Advanced Topics in Android: Content Providers and Sync Adapters
As an Android developer, you may have already mastered the fundamentals of building user interfaces, handling user interactions, and managing data locally within your app. However, as your app grows in complexity and features, you’ll find the need to handle data in a more sophisticated way. This is where Content Providers and Sync Adapters come into play.
Content Providers and Sync Adapters are advanced concepts in Android that allow you to share and synchronize data between different apps and backend servers seamlessly. They offer powerful mechanisms to interact with data, ensuring data integrity and optimal performance. In this blog, we will delve into the world of Content Providers and Sync Adapters, understanding their concepts, implementation, and how they enhance the capabilities of your Android applications.
1. Understanding Content Providers
1.1. What are Content Providers?
Content Providers act as an intermediary between your app’s data and other apps or system components. They provide a standardized way to share data across apps while ensuring data security and encapsulation. Content Providers are essential when you want to share data with other apps or allow other apps to access and modify your app’s data.
1.2. Implementing a Content Provider
To create a Content Provider, you need to follow these key steps:
1.2.1. Define the Contract
The first step is to define the contract for your Content Provider. The contract includes the data columns, URIs (Uniform Resource Identifiers), and other necessary information about the data you want to expose. Let’s look at an example of a simple contract for a “Books” table:
java public class BooksContract { public static final String AUTHORITY = "com.example.myapp.booksprovider"; public static final Uri BASE_CONTENT_URI = Uri.parse("content://" + AUTHORITY); public static final String PATH_BOOKS = "books"; public static class BooksEntry implements BaseColumns { public static final Uri CONTENT_URI = BASE_CONTENT_URI.buildUpon().appendPath(PATH_BOOKS).build(); public static final String TABLE_NAME = "books"; public static final String COLUMN_TITLE = "title"; public static final String COLUMN_AUTHOR = "author"; } }
1.2.2. Create the Content Provider Class
Next, you’ll create a class that extends ContentProvider and implements the necessary methods. The key methods to override are onCreate(), query(), insert(), update(), and delete(). These methods handle data retrieval, insertion, updating, and deletion operations.
java public class BooksProvider extends ContentProvider { // ... Content Provider implementation ... }
1.2.3. Register the Content Provider
Don’t forget to register your Content Provider in the AndroidManifest.xml file:
xml <provider android:name=".BooksProvider" android:authorities="com.example.myapp.booksprovider" android:exported="false" />
1.3. Querying the Content Provider
After implementing the Content Provider, other apps can query it to retrieve data. For example:
java Cursor cursor = getContentResolver().query( BooksContract.BooksEntry.CONTENT_URI, null, null, null, null ); if (cursor != null && cursor.moveToFirst()) { do { String title = cursor.getString(cursor.getColumnIndex(BooksContract.BooksEntry.COLUMN_TITLE)); String author = cursor.getString(cursor.getColumnIndex(BooksContract.BooksEntry.COLUMN_AUTHOR)); // Do something with the data... } while (cursor.moveToNext()); cursor.close(); }
2. Synchronizing Data with Sync Adapters
2.1. What are Sync Adapters?
Sync Adapters enable your app to synchronize data with backend servers or any other data source. They allow you to perform background data synchronization without draining the device’s battery or affecting the user experience. Sync Adapters work hand-in-hand with the Android Sync framework, providing a smooth and efficient synchronization process.
2.2. Implementing a Sync Adapter
To create a Sync Adapter, follow these steps:
2.2.1. Define the Sync Adapter
First, you need to create a class that extends AbstractThreadedSyncAdapter and implement the required methods:
java public class MySyncAdapter extends AbstractThreadedSyncAdapter { // ... Sync Adapter implementation ... }
2.2.2. Set up the Sync Adapter in the AndroidManifest.xml
Next, declare your Sync Adapter in the AndroidManifest.xml file:
xml <service android:name=".MySyncAdapter" android:exported="true" android:process=":sync"> <intent-filter> <action android:name="android.content.SyncAdapter" /> </intent-filter> <meta-data android:name="android.content.SyncAdapter" android:resource="@xml/sync_adapter" /> </service>
2.2.3. Create the sync_adapter.xml
In the res/xml folder, create the sync_adapter.xml file, where you define the Sync Adapter properties:
xml <sync-adapter xmlns:android="http://schemas.android.com/apk/res/android" android:contentAuthority="com.example.myapp.booksprovider" android:accountType="com.example.myapp" android:userVisible="false" android:supportsUploading="false" android:allowParallelSyncs="false" android:isAlwaysSyncable="true" />
3. Triggering Data Synchronization
Now that you have implemented the Sync Adapter, you can trigger data synchronization manually or schedule periodic syncs.
java // Trigger a manual sync Bundle extras = new Bundle(); extras.putBoolean(ContentResolver.SYNC_EXTRAS_MANUAL, true); ContentResolver.requestSync( account, // Account object BooksContract.AUTHORITY, extras ); // Schedule periodic sync (e.g., every hour) ContentResolver.addPeriodicSync( account, // Account object BooksContract.AUTHORITY, Bundle.EMPTY, TimeUnit.HOURS.toSeconds(1) );
Conclusion
Content Providers and Sync Adapters are essential components for advanced Android development. Content Providers enable secure data sharing among apps, while Sync Adapters facilitate background data synchronization, ensuring your app always has up-to-date information. By mastering these concepts, you can deliver a seamless user experience and unlock a world of possibilities for your Android applications.
In this blog, we’ve covered the fundamental concepts of Content Providers and Sync Adapters, including their implementation and usage. As you dive deeper into Android development, these advanced topics will prove indispensable in building robust, scalable, and efficient apps.
Remember, as an Android developer, continuous learning and practice are vital to stay at the forefront of technology and provide innovative solutions to users’ needs. So, keep exploring, experimenting, and pushing the boundaries of what’s possible with Android! Happy coding!
Table of Contents
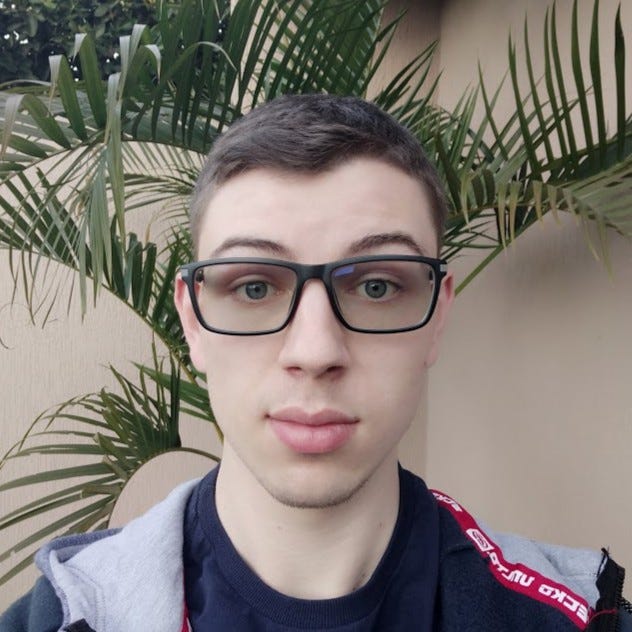
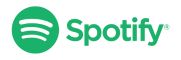