Simplify Your Android Applications: Exploring Dependency Injection in Detail
In the vast realm of Android application development, a world where businesses constantly hire Android developers, certain practices significantly contribute to the creation of cleaner, more modular, and more maintainable codebases. One such practice is Dependency Injection (DI). Although seemingly complex at first, DI can greatly streamline application setup and operation.
Table of Contents
As more organizations hire Android developers to stay competitive, understanding and applying these critical skills becomes even more important. This blog post aims to delve into Dependency Injection in Android, provide clear examples, and illustrate how it can simplify your application setup, helping both novice and experienced Android developers elevate their game.
1. What is Dependency Injection?
Dependency Injection is a coding design pattern that reduces hard-coded dependencies among classes, promoting loosely coupled and easily testable code. Instead of a class instantiating its dependencies directly, they are ‘injected’ into the class, typically via the constructor, a public setter method, or a dedicated injection method.
2. The Need for Dependency Injection in Android
Android applications can become rapidly complex. This complexity is not merely due to the number of classes and interfaces but also because of their interdependencies. When these dependencies are hardcoded, it becomes difficult to modify, test, or reuse the components.
For instance, consider an Android application with a `NetworkClient` class that fetches data from an API and a `DataRepository` class that uses `NetworkClient` to fetch the data and process it. If `DataRepository` directly instantiates `NetworkClient`, the two classes are tightly coupled. Modifying `NetworkClient` will impact `DataRepository`, and unit testing `DataRepository` without hitting the network becomes a challenge.
This is where Dependency Injection comes to the rescue. Instead of `DataRepository` creating `NetworkClient`, `NetworkClient` is created elsewhere and passed (injected) to `DataRepository`. This separation allows us to substitute `NetworkClient` with a mock object in unit tests, enhancing testability and maintainability of code.
3. Dependency Injection in Android with Dagger/Hilt
Dagger is a popular Dependency Injection framework used in Android, and Hilt is a library built on top of Dagger to simplify DI in Android. Let’s explore an example using Hilt.
First, set up Hilt in your project by adding the following dependencies in your build.gradle:
```java dependencies { implementation 'com.google.dagger:hilt-android:2.38.1' kapt 'com.google.dagger:hilt-android-compiler:2.38.1' } ```
Then, initialize Hilt by annotating your Application class with `@HiltAndroidApp`:
```java @HiltAndroidApp class MyApplication : Application() { } ```
Now, let’s create `NetworkClient` and `DataRepository` classes.
```java class NetworkClient { fun fetchData(): String { // Code to fetch data from the network } } class DataRepository(private val networkClient: NetworkClient) { fun getData(): String { return networkClient.fetchData() } } ```
Next, we need to instruct Hilt how to create instances of `NetworkClient` and `DataRepository`. We do this in a Module:
```java @Module @InstallIn(SingletonComponent::class) object AppModule { @Singleton @Provides fun provideNetworkClient(): NetworkClient { return NetworkClient() } @Provides fun provideDataRepository(networkClient: NetworkClient): DataRepository { return DataRepository(networkClient) } } ```
With these declarations, Hilt knows how to construct `NetworkClient` and `DataRepository`. We can now request these dependencies in our Android components.
Let’s see how we can inject `DataRepository` into an `Activity`:
```java class MainActivity : AppCompatActivity() { @Inject lateinit var dataRepository: DataRepository override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) val data = dataRepository.getData() // Use the data } } ```
By annotating `dataRepository` with `@Inject`, Hilt will provide the `DataRepository` instance when `MainActivity` is created.
This setup allows us to easily swap the real `NetworkClient` with a mock version for testing. We could create a different Hilt module for tests that provides a mock `NetworkClient`. Therefore, DI enhances the testability of our code.
Conclusion
Dependency Injection is a powerful design pattern that fosters code modularity, reusability, and testability, factors that are critical when businesses seek to hire Android developers. In Android, frameworks like Dagger and libraries like Hilt simplify the implementation of DI. While the initial setup might seem daunting, the benefits it brings to code maintainability and testability are significant and worthwhile, making you an attractive candidate for those looking to hire Android developers.
This guide has hopefully helped you grasp the nuances of Dependency Injection in Android and its potential to simplify your application setup. Armed with this knowledge, you’re now prepared to manage complex interdependencies in your applications with ease, creating robust, maintainable Android applications that stand out in the crowded marketplace. This mastery not only enhances your personal toolkit but also makes you a valuable asset for organizations planning to hire Android developers.
Happy coding!
Table of Contents
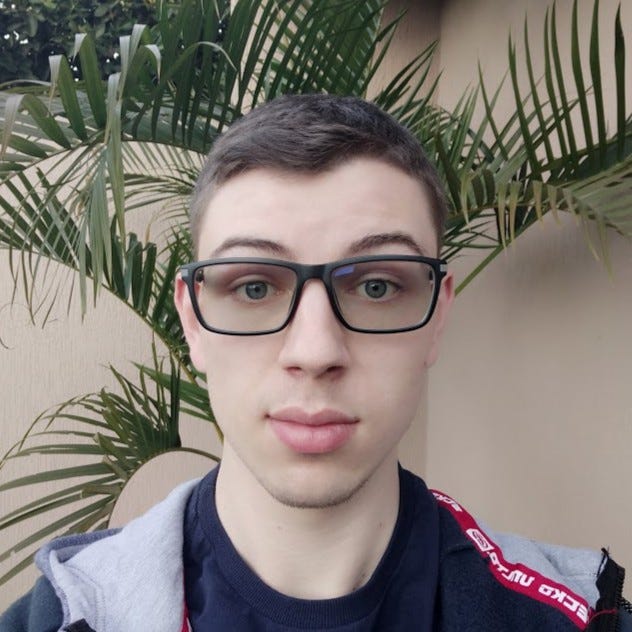
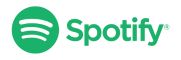