Elevate Your Android App with Firebase’s Real-time Firestore Database
Firebase Cloud Firestore is a NoSQL cloud database from Google that provides real-time synchronization, offline support, and scalability for mobile and web applications. It’s a part of the Firebase platform, which offers various tools for building modern apps. One of the standout features of Firestore is its ability to update data in real-time across connected devices. As the demand grows, many businesses look to hire Android developers skilled in Firebase integration. In this post, we’ll explore how to integrate Firebase Cloud Firestore into an Android application and showcase some practical examples.
Table of Contents
1. Why Firebase Cloud Firestore?
Before diving into the integration process, it’s essential to understand the value Firestore brings:
- Real-time synchronization: Changes to the data reflect instantly across all connected devices, making it suitable for apps that require real-time features.
- Offline support: Firestore caches data for offline use, ensuring your app functions smoothly even without an internet connection.
- Scalability: Firestore automatically scales based on the number of reads, writes, and the amount of data stored, making it suitable for both small and large applications.
- Flexible querying: Query data in complex ways without needing to fetch entire documents.
2. Integration Steps
2.1. Setting up Firebase
Before integrating Firestore, make sure you’ve set up Firebase in your Android project. Here’s a brief overview:
– Go to the [Firebase console](https://console.firebase.google.com/).
– Click on ‘Add project’.
– Follow the setup wizard. Once done, click on ‘Add Firebase to your Android app’.
– Enter your app’s package name and download the `google-services.json` file.
– Add the Firebase SDK by including the necessary dependencies in your app-level `build.gradle` file.
```gradle implementation 'com.google.firebase:firebase-firestore:XX.X.X' // replace XX.X.X with the latest version ```
2.2. Initialize Firestore:
In your main activity or application class, initialize Firebase:
```java import com.google.firebase.firestore.FirebaseFirestore; public class MainActivity extends AppCompatActivity { private FirebaseFirestore db; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); db = FirebaseFirestore.getInstance(); } } ```
3. Real-time Data Examples
Example 1: Add a new document
Let’s add a document to a collection called “users”.
```java Map<String, Object> user = new HashMap<>(); user.put("first", "John"); user.put("last", "Doe"); user.put("age", 30); db.collection("users") .add(user) .addOnSuccessListener(new OnSuccessListener<DocumentReference>() { @Override public void onSuccess(DocumentReference documentReference) { Log.d(TAG, "DocumentSnapshot added with ID: " + documentReference.getId()); } }) .addOnFailureListener(new OnFailureListener() { @Override public void onFailure(@NonNull Exception e) { Log.w(TAG, "Error adding document", e); } }); ```
Example 2: Listen for real-time updates:
To get real-time updates when a document changes, attach a listener:
```java final DocumentReference docRef = db.collection("users").document("user_id"); // Replace 'user_id' with a valid document ID docRef.addSnapshotListener(new EventListener<DocumentSnapshot>() { @Override public void onEvent(@Nullable DocumentSnapshot snapshot, @Nullable FirebaseFirestoreException e) { if (e != null) { Log.w(TAG, "Listen failed.", e); return; } if (snapshot != null && snapshot.exists()) { Log.d(TAG, "Current data: " + snapshot.getData()); } else { Log.d(TAG, "Current data: null"); } } }); ```
Example 3: Querying data
To retrieve all users aged over 25:
```java db.collection("users") .whereGreaterThan("age", 25) .addSnapshotListener(new EventListener<QuerySnapshot>() { @Override public void onEvent(@Nullable QuerySnapshot snapshots, @Nullable FirebaseFirestoreException e) { if (e != null) { Log.w(TAG, "listen:error", e); return; } for (DocumentChange dc : snapshots.getDocumentChanges()) { switch (dc.getType()) { case ADDED: Log.d(TAG, "New user: " + dc.getDocument().getData()); break; case MODIFIED: Log.d(TAG, "Modified user: " + dc.getDocument().getData()); break; case REMOVED: Log.d(TAG, "Removed user: " + dc.getDocument().getData()); break; } } } }); ```
Conclusion
Firebase Cloud Firestore offers a robust and scalable solution for apps needing real-time database functionality. With straightforward integration into Android applications, many businesses are keen to hire Android developers who can harness the power of real-time synchronization, offline support, and flexible querying. Firestore can truly transform the way you manage and sync data in your applications.
Remember, the key to successful integration is understanding Firestore’s document and collection structure, efficiently querying data, and handling real-time updates seamlessly. For those looking to hire Android developers, expertise in Firestore is a valuable asset. Happy coding!
Table of Contents
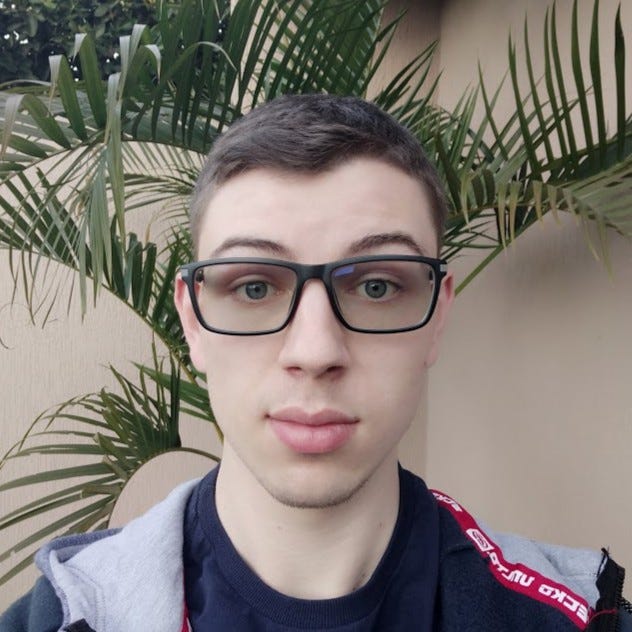
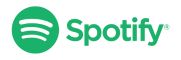