How to Tackle Android Debugging: Proven Tools and Techniques
Developing Android applications can be an exciting endeavor, but the process is often interspersed with multiple debugging sessions. The occasional bugs that pop up during development can be as simple as minor UI glitches or as complex as memory leaks or performance issues. Debugging is an essential part of the Android app development process, which requires the proper tools and techniques to ensure an efficient troubleshooting experience. This understanding and expertise are why many choose to hire Android developers for their projects. In this blog post, we’ll explore several tools and techniques that these professionals use for effective Android app debugging.
Table of Contents
1. Android Studio
As the official Integrated Development Environment (IDE) for Android development, Android Studio offers a host of built-in tools designed specifically for Android app debugging.
- Android Logcat
Android Logcat is a command-line tool that dumps a log of system messages. You can use these messages to track the behavior of your application or the system, including stack traces when the application crashes.
Example: In Android Studio, navigate to the Logcat tab at the bottom of the IDE. Select your device and application from the drop-down menu, and then set the log level you are interested in. You can also filter messages by a specific tag using the search box.
- Android Debugger (ADB)
The Android Debug Bridge is a versatile command-line tool that allows communication between a computer and an Android device (or emulator). It facilitates a variety of device actions, such as installing and debugging apps.
Example: To install an application via ADB, use the following command in your terminal:
```bash adb install my-app.apk ```
To start debugging an application, use the following command:
```bash adb shell am debug -W com.example.myapp/.MainActivity ```
- Android Profiler
This tool helps track the CPU, memory, and network usage of your app in real-time. It helps identify performance bottlenecks.
Example: To use the Android Profiler, click on the “Profiler” tab in Android Studio. Select your device and application, and the tool will start tracking the application’s resource usage.
2. LLDB Debugger
The LLDB debugger, integrated within Android Studio, is used for debugging native C/C++ code in your Android apps. With breakpoints, memory, and variable inspections, this is a powerful tool for catching bugs in your native code.
Example: To debug native code, set breakpoints within your C/C++ code, then run your app in debug mode. The execution will pause at the breakpoints, and you can inspect variable values and step through the code.
3. LeakCanary
LeakCanary is an open-source library that helps detect and fix memory leaks in your Android apps. It notifies you whenever it detects a potential leak, saving a lot of time and effort spent identifying these tricky bugs.
Example: To use LeakCanary, add the following to your `build.gradle` file:
```gradle dependencies { debugImplementation 'com.squareup.leakcanary:leakcanary-android:2.7' } ```
Then, initialize LeakCanary in your custom Application class:
```kotlin class ExampleApplication : Application() { override fun onCreate() { super.onCreate() if (LeakCanary.isInAnalyzerProcess(this)) { return } LeakCanary.install(this) } } ```
4. Firebase Test Lab
Firebase Test Lab is a cloud-based app-testing infrastructure. With one operation, you can test your app across a wide variety of devices and device configurations.
Example: To run tests on Firebase Test Lab, navigate to the Firebase console and select Test Lab from the left menu. Click on “Run a Test”, select your APK and the devices you want to test on, and click “Start Tests”.
5. Techniques for Effective Debugging
- Breakpoint Debugging: One of the most commonly used debugging techniques, setting breakpoints at critical points in your code allows you to pause execution and inspect the app’s current state. This method is particularly useful for tracking down hard-to-find bugs.
- Logging: Properly placed log statements help monitor the execution flow of your application and the state of your variables at any given time.
- Unit Testing: Writing unit tests for your code can help catch bugs early in the development process, before you even start your manual or automated testing.
- Code Reviews: Regularly reviewing code with peers can help catch mistakes and also provide insights into improving the design or performance of your app.
- Reproducing Bugs: The first step in any debugging process should be to reproduce the bug consistently. Understanding the steps to reproduce a bug is crucial for efficient debugging.
- Isolating the Problem: Try to isolate the bug to a specific part of your code. This will help you focus your debugging efforts and may also make it easier to reproduce the bug.
Conclusion
Debugging can be a daunting task, especially when dealing with a large codebase. However, having knowledge of the right tools and the correct techniques can help streamline this process. That’s why businesses often prefer to hire Android developers, who are skilled in tackling these challenges. Remember, effective troubleshooting isn’t just about fixing a bug – it’s about understanding why that bug occurred in the first place. With practice, patience, and persistence, these developers become adept at debugging, making the Android app development journey more enjoyable and efficient.
Table of Contents
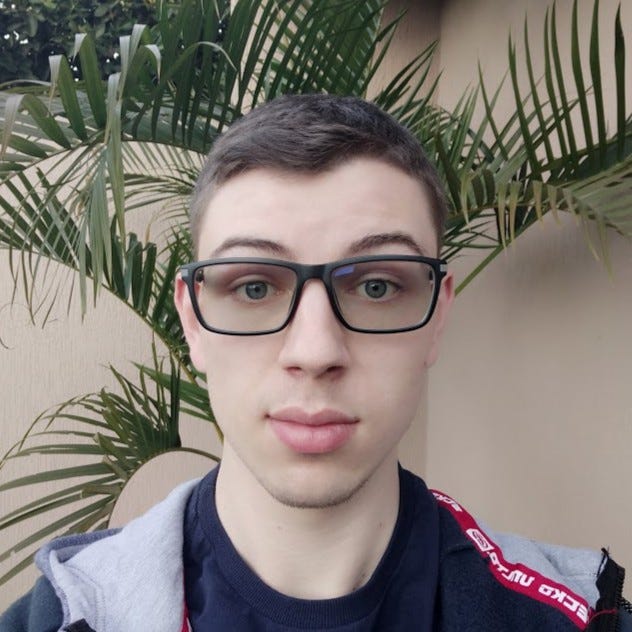
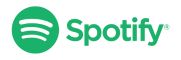