Exploring Android Graphics: Canvas and OpenGL ES
In the vast and dynamic world of Android app development, graphics play a crucial role in creating engaging and visually appealing user experiences. From simple 2D graphics to immersive 3D scenes, Android offers two powerful frameworks to work with: Canvas and OpenGL ES. These frameworks enable developers to create stunning visualizations, animations, and interactive elements that captivate users. In this blog, we’ll explore both Canvas and OpenGL ES, their differences, capabilities, and how to use them to elevate your Android app’s graphics to the next level.
Table of Contents
1. Understanding Canvas
1.1. What is Canvas?
Canvas is a 2D drawing framework in Android that allows you to draw shapes, text, and images directly onto a surface. It acts as a drawing board where you can define what should appear on the screen. Every Android view comes with an associated Canvas, which can be accessed through the onDraw() method. By obtaining the Canvas object, you can draw your custom graphics onto the view.
1.2. Basic Drawing on Canvas
Let’s start with a simple example of drawing a circle on a custom view using Canvas:
java public class CircleView extends View { private Paint paint; public CircleView(Context context) { super(context); paint = new Paint(); paint.setColor(Color.BLUE); } @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); int centerX = getWidth() / 2; int centerY = getHeight() / 2; int radius = 100; canvas.drawCircle(centerX, centerY, radius, paint); } }
1.3. Working with Paths
Besides basic shapes, Canvas allows you to draw complex paths by defining a series of points and curves. You can create custom shapes and intricate designs using the Path class.
java public class CustomShapeView extends View { private Paint paint; private Path path; public CustomShapeView(Context context) { super(context); paint = new Paint(); paint.setColor(Color.RED); path = new Path(); // Define your custom path here path.moveTo(50, 50); path.lineTo(150, 150); path.lineTo(250, 100); path.close(); } @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); canvas.drawPath(path, paint); } }
1.4. Transformations and Animations
Canvas also supports transformations like translation, rotation, and scaling, allowing you to animate your drawings dynamically.
java public class AnimatedShapeView extends View { private Paint paint; private float rotationAngle = 0f; public AnimatedShapeView(Context context) { super(context); paint = new Paint(); paint.setColor(Color.GREEN); } @Override protected void onDraw(Canvas canvas) { super.onDraw(canvas); int centerX = getWidth() / 2; int centerY = getHeight() / 2; int size = 200; canvas.save(); canvas.rotate(rotationAngle, centerX, centerY); canvas.drawRect(centerX - size / 2, centerY - size / 2, centerX + size / 2, centerY + size / 2, paint); canvas.restore(); rotationAngle += 2f; invalidate(); } }
2. Introducing OpenGL ES
2.1. What is OpenGL ES?
OpenGL ES (OpenGL for Embedded Systems) is a cross-platform, royalty-free API that allows you to render 2D and 3D graphics in Android apps. It provides low-level access to the GPU (Graphics Processing Unit) and is highly optimized for rendering complex scenes efficiently.
2.2. Setting Up OpenGL ES
Before using OpenGL ES, you need to set up the renderer and the rendering surface. First, create a GLSurfaceView in your activity layout:
xml <android.opengl.GLSurfaceView android:id="@+id/glSurfaceView" android:layout_width="match_parent" android:layout_height="match_parent" />
Then, create a renderer class that implements GLSurfaceView.Renderer:
java public class MyGLRenderer implements GLSurfaceView.Renderer { // Implement required methods: onSurfaceCreated, onDrawFrame, onSurfaceChanged // ... }
Finally, set the renderer in your activity:
java public class MyActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); GLSurfaceView glSurfaceView = findViewById(R.id.glSurfaceView); glSurfaceView.setRenderer(new MyGLRenderer()); } }
2.3. Drawing with OpenGL ES
OpenGL ES provides several drawing primitives like points, lines, and triangles. Here’s an example of drawing a colored triangle using OpenGL ES:
java public class MyGLRenderer implements GLSurfaceView.Renderer { // ... @Override public void onSurfaceCreated(GL10 gl, EGLConfig config) { // Set the clear color (background) and enable smooth shading gl.glClearColor(0.0f, 0.0f, 0.0f, 1.0f); gl.glShadeModel(GL10.GL_SMOOTH); } @Override public void onDrawFrame(GL10 gl) { // Clear the screen and depth buffer gl.glClear(GL10.GL_COLOR_BUFFER_BIT | GL10.GL_DEPTH_BUFFER_BIT); // Set the color of the triangle (R, G, B, Alpha) gl.glColor4f(0.0f, 1.0f, 0.0f, 1.0f); // Define the vertices of the triangle float[] triangleVertices = { 0.0f, 1.0f, 0.0f, -1.0f, -1.0f, 0.0f, 1.0f, -1.0f, 0.0f }; // Enable vertex array for drawing gl.glEnableClientState(GL10.GL_VERTEX_ARRAY); // Define the vertex array gl.glVertexPointer(3, GL10.GL_FLOAT, 0, vertexBuffer); // Draw the triangle gl.glDrawArrays(GL10.GL_TRIANGLES, 0, 3); // Disable vertex array after drawing gl.glDisableClientState(GL10.GL_VERTEX_ARRAY); } // ... }
3. Canvas vs. OpenGL ES
3.1. Choosing the Right Approach
Canvas and OpenGL ES each have their strengths and best use cases. When to use one over the other depends on the complexity and requirements of your graphics.
3.2. Use Canvas When:
- You need to draw simple 2D graphics like shapes, text, or basic animations.
- Your app requires fewer system resources and runs on older devices.
- Your graphics are not performance-critical.
3.3. Use OpenGL ES When:
- You want to create complex 2D or 3D graphics with high-performance rendering.
- Your app involves real-time animations, 3D modeling, or games.
- You require low-level control over the rendering pipeline.
Conclusion
In this blog, we’ve explored the powerful Android graphics frameworks: Canvas and OpenGL ES. We started by understanding Canvas, how to draw basic shapes and paths, and animate them. Then, we introduced OpenGL ES, discussing its setup and how to draw with it using low-level OpenGL commands.
Choosing between Canvas and OpenGL ES depends on your app’s specific needs and the complexity of your graphics. Both frameworks offer exceptional capabilities, whether it’s simple 2D drawing or immersive 3D scenes.
By harnessing the potential of Canvas and OpenGL ES, you can elevate your Android app’s visuals and create captivating user experiences that leave a lasting impression. So go ahead, experiment, and let your creativity shine in the world of Android graphics!
Remember, with great graphics comes great user engagement! Happy coding!
Table of Contents
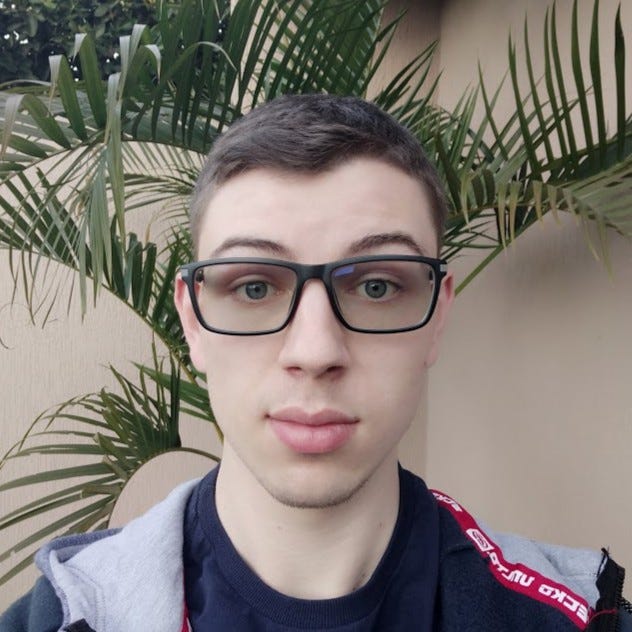
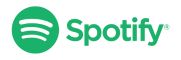