Android Notifications 101: FCM’s Role in Transforming User Experiences
Firebase Cloud Messaging (FCM) is a free, scalable messaging solution provided by Firebase that allows you to seamlessly integrate push notifications in your Android app. Push notifications are essential for engaging users and delivering timely updates. In this article, we’ll delve into how FCM makes implementing push notifications simple, and walk through some hands-on examples.
Table of Contents
1. What is Firebase Cloud Messaging (FCM)?
Firebase Cloud Messaging (FCM) is a cross-platform messaging solution that lets you deliver messages reliably at no cost. With FCM, you can send two types of messages:
- Notification messages: These are automatically displayed by Firebase SDK, and they are used mainly for informational purposes.
- Data messages: These are processed by the client app, providing developers with more control over their delivery and handling.
2. Setting up FCM in Your Android Project
Before diving into code examples, here’s a quick setup guide:
- Add Firebase to your Android project: If you haven’t already, add Firebase to your Android project by following [this guide](https://firebase.google.com/docs/android/setup).
- Add FCM Dependency: In your app-level `build.gradle` file, add the Firebase messaging dependency:
```gradle implementation 'com.google.firebase:firebase-messaging:latest_version' ```
- Add Permissions: Ensure that these permissions are present in your `AndroidManifest.xml`:
```xml <uses-permission android:name="android.permission.INTERNET" /> <uses-permission android:name="android.permission.WAKE_LOCK" /> ```
3. Sending and Receiving Notifications
3.1. Receiving Notifications
To handle FCM messages, you need to set up a service:
```kotlin class MyFirebaseMessagingService : FirebaseMessagingService() { override fun onMessageReceived(remoteMessage: RemoteMessage) { super.onMessageReceived(remoteMessage) // Handle FCM Notification Message remoteMessage.notification?.let { showNotification(it.title, it.body) } // Handle FCM Data Message remoteMessage.data.let { // Handle the data payload here } } private fun showNotification(title: String?, body: String?) { // Implement your notification logic here } } ```
Add this service to your `AndroidManifest.xml`:
```xml <service android:name=".MyFirebaseMessagingService" android:exported="false"> <intent-filter> <action android:name="com.google.firebase.MESSAGING_EVENT" /> </intent-filter> </service> ```
3.2. Sending Notifications
While you can send notifications from the Firebase console, many applications will find it useful to send them programmatically. Here’s a simple Python script to send a notification using FCM:
```python import requests FCM_URL = "https://fcm.googleapis.com/fcm/send" API_KEY = "YOUR_SERVER_KEY" headers = { "Authorization": f"key={API_KEY}", "Content-Type": "application/json" } data = { "to": "FCM_REGISTRATION_TOKEN", # or "/topics/TOPIC_NAME" "notification": { "title": "Your Notification Title", "body": "Your Notification Body", } } response = requests.post(FCM_URL, headers=headers, json=data) print(response.json()) ```
Replace `YOUR_SERVER_KEY` with your FCM server key from the Firebase console and `FCM_REGISTRATION_TOKEN` with the registration token from your client device or a topic name if sending to a topic.
4. Topics and Subscription
FCM allows you to define topics and let users subscribe to these topics. When you send a message to a topic, all subscribed users receive it.
Subscribing to a Topic:
```kotlin FirebaseMessaging.getInstance().subscribeToTopic("news") .addOnCompleteListener { task -> if (!task.isSuccessful) { // Handle failure } } ```
Unsubscribing from a Topic:
```kotlin FirebaseMessaging.getInstance().unsubscribeFromTopic("news") ```
5. Advanced Features and Considerations
– Priority: FCM allows setting the priority of messages. Use `high` priority for important messages as they wake up idle devices. Use `normal` priority for less critical messages.
– Time To Live (TTL): If a message is not urgent, you can set a TTL (time-to-live). It dictates how long a message should wait to be delivered if the device is offline.
– Handling in Foreground: By default, if your app is in the foreground, the notification message won’t be shown. Handle it within `onMessageReceived()`.
Conclusion
Firebase Cloud Messaging simplifies the process of integrating push notifications into your Android application. It not only abstracts away the complexities but also provides advanced features that give developers flexibility in handling notifications.
By following the steps and examples above, you can easily set up FCM in your Android app and harness the power of push notifications to enhance user engagement and deliver timely updates.
Table of Contents
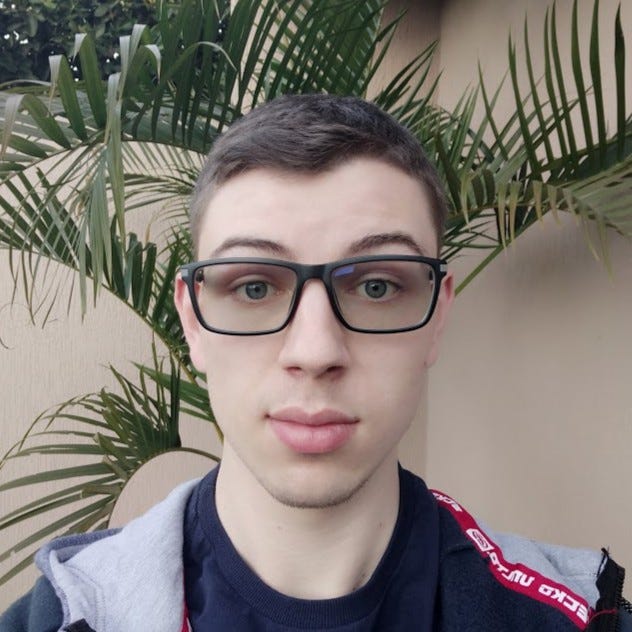
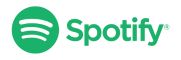