Geolocation Mastery: Enhancing User Experience with Android’s Mapping Tools
The smartphone revolution has led to a surge in location-based services. Whether you’re hailing a ride, tracking a workout, or hunting for the best local pizza, geolocation plays an integral role. For those looking to harness these capabilities, hiring Android developers becomes essential. Android, being one of the most widespread mobile operating systems, offers a comprehensive set of tools and services to integrate geolocation into applications.
Table of Contents
In this blog post, we will delve into the process of integrating maps and location services on Android, with a particular emphasis on Google Maps and Android’s Location API.
1. Google Maps API
The Google Maps API is a set of tools that allow you to integrate Google Maps into your Android applications.
1.1. Setting Up
To use the Google Maps API:
- Go to the [Google Cloud Console](https://console.cloud.google.com/).
- Create a new project.
- Navigate to the APIs & Services -> Library, then search and enable ‘Maps SDK for Android’.
- Obtain the API key.
1.2. Integration
1. Add the API key to your project’s `AndroidManifest.xml`:
```xml <meta-data android:name="com.google.android.geo.API_KEY" android:value="YOUR_API_KEY"/> ```
2. Add the Map fragment to your layout file:
```xml <fragment xmlns:android="http://schemas.android.com/apk/res/android" xmlns:map="http://schemas.android.com/apk/res-auto" android:id="@+id/map" android:name="com.google.android.gms.maps.SupportMapFragment" android:layout_width="match_parent" android:layout_height="match_parent"/> ```
1.3. Displaying the Map
To display the map in an activity, you need to obtain the `SupportMapFragment` and get notified when the map is ready to be used.
```java public class MapsActivity extends FragmentActivity implements OnMapReadyCallback { private GoogleMap mMap; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_maps); SupportMapFragment mapFragment = (SupportMapFragment) getSupportFragmentManager() .findFragmentById(R.id.map); mapFragment.getMapAsync(this); } @Override public void onMapReady(GoogleMap googleMap) { mMap = googleMap; // Add a marker in Sydney, Australia, and move the camera. LatLng sydney = new LatLng(-34, 151); mMap.addMarker(new MarkerOptions().position(sydney).title("Marker in Sydney")); mMap.moveCamera(CameraUpdateFactory.newLatLng(sydney)); } } ```
2. Android’s Location API
The Android framework offers the Location API to determine a device’s location using various inputs.
2.1. Setting Up
1. Add the necessary permissions in the `AndroidManifest.xml`:
```xml <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> ```
2. Request runtime permissions for location access (for Android 6.0 and above):
```java if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) { ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, 1); return; } ```
2.2. Retrieving Location
```java LocationManager locationManager = (LocationManager) getSystemService(Context.LOCATION_SERVICE); LocationListener locationListener = new LocationListener() { public void onLocationChanged(Location location) { double lat = location.getLatitude(); double lon = location.getLongitude(); } // Other methods like onProviderDisabled, onProviderEnabled, etc. }; locationManager.requestLocationUpdates(LocationManager.GPS_PROVIDER, 0, 0, locationListener); ```
2.3. Combining with Google Maps
Using the retrieved location, you can then update the Google Maps view:
```java public void onLocationChanged(Location location) { double lat = location.getLatitude(); double lon = location.getLongitude(); LatLng currentLocation = new LatLng(lat, lon); mMap.addMarker(new MarkerOptions().position(currentLocation).title("You are here!")); mMap.moveCamera(CameraUpdateFactory.newLatLngZoom(currentLocation, 15)); } ```
Conclusion
Android offers a robust set of tools to seamlessly integrate geolocation capabilities into applications. By effectively using the Google Maps API in conjunction with Android’s Location API, developers can deliver rich, context-aware applications that provide users with enhanced experiences, be it navigation, local searches, or myriad other location-based services. For those aiming to leverage these advanced features, it’s often a wise decision to hire Android developers who specialize in such integrations. Always remember, though, to handle user permissions respectfully and ensure the privacy of their location data
Table of Contents
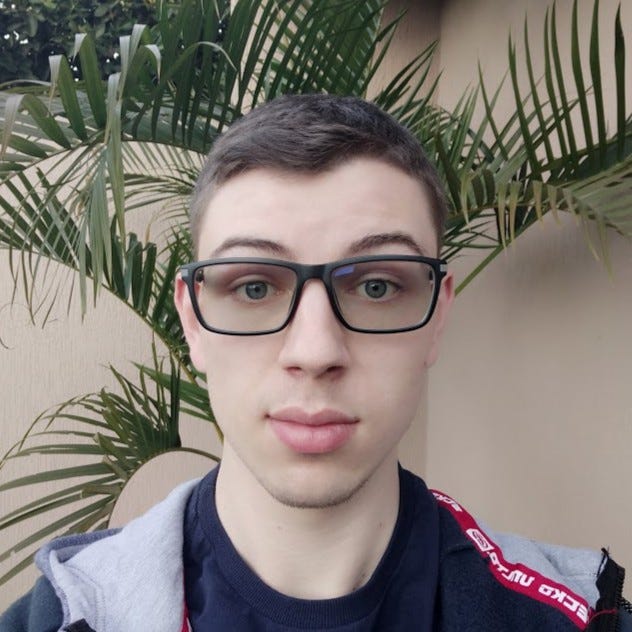
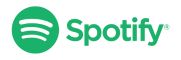