Exploring Android Gesture Recognition: Adding Touch Interaction
Smartphones have revolutionized the way we interact with technology. One of the key advancements in this realm is gesture recognition, which allows us to engage with our devices in a more intuitive and immersive manner. Android, being one of the world’s most popular mobile operating systems, offers a comprehensive suite of tools and APIs to implement gesture recognition in apps. This blog post aims to guide you through the process of adding touch interactions to your Android application.
1. The Power of Gestures
Before we jump into the how, let’s explore the why. Why are gestures so integral to the modern user experience? It’s simple – they provide a more intuitive, natural way to interact with apps. Swiping, pinching, zooming, or rotating can manipulate on-screen elements in ways that buttons or menus cannot. It’s this seamless integration of user interaction and visual feedback that makes gestures a must-have feature in today’s app development landscape.
2. Getting Started with Gesture Recognition
Android provides several classes for recognizing gestures. The two most important ones are the GestureDetector and ScaleGestureDetector classes. GestureDetector is used for simple gestures like single touch, double touch, long press, scroll, and fling. ScaleGestureDetector, on the other hand, is used for detecting multi-touch gestures like pinch and stretch.
3. Setting up the GestureDetector
Here’s an example of how to set up a GestureDetector:
java GestureDetector gestureDetector = new GestureDetector(this, new GestureDetector.SimpleOnGestureListener() { @Override public boolean onDown(MotionEvent event) { // Called when the user first touches the screen. return true; } @Override public boolean onFling(MotionEvent event1, MotionEvent event2, float velocityX, float velocityY) { // Called when the user performs a fling gesture. return true; } });
Here, onDown is triggered when a user first touches the screen, while onFling is called when the user executes a quick, swipe-like gesture.
4. Setting up the ScaleGestureDetector
Let’s take a look at how to set up a ScaleGestureDetector:
java ScaleGestureDetector scaleGestureDetector = new ScaleGestureDetector(this, new ScaleGestureDetector.SimpleOnScaleGestureListener() { @Override public boolean onScale(ScaleGestureDetector detector) { // Called when the user performs a pinch or stretch gesture. return true; } });
The onScale method is invoked when a user performs a pinch or stretch gesture.
5. Handling Touch Events
Now that we have set up our gesture detectors, we need to feed them touch events. These events can be captured in the onTouchEvent method of your view or activity. Here’s how:
java @Override public boolean onTouchEvent(MotionEvent event) { // Pass the event to the gesture detectors. gestureDetector.onTouchEvent(event); scaleGestureDetector.onTouchEvent(event); return super.onTouchEvent(event); }
6. Expanding the Gesture Vocabulary
While the above code samples cover basic gestures, Android also allows you to recognize more complex, custom gestures using the GestureOverlayView and GestureLibrary classes.
GestureOverlayView is a special type of view where gestures can be drawn, and GestureLibrary is a pre-defined set of gestures that are loaded into memory.
java GestureOverlayView gestureOverlayView = new GestureOverlayView(this); gestureOverlayView.setGestureColor(Color.TRANSPARENT); gestureOverlayView.setGestureVisible(true); gestureOverlayView.setEventsInterceptionEnabled(true); gestureOverlayView.setFadeEnabled(true); GestureLibrary library = GestureLibraries.fromRawResource(this, R.raw.gestures); if (!library.load()) { finish(); }
The above code creates a GestureOverlayView and loads a gesture library from a raw resource file.
Android’s gesture recognition capability offers an exciting avenue for creating more intuitive, user-friendly apps. It enables users to engage with your app in a natural, immersive way, greatly improving the overall user experience. With this guide, you now have a basic understanding of how to incorporate touch interactions into your Android applications. The next step is to experiment with these tools and create unique, captivating experiences for your users.
Remember, technology is all about making life simpler. Gesture recognition is one such tool that allows us to simplify user interactions. So go ahead, implement it, and let your app users swipe, pinch, and zoom their way to a delightful user experience. Happy coding!
Conclusion
Android’s gesture recognition tools provide developers with a powerful means to enhance their applications. Whether you’re aiming for a more immersive game interface, a slick photo editing app, or an innovative tool for artists, the ability to understand and respond to user gestures can significantly enrich your application’s interactivity. Implementing these techniques can be an exciting journey, and the possibilities are limited only by your imagination. Embrace the touch, and give your applications a new dimension of user interaction.
Table of Contents
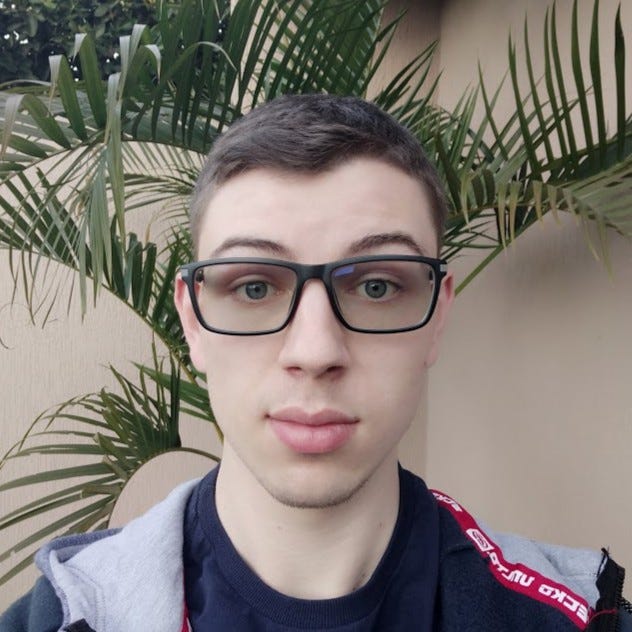
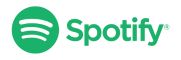