Exploring Android Bluetooth: Building Wireless Connections
In our increasingly connected world, Bluetooth technology has become an integral part of our daily lives. From wireless headphones and smartwatches to home automation and IoT devices, Bluetooth enables seamless communication between devices. Android, being the most popular mobile operating system, provides robust support for Bluetooth functionality, allowing developers to create innovative applications that leverage wireless connections.
In this blog, we will embark on an exciting journey to explore Android Bluetooth and learn how to build wireless connections between devices. Whether you’re a beginner or an experienced Android developer, this guide will equip you with the necessary knowledge to implement Bluetooth functionalities effectively.
1. Understanding Bluetooth Technology
1.1 Bluetooth Basics
Bluetooth is a short-range wireless communication technology that enables data exchange between devices over short distances. It operates on the 2.4 GHz ISM band and uses radio waves for transmission. Bluetooth technology is designed to be energy-efficient, making it ideal for applications that require low power consumption.
1.2 Bluetooth Classic vs. Bluetooth Low Energy (BLE)
Android supports both Bluetooth Classic and Bluetooth Low Energy (BLE). Bluetooth Classic is suitable for applications that require continuous data transmission, such as audio streaming. On the other hand, BLE is optimized for intermittent data exchange and is ideal for applications like fitness trackers and IoT devices.
1.3 Bluetooth Profiles and Services
Bluetooth devices use profiles to define the communication protocols they support. Common profiles include Headset Profile (HSP), Hands-Free Profile (HFP), Advanced Audio Distribution Profile (A2DP), and more. Services, on the other hand, represent specific functionalities offered by a Bluetooth device. Understanding profiles and services is crucial for successful Bluetooth communication.
2. Setting Up Your Android Project
Before diving into Bluetooth development, ensure you have the necessary tools and environment set up. First, download and install Android Studio, the official Integrated Development Environment (IDE) for Android app development. Next, create a new Android project or open an existing one.
3. Checking Bluetooth Support
To begin working with Bluetooth in your Android app, you must first check if the device supports Bluetooth and if it is enabled. Use the following code snippet to perform this check:
java // Check if the device supports Bluetooth BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter(); if (bluetoothAdapter == null) { // Device does not support Bluetooth // Handle this case as per your application requirements return; } // Check if Bluetooth is enabled if (!bluetoothAdapter.isEnabled()) { // If Bluetooth is not enabled, prompt the user to enable it Intent enableBtIntent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE); startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT); }
In this code snippet, we first get the default Bluetooth adapter using BluetoothAdapter.getDefaultAdapter(). If the adapter is null, it means that the device does not support Bluetooth, and we can handle this scenario accordingly.
Next, we check if Bluetooth is enabled using bluetoothAdapter.isEnabled(). If it’s not enabled, we prompt the user to enable it using the ACTION_REQUEST_ENABLE intent, which will show a dialog to the user requesting Bluetooth activation. Don’t forget to handle the result of this intent in the onActivityResult method.
4. Requesting Bluetooth Permissions
To access Bluetooth functionality, your app needs the appropriate permissions declared in the AndroidManifest.xml file. The following permissions are required for Bluetooth communication:
xml <uses-permission android:name="android.permission.BLUETOOTH"/> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
The BLUETOOTH and BLUETOOTH_ADMIN permissions are necessary for basic Bluetooth operations, while ACCESS_FINE_LOCATION is required for discovering nearby Bluetooth devices. Starting from Android 12 (API level 31), you also need to request the ACCESS_FINE_LOCATION permission at runtime explicitly. Use the following code to request runtime permission:
java // Check for ACCESS_FINE_LOCATION permission if (ContextCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) { // Request permission if it has not been granted ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, REQUEST_ACCESS_FINE_LOCATION); }
In this code, we check if the ACCESS_FINE_LOCATION permission has been granted using ContextCompat.checkSelfPermission(). If it hasn’t been granted, we request the permission using ActivityCompat.requestPermissions(). Remember to handle the permission request result in the onRequestPermissionsResult method.
5. Discovering Nearby Bluetooth Devices
Discovering nearby Bluetooth devices is one of the primary functionalities in many Bluetooth-enabled applications. You can list paired devices and discover new ones to establish connections.
5.1 Listing Paired Devices
To retrieve the list of paired Bluetooth devices, use the following code:
java // Get the paired devices set Set<BluetoothDevice> pairedDevices = bluetoothAdapter.getBondedDevices(); // Check if there are paired devices if (pairedDevices.size() > 0) { // Loop through paired devices and do something with each device for (BluetoothDevice device : pairedDevices) { String deviceName = device.getName(); String deviceAddress = device.getAddress(); // Do something with the device information } }
In this code snippet, we use bluetoothAdapter.getBondedDevices() to obtain a set of paired Bluetooth devices. If there are paired devices, we iterate through the set and extract information such as the device name and address.
5.2 Discovering New Devices
To discover nearby Bluetooth devices, you need to use a BroadcastReceiver to receive the discovery results. The following code demonstrates how to start the discovery process and handle the results:
java // Register for discovery broadcasts BroadcastReceiver discoveryReceiver = new BroadcastReceiver() { @Override public void onReceive(Context context, Intent intent) { String action = intent.getAction(); if (BluetoothAdapter.ACTION_DISCOVERY_STARTED.equals(action)) { // Discovery process started // Perform any necessary initialization } else if (BluetoothDevice.ACTION_FOUND.equals(action)) { // Discovery found a new device BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE); String deviceName = device.getName(); String deviceAddress = device.getAddress(); // Do something with the discovered device } else if (BluetoothAdapter.ACTION_DISCOVERY_FINISHED.equals(action)) { // Discovery process finished // Perform any necessary cleanup } } }; // Register the receiver IntentFilter filter = new IntentFilter(); filter.addAction(BluetoothAdapter.ACTION_DISCOVERY_STARTED); filter.addAction(BluetoothDevice.ACTION_FOUND); filter.addAction(BluetoothAdapter.ACTION_DISCOVERY_FINISHED); registerReceiver(discoveryReceiver, filter); // Start the discovery process bluetoothAdapter.startDiscovery();
In this code, we create a BroadcastReceiver to handle the discovery process events. When the discovery starts (BluetoothAdapter.ACTION_DISCOVERY_STARTED), you can perform any necessary initialization. When a new device is found (BluetoothDevice.ACTION_FOUND), you can retrieve information about the device and take appropriate actions. Finally, when the discovery process finishes (BluetoothAdapter.ACTION_DISCOVERY_FINISHED), you can perform any necessary cleanup.
Remember to unregister the receiver when it’s no longer needed to prevent memory leaks:
java unregisterReceiver(discoveryReceiver);
In the next part of this blog, we’ll cover how to establish a Bluetooth connection between devices and transfer data seamlessly. Stay tuned for more exciting code samples and in-depth explanations.
6. Establishing a Bluetooth Connection
Once you have discovered the Bluetooth devices nearby, you may want to establish a connection with a specific device. The connection process involves creating a Bluetooth socket and managing the connection as either a server or a client.
6.1 Creating a Server Socket
As a server, your Android device will listen for incoming Bluetooth connections from other devices. To create a server socket, use the following code:
java BluetoothServerSocket serverSocket = null; try { // Create a server socket serverSocket = bluetoothAdapter.listenUsingRfcommWithServiceRecord("YourApp", MY_UUID); } catch (IOException e) { // Handle any errors that may occur during socket creation } // Start listening for incoming connections if (serverSocket != null) { BluetoothSocket socket; while (true) { try { // Accept incoming connection socket = serverSocket.accept(); } catch (IOException e) { // Handle any errors that may occur during connection acceptance break; } // If a connection was accepted, handle the connection in a separate thread if (socket != null) { // Handle the connection in a separate thread // For example, create a ConnectedThread to manage the connection // (code for ConnectedThread is shown later) } } }
In this code, we create a server socket using bluetoothAdapter.listenUsingRfcommWithServiceRecord(). The listenUsingRfcommWithServiceRecord method takes two arguments: the service name (a string identifier for your app) and a universally unique identifier (UUID) that identifies the service. Both the server and client must use the same UUID to establish a connection.
Once the server socket is created, we enter a loop to listen for incoming connections using serverSocket.accept(). When a connection is accepted, we obtain a BluetoothSocket that represents the connection with the remote device.
6.2 Creating a Client Socket
As a client, your Android device will initiate a connection to a remote Bluetooth server. To create a client socket, use the following code:
java BluetoothDevice remoteDevice = ...; // Obtain the BluetoothDevice object representing the remote server BluetoothSocket clientSocket = null; try { // Create a client socket clientSocket = remoteDevice.createRfcommSocketToServiceRecord(MY_UUID); } catch (IOException e) { // Handle any errors that may occur during socket creation } // Connect to the remote device try { clientSocket.connect(); } catch (IOException e) { // Handle any errors that may occur during the connection process } // If the connection was successful, handle the connection in a separate thread if (clientSocket.isConnected()) { // Handle the connection in a separate thread // For example, create a ConnectedThread to manage the connection // (code for ConnectedThread is shown later) }
In this code, we create a client socket using remoteDevice.createRfcommSocketToServiceRecord(). The createRfcommSocketToServiceRecord method takes the same UUID that the server used to create its server socket.
After creating the client socket, we call clientSocket.connect() to initiate the connection to the remote device. If the connection is successful (clientSocket.isConnected() returns true), we can handle the connection in a separate thread, just like in the server example.
7. Data Transfer over Bluetooth
Once the connection is established, you can transfer data between the devices using the Bluetooth socket. To send and receive data, we’ll create a separate thread that manages the data transfer.
7.1 Sending Data
To send data over Bluetooth, use the following code:
java BluetoothSocket socket = ...; // Obtain the BluetoothSocket object representing the connection // Get the output stream from the Bluetooth socket OutputStream outputStream; try { outputStream = socket.getOutputStream(); } catch (IOException e) { // Handle any errors that may occur when getting the output stream return; } // Send data over the output stream String message = "Hello, Bluetooth!"; byte[] messageBytes = message.getBytes(Charset.defaultCharset()); try { outputStream.write(messageBytes); } catch (IOException e) { // Handle any errors that may occur during data transmission }
In this code, we obtain the output stream from the Bluetooth socket using socket.getOutputStream(). Then, we convert the message string into bytes using the device’s default character set and write the data to the output stream using outputStream.write().
7.2 Receiving Data
To receive data over Bluetooth, use the following code:
java BluetoothSocket socket = ...; // Obtain the BluetoothSocket object representing the connection // Get the input stream from the Bluetooth socket InputStream inputStream; try { inputStream = socket.getInputStream(); } catch (IOException e) { // Handle any errors that may occur when getting the input stream return; } // Create a buffer to store the incoming data byte[] buffer = new byte[1024]; int numBytes; // Number of bytes read from the input stream try { // Read data from the input stream numBytes = inputStream.read(buffer); } catch (IOException e) { // Handle any errors that may occur during data reception } // Convert the received data to a string String receivedMessage = new String(buffer, 0, numBytes, Charset.defaultCharset());
In this code, we obtain the input stream from the Bluetooth socket using socket.getInputStream(). Then, we create a buffer to store the incoming data and read data from the input stream using inputStream.read(buffer). The method returns the number of bytes read, which we use to create a string from the received data.
8. Handling Bluetooth State Changes
Bluetooth state changes, such as turning on/off Bluetooth or entering/leaving airplane mode, can affect your app’s Bluetooth functionality. You can listen for these state changes using a BroadcastReceiver and handle them accordingly.
java BroadcastReceiver stateChangeReceiver = new BroadcastReceiver() { @Override public void onReceive(Context context, Intent intent) { String action = intent.getAction(); if (BluetoothAdapter.ACTION_STATE_CHANGED.equals(action)) { int newState = intent.getIntExtra(BluetoothAdapter.EXTRA_STATE, BluetoothAdapter.STATE_OFF); switch (newState) { case BluetoothAdapter.STATE_OFF: // Bluetooth has been turned off // Handle this state change break; case BluetoothAdapter.STATE_TURNING_OFF: // Bluetooth is turning off // Handle this state change break; case BluetoothAdapter.STATE_ON: // Bluetooth has been turned on // Handle this state change break; case BluetoothAdapter.STATE_TURNING_ON: // Bluetooth is turning on // Handle this state change break; } } } }; // Register the receiver IntentFilter filter = new IntentFilter(BluetoothAdapter.ACTION_STATE_CHANGED); registerReceiver(stateChangeReceiver, filter);
In this code, we create a BroadcastReceiver to listen for Bluetooth state changes using BluetoothAdapter.ACTION_STATE_CHANGED. When a state change occurs, we retrieve the new state from the Intent using intent.getIntExtra(BluetoothAdapter.EXTRA_STATE, BluetoothAdapter.STATE_OFF) and handle it accordingly.
Remember to unregister the receiver when it’s no longer needed to prevent memory leaks:
java unregisterReceiver(stateChangeReceiver);
9. Bluetooth Best Practices
To ensure a smooth and efficient Bluetooth experience for your users, consider the following best practices:
9.1 Managing Battery Consumption
Bluetooth can consume a significant amount of battery power, especially during continuous data transmission. Optimize your app’s power usage by minimizing data transfers and using energy-efficient Bluetooth profiles, such as Bluetooth Low Energy (BLE), whenever possible.
9.2 Error Handling and Exception Management
Bluetooth operations may fail due to various reasons, such as device disconnections or communication errors. Implement robust error handling and exception management to handle these situations gracefully and provide meaningful feedback to users.
10. Troubleshooting Common Bluetooth Issues
Bluetooth development can be complex, and you may encounter various issues during the process. When troubleshooting, consider the following steps:
- Verify that Bluetooth is enabled on both the client and server devices.
- Ensure that both devices are discoverable and within range of each other.
- Check for proper permissions in your app to access Bluetooth functionality.
- Test your app with different Bluetooth devices to ensure compatibility.
- Monitor logcat for any error messages related to Bluetooth operations.
11. Bluetooth Security
Bluetooth communication can potentially be vulnerable to security threats, such as unauthorized access or data interception. To enhance Bluetooth security, consider implementing the following measures:
11.1 Pairing and Bonding
Use Bluetooth pairing and bonding to establish secure connections between devices. Pairing involves creating a link key shared between devices to encrypt data during communication. Bonding stores this link key for future use, reducing the need for repeated pairing.
11.2 Secure Data Transmission
Encrypt sensitive data before sending it over a Bluetooth connection. Use encryption algorithms supported by both devices to ensure secure data transmission.
12. Advanced Bluetooth Features
Android provides additional features to enhance Bluetooth functionality in your apps:
12.1 Bluetooth Low Energy (BLE) Communication
BLE is designed for low-power devices that require intermittent data transfer. Implement BLE communication for applications like fitness trackers, smartwatches, and other IoT devices.
12.2 Bluetooth Background Processing
To ensure uninterrupted Bluetooth communication, consider managing Bluetooth operations in the background using services or foreground services.
13. Real-world Applications of Bluetooth Technology
Bluetooth technology finds applications in various industries and use cases:
- Smart Home Automation: Control smart home devices, such as lights and thermostats, wirelessly using Bluetooth.
- Health and Fitness: Track fitness activities, heart rate, and sleep patterns using Bluetooth-enabled fitness trackers and smartwatches.
- Retail and Marketing: Deliver targeted advertisements and promotions to customers via Bluetooth beacons.
- Automotive: Enable hands-free calling and media streaming in cars using Bluetooth connectivity.
Conclusion
Congratulations on exploring the exciting world of Android Bluetooth! In this blog, we covered the fundamentals of Bluetooth technology, setting up your Android project, discovering nearby devices, establishing Bluetooth connections, and transferring data seamlessly.
Remember to follow best practices for battery consumption, error handling, and security to create a reliable and user-friendly Bluetooth-enabled app. As you continue to develop applications that leverage Bluetooth, you’ll discover endless possibilities for wireless communication and innovative solutions in the connected world.
Happy Bluetooth coding!
Table of Contents
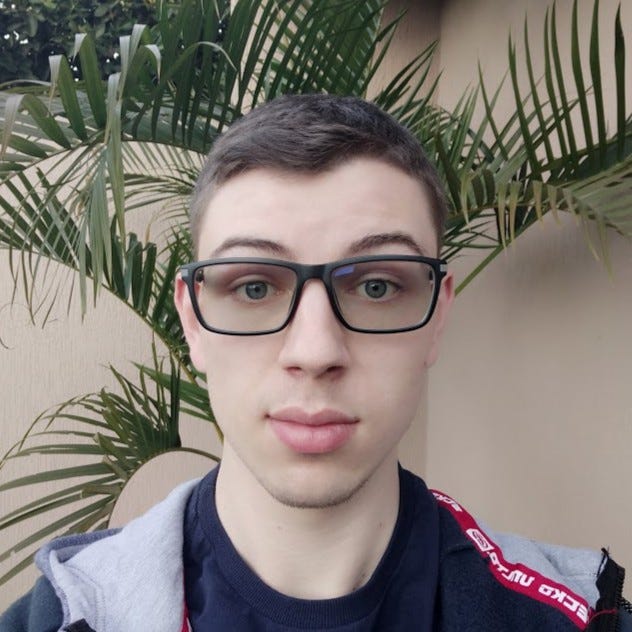
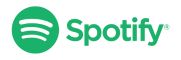