Android’s BLE Integration: The Catalyst for Innovative IoT Projects
Bluetooth Low Energy (BLE), also known as Bluetooth Smart, is a universal low-power wireless standard designed for short-range communication between devices. Due to its power efficiency, it has become the preferred choice for Internet of Things (IoT) devices. For businesses looking to capitalize on this trend, it might be a good time to hire Android developers. The Android framework offers them extensive BLE support to craft IoT applications that interact seamlessly with BLE devices.
Table of Contents
In this article, we’ll delve into building an Android application using BLE and showcase some examples of IoT applications.
1. Understanding BLE Basics
BLE operates in the 2.4 GHz ISM band and is optimized for applications that need low latency and power consumption. Some of the prominent features of BLE include:
– Stateless Communication: BLE does not maintain a consistent connection but uses a concept of advertising and discovering.
– GATT (Generic Attribute Profile): Defines the way two BLE devices send and receive standard messages.
– Services and Characteristics: BLE devices provide services, and each service can have one or many characteristics.
2. Setting Up Android for BLE Development
First, ensure you have permissions in the AndroidManifest.xml file:
```xml <uses-permission android:name="android.permission.BLUETOOTH"/> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/> ```
Next, check if the Android device supports BLE:
```java BluetoothManager bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE); BluetoothAdapter bluetoothAdapter = bluetoothManager.getAdapter(); if (bluetoothAdapter == null || !bluetoothAdapter.isEnabled() || !getPackageManager().hasSystemFeature(PackageManager.FEATURE_BLUETOOTH_LE)) { Toast.makeText(this, "BLE not supported", Toast.LENGTH_SHORT).show(); finish(); } ```
3. Scanning for BLE Devices
Use `BluetoothAdapter` to start and stop scanning:
```java BluetoothLeScanner scanner = bluetoothAdapter.getBluetoothLeScanner(); scanner.startScan(scanCallback); // Callback private ScanCallback scanCallback = new ScanCallback() { @Override public void onScanResult(int callbackType, ScanResult result) { // Process the scan result. } }; ```
Remember to stop scanning when necessary to save power:
```java scanner.stopScan(scanCallback); ```
4. Connecting to a BLE Device
Once you find a device, you can connect to it:
```java BluetoothDevice device = scanResult.getDevice(); BluetoothGatt bluetoothGatt = device.connectGatt(this, false, gattCallback); ```
5. Reading and Writing Characteristics
After connecting, you can discover services and interact with characteristics:
```java bluetoothGatt.discoverServices(); // Callback BluetoothGattCallback gattCallback = new BluetoothGattCallback() { @Override public void onServicesDiscovered(BluetoothGatt gatt, int status) { if (status == BluetoothGatt.GATT_SUCCESS) { for (BluetoothGattService service : gatt.getServices()) { for (BluetoothGattCharacteristic characteristic : service.getCharacteristics()) { // Read characteristic gatt.readCharacteristic(characteristic); // Or write to it characteristic.setValue("Some Value"); gatt.writeCharacteristic(characteristic); } } } } }; ```
Examples of IoT Applications Using BLE:
- Smart Home: Imagine controlling your lights, thermostats, or even your coffee maker using BLE. Android apps can communicate with these devices, fetching their states or sending commands.
- Healthcare: Wearable devices, like heart rate monitors or insulin pumps, can send patient data in real-time to an Android application, providing a seamless health monitoring experience.
- Fitness Trackers: Track your steps, heart rate, or sleep patterns by syncing your fitness tracker with a dedicated Android application. This provides rich data analytics and visualizations for users.
- Industrial IoT: BLE sensors can be deployed in factories to monitor machinery or environmental conditions. An Android application can collect this data, offering insights and potentially predicting machinery failures.
- Asset Tracking: Track assets or inventory in real-time. BLE tags attached to items send signals to Android devices, allowing businesses to track and manage their inventory seamlessly.
Conclusion
BLE is a powerful technology, particularly suited for IoT applications due to its low energy consumption. The Android platform offers a robust set of tools and APIs for developers to integrate BLE and create innovative IoT solutions. For businesses aiming to harness this potential, it might be an opportune time to hire Android developers. Whether you’re looking to dive into smart home applications, health monitoring, or industrial IoT, BLE on Android is a strong starting point.
Table of Contents
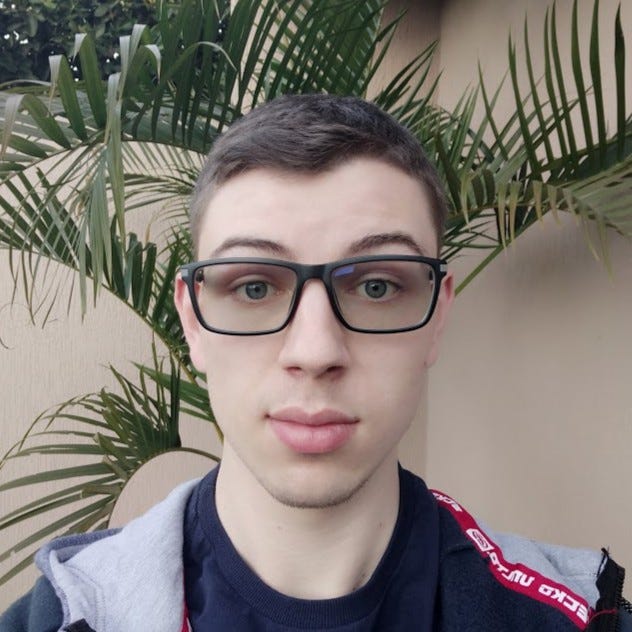
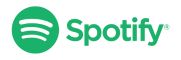