NotificationManager.class); manager.createNotificationChannel(channel); } } private void startForegroundService() { createNotificationChannel(); Notification notification = new NotificationCompat.Builder(this, CHANNEL_ID) .setContentTitle("My Foreground Service") .setContentText("Task running in the background") .setSmallIcon(R.drawable.ic_notification) .build(); startForeground(NOTIFICATION_ID, notification); }
5. Handling Background Service Concurrency:
When dealing with long-running tasks, you must consider concurrency and thread safety to avoid conflicts and data corruption.
5.1. Using AsyncTask:
For simple background tasks that don’t require complex concurrency, AsyncTask can be a suitable choice. It provides easy ways to perform background work and update UI elements on the main thread.
java public class MyBackgroundTask extends AsyncTask<Void, Void, Void> { @Override protected Void doInBackground(Void... voids) { // Background task execution return null; } @Override protected void onPostExecute(Void aVoid) { // UI updates after background task completion } }
5.2. Using Thread and Handler:
For more complex tasks, consider using the Thread class in combination with Handler. This allows you to run tasks on a separate thread and communicate back with the main thread.
java public class MyBackgroundTask implements Runnable { private Handler handler; public MyBackgroundTask(Handler handler) { this.handler = handler; } @Override public void run() { // Background task execution // Send result to the main thread handler.post(() -> { // UI updates after background task completion }); } }
Conclusion
Implementing background services is crucial in Android app development to perform resource-intensive tasks without affecting the user experience. By understanding the different types of background services, managing their lifecycles, and ensuring proper concurrency handling, developers can create responsive and efficient applications. Whether it’s downloading files, fetching data from servers, or processing large amounts of information, background services offer a powerful tool to enhance the performance of Android apps and keep users satisfied with their seamless interactions.
Table of Contents
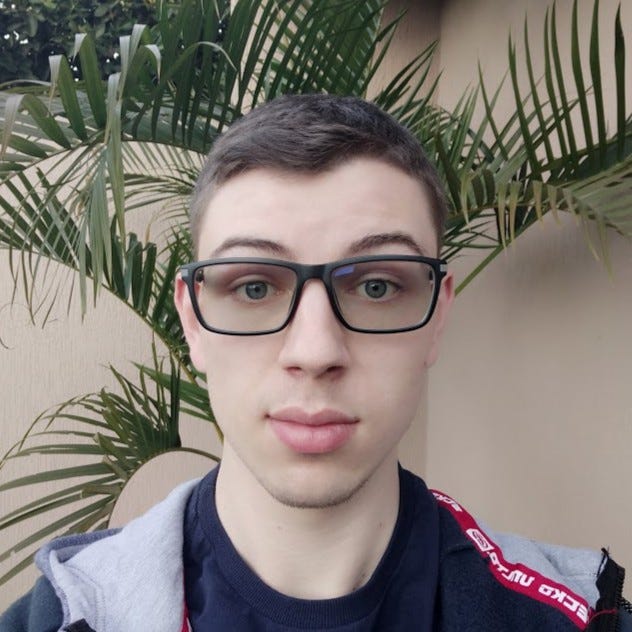
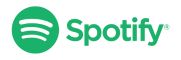