The Definitive Guide to Android Background Tasks and Job Scheduling
Android applications often need to perform tasks in the background, away from the user’s immediate view. These tasks may range from periodically syncing data to the cloud, downloading new content, or performing intensive computations. Given the battery constraints and diverse capabilities of Android devices, managing background tasks efficiently is crucial. If this sounds complex, you might want to consider hiring Android developers to streamline and optimize this process for you.
Table of Contents
In this blog post, we’ll delve into the various methods available for scheduling and executing jobs on Android. By the end, you’ll have a good understanding of when and how to use these methods.
1. Android’s Legacy: `AsyncTask`
Before diving into newer methodologies, it’s worth mentioning `AsyncTask`. It was a popular way to handle background operations. However, it has been deprecated in Android 11, primarily due to its potential to cause memory leaks and complexities around configuration changes.
Example:
```java private class DownloadTask extends AsyncTask<String, Integer, String> { @Override protected String doInBackground(String... urls) { // Perform background operation return "Downloaded!"; } @Override protected void onPostExecute(String result) { // Update UI after the task is completed } } ```
Despite its legacy, `AsyncTask` is no longer recommended. Let’s look at better alternatives.
2. WorkManager
Introduced in Android Jetpack, WorkManager is a powerful tool for scheduling background tasks that are deferrable and require guaranteed execution. This means even if the app exits or the device restarts, the task will be executed.
Example
To run a simple task:
```kotlin val workRequest = OneOffWorkRequestBuilder<MyWorker>().build() WorkManager.getInstance().enqueue(workRequest) ```
`MyWorker` should extend the `Worker` class:
```kotlin class MyWorker(ctx: Context, params: WorkerParameters) : Worker(ctx, params) { override fun doWork(): Result { // Execute your task return Result.success() } } ```
3. JobScheduler
For devices running API 21 (Lollipop) and above, `JobScheduler` is another efficient way to schedule tasks. It’s particularly useful when you have requirements like network connectivity or charging status.
Example:
```java ComponentName componentName = new ComponentName(this, MyJobService.class); JobInfo jobInfo = new JobInfo.Builder(123, componentName) .setRequiresCharging(true) .setRequiredNetworkType(JobInfo.NETWORK_TYPE_UNMETERED) .build(); JobScheduler jobScheduler = (JobScheduler) getSystemService(JOB_SCHEDULER_SERVICE); jobScheduler.schedule(jobInfo); ```
Where `MyJobService` is:
```java public class MyJobService extends JobService { @Override public boolean onStartJob(JobParameters params) { // Execute your task return true; // return `true` if the job is running in a separate thread } @Override public boolean onStopJob(JobParameters params) { // Handle job being interrupted return true; // return `true` to reschedule } } ```
4. AlarmManager
For periodic tasks or events, `AlarmManager` is quite handy. However, it’s not guaranteed to run precisely at the scheduled time, especially with the introduction of Doze mode in Android 6.0.
Example:
```java AlarmManager alarmManager = (AlarmManager) getSystemService(ALARM_SERVICE); Intent intent = new Intent(this, MyAlarmReceiver.class); PendingIntent pendingIntent = PendingIntent.getBroadcast(this, 0, intent, 0); long triggerAtMillis = System.currentTimeMillis() + (60 * 1000); // in 1 minute alarmManager.set(AlarmManager.RTC_WAKEUP, triggerAtMillis, pendingIntent); ```
5. Foreground Service
For tasks that need to be immediately visible to the user, a foreground service with a notification is the way to go. Itβs not terminated by the system even if the user is not interacting with the app.
Example:
```kotlin val notification: Notification = ... startForeground(NOTIFICATION_ID, notification); // Execute your task in the service ```
Conclusion:
Background tasks in Android have evolved significantly over the years. While we discussed a few methods above, it’s essential to pick the right one based on the nature of the task, required precision, and user experience. For most use cases, `WorkManager` tends to be the most versatile and recommended option. If you’re looking to optimize these processes further or feel overwhelmed, you might want to hire Android developers. They can provide expertise and understanding of these tools, enabling you to make more informed decisions when building robust Android applications.
Table of Contents
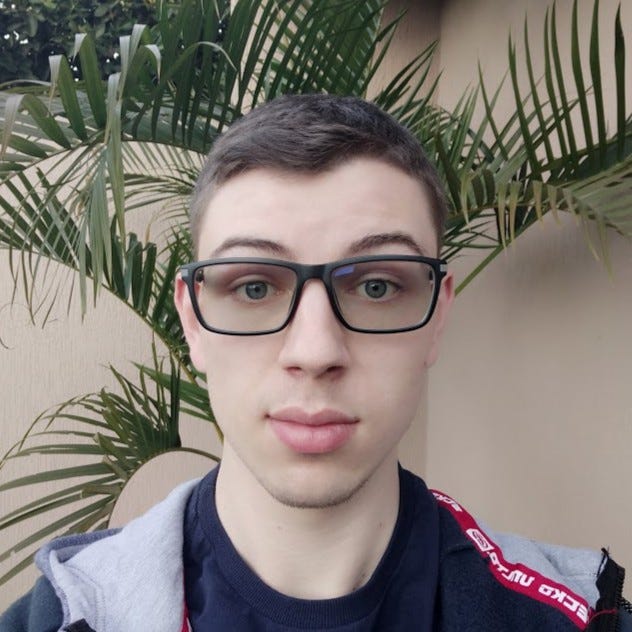
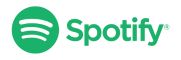