Exploring Android File System: Working with Internal and External Storage
In the realm of mobile app development, dealing with files and data storage is an essential aspect. Android, being one of the most popular mobile operating systems, offers various options for storing and managing files. In this blog, we will dive deep into the Android File System, understand the concepts of internal and external storage, explore their differences, and learn how to effectively work with them through code examples.
In Android, the File System is a structured approach to storing, accessing, and managing data and files on the device. The File System consists of two primary storage options: Internal Storage and External Storage. Understanding the differences between these two storage types is crucial for developing efficient and user-friendly Android applications.
1. Internal Storage
1.1. Understanding Internal Storage
Internal storage, as the name suggests, refers to the storage space that is private to the app itself. The data stored in the internal storage is only accessible to the app that created it. This makes it a secure option for storing sensitive user data that should not be accessible to other apps or users.
The internal storage of an app is a part of the device’s built-in storage and is always available to the app, regardless of whether the device has external storage (SD card) or not. However, it’s essential to note that the internal storage has limited space, and using it excessively might result in running out of storage, leading to potential crashes or data loss.
1.2. Accessing Internal Storage
To access the internal storage in an Android app, we use the Context object. The Context provides methods to open and create files or directories in the internal storage.
java // Get the context of the app Context context = getApplicationContext(); // Create or access a file in internal storage String filename = "example.txt"; File file = new File(context.getFilesDir(), filename);
1.3. Code Sample: Writing to Internal Storage
Writing data to the internal storage involves creating or opening a file and then writing data to it. Here’s an example of how you can write a string to a file in internal storage:
java // Writing data to the file String data = "Hello, this is some data to be written to the file."; try { FileOutputStream outputStream = new FileOutputStream(file); outputStream.write(data.getBytes()); outputStream.close(); Log.d("InternalStorage", "Data written to internal storage."); } catch (IOException e) { e.printStackTrace(); }
1.4. Code Sample: Reading from Internal Storage
Reading data from a file in internal storage is equally straightforward. You need to open the file and then read the data from it:
java // Reading data from the file try { FileInputStream inputStream = new FileInputStream(file); InputStreamReader inputStreamReader = new InputStreamReader(inputStream); BufferedReader bufferedReader = new BufferedReader(inputStreamReader); StringBuilder stringBuilder = new StringBuilder(); String line; while ((line = bufferedReader.readLine()) != null) { stringBuilder.append(line).append("\n"); } inputStream.close(); Log.d("InternalStorage", "Read data from internal storage: " + stringBuilder.toString()); } catch (IOException e) { e.printStackTrace(); }
1.5. Internal Storage Best Practices
When working with internal storage, consider the following best practices:
- Use Internal Storage for Sensitive Data: Since internal storage is private to the app, use it to store sensitive data like user credentials, authentication tokens, etc.
- Manage Storage Space: Be mindful of the limited storage space. Remove unnecessary files or offer options to the user to clear cache or old data.
- Backup and Restore: If the data is critical, provide backup and restore options to prevent data loss when the user changes devices or reinstalls the app.
2. External Storage
2.1. Understanding External Storage
Unlike internal storage, external storage is accessible by all apps on the device, and the user can also access it directly through a file manager. External storage usually refers to the SD card or any other storage media mounted on the device. Since external storage is shared, it’s suitable for storing files that can be shared between apps or files that the user may want to access independently.
While external storage offers more space compared to internal storage, not all devices have external storage, so it’s essential to handle scenarios where external storage might not be available.
2.2. Accessing External Storage
To access the external storage in an Android app, you need to check if it’s available and request the necessary permissions in the AndroidManifest.xml file.
xml <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" /> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
Next, you can use the Environment.getExternalStorageDirectory() method to get the path to the external storage directory:
java // Get the path to the external storage directory File externalDir = Environment.getExternalStorageDirectory();
2.3. Code Sample: Writing to External Storage
Writing to external storage follows a similar process as writing to internal storage. However, since external storage is shared, it’s good practice to check if it’s available and if you have the necessary permissions before proceeding with file operations:
java // Check if external storage is available and writable if (Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED)) { File externalFile = new File(externalDir, "example.txt"); // Continue with writing to the file } else { Log.d("ExternalStorage", "External storage not available or read-only."); }
2.4. Code Sample: Reading from External Storage
Reading from external storage also requires the same checks as writing. Here’s a sample code to read data from a file in external storage:
java // Check if external storage is available and readable if (Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED) || Environment.getExternalStorageState().equals(Environment.MEDIA_MOUNTED_READ_ONLY)) { File externalFile = new File(externalDir, "example.txt"); // Continue with reading from the file } else { Log.d("ExternalStorage", "External storage not available or not readable."); }
2.5. Permissions for External Storage
Starting from Android 10 (API level 29), the approach to accessing external storage changed. Instead of using WRITE_EXTERNAL_STORAGE permission, apps need to request a more specific permission called MANAGE_EXTERNAL_STORAGE. This permission allows apps to read and write from/to specific file paths on external storage.
Additionally, apps need to use the ACTION_OPEN_DOCUMENT_TREE intent to prompt the user to select a directory for storing their files on external storage.
3. Choosing between Internal and External Storage
The choice between internal and external storage depends on the type of data your app needs to store:
Use Internal Storage When:
- Storing sensitive data specific to the app.
- Avoiding the risk of data tampering from other apps or users.
- Ensuring data is available even if the device doesn’t have external storage.
Use External Storage When:
- Sharing data between multiple apps or making it accessible to the user.
- Storing large files that might exceed the internal storage limit.
- Providing an option to access files independently from other apps.
4. Working with File Directories
4.1. Creating App-Specific Directories
Android allows you to create app-specific directories in both internal and external storage. These directories are automatically removed when the user uninstalls the app.
To create an app-specific directory in internal storage, you can use the getDir() method:
java String dirName = "myAppDir"; File myAppDir = getDir(dirName, Context.MODE_PRIVATE);
For external storage, you can use the getExternalFilesDir() method:
java String dirName = "myAppDir"; File myAppDir = new File(getExternalFilesDir(null), dirName);
4.2. Code Sample: Creating a Directory
Here’s a code snippet to create a directory named “myDir” in internal storage:
java String dirName = "myDir"; File myDir = getDir(dirName, Context.MODE_PRIVATE); if (!myDir.exists()) { if (myDir.mkdirs()) { Log.d("FileDirectory", "Directory created: " + myDir.getAbsolutePath()); } else { Log.d("FileDirectory", "Failed to create directory."); } }
4.3. Code Sample: Listing Directory Contents
To list the contents of a directory, you can use the list() method:
java File[] files = myDir.listFiles(); if (files != null) { for (File file : files) { Log.d("FileDirectory", "File: " + file.getName()); } }
4.4. Code Sample: Deleting a Directory
To delete a directory and its contents, you can use the delete() method:
java if (myDir.exists()) { if (myDir.delete()) { Log.d("FileDirectory", "Directory deleted."); } else { Log.d("FileDirectory", "Failed to delete directory."); } }
Conclusion
Understanding the Android File System and its internal and external storage options is essential for effective mobile app development. Whether you need secure and private storage or shared and accessible storage, Android provides the necessary tools to handle file operations seamlessly. By implementing the code samples and best practices discussed in this blog, you can efficiently work with internal and external storage, creating robust and user-friendly applications.
Remember to consider the type of data your app handles and choose the appropriate storage option accordingly. Proper file handling ensures a smooth user experience and reduces the risk of data loss, enhancing the overall quality of your Android application. Happy coding!
Table of Contents
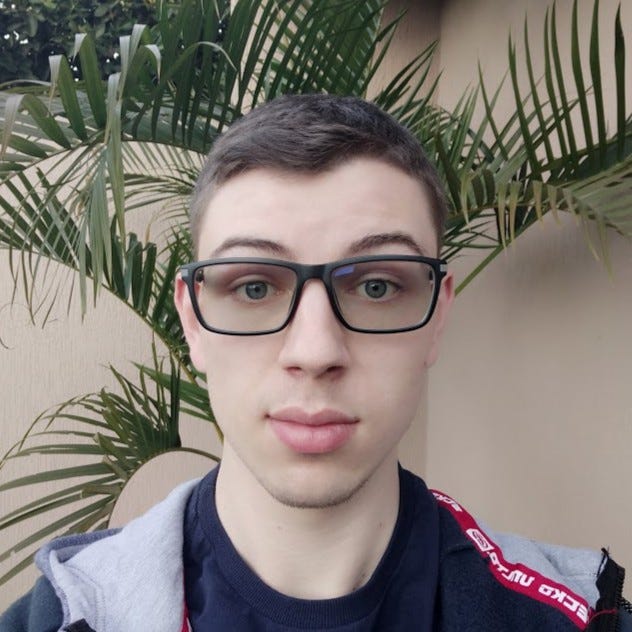
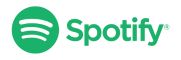