Integrating Firebase in Android Apps: Real-Time Data and Analytics
In today’s fast-paced world, real-time data and analytics have become crucial for the success of mobile applications. Firebase, a powerful mobile development platform, offers a suite of tools and services to help developers build high-quality apps with real-time data synchronization and robust analytics capabilities. In this comprehensive guide, we will explore how to integrate Firebase into your Android applications, enabling you to leverage its real-time data and analytics features.
1. What is Firebase?
Firebase is a comprehensive mobile development platform acquired by Google that provides developers with a wide range of tools and services to build and scale high-quality apps. It offers features such as real-time database, authentication, cloud storage, hosting, and more. In this guide, we will focus on integrating Firebase’s real-time data and analytics capabilities into Android applications.
2. Setting Up Firebase for Your Android Project
2.1 Creating a Firebase Project
To get started with Firebase, you need to create a Firebase project. Follow these steps:
Step 1: Go to the Firebase Console (https://console.firebase.google.com/).
Step 2: Click on the “Add project” button and provide a name for your project.
Step 3: Choose your desired project settings and click on the “Create project” button.
2.2 Adding Firebase to Your Android Project
Once you have created your Firebase project, you need to add it to your Android project. Here’s how:
Step 1: Open your Android project in Android Studio.
Step 2: In the Firebase Console, select your project and click on the “Add an app” button.
Step 3: Provide your Android package name and click on the “Register app” button.
Step 4: Download the google-services.json file and place it in the app module of your Android project.
Step 5: Open your project-level build.gradle file and add the following classpath dependency:
groovy classpath 'com.google.gms:google-services:4.3.10'
Step 6: Open your app-level build.gradle file and add the following plugin and dependencies:
groovy apply plugin: 'com.google.gms.google-services' dependencies { // Firebase Real-Time Database implementation 'com.google.firebase:firebase-database:20.0.0' // Firebase Analytics implementation 'com.google.firebase:firebase-analytics:20.0.1' }
3. Real-Time Database Integration
3.1 Introduction to Firebase Real-Time Database
Firebase Real-Time Database is a NoSQL cloud-hosted database that allows developers to store and sync data in real-time. It provides a flexible and scalable solution for managing app data and enables real-time updates across multiple devices.
3.2 Adding Firebase Real-Time Database Dependency
To integrate Firebase Real-Time Database into your Android app, add the following dependency in your app-level build.gradle file:
groovy implementation 'com.google.firebase:firebase-database:20.0.0'
3.3 Initializing Firebase Real-Time Database
Before accessing the Firebase Real-Time Database, you need to initialize it in your app’s entry point, typically the Application class or the main activity’s onCreate method. Add the following code:
java FirebaseApp.initializeApp(this);
3.4 Reading and Writing Data
To read and write data in the Firebase Real-Time Database, you can use the DatabaseReference class. Here’s an example of how to write data to the database:
java DatabaseReference databaseRef = FirebaseDatabase.getInstance().getReference(); databaseRef.child("users").child("userId").setValue("John Doe");
To read data from the database, you can use a ValueEventListener. Here’s an example of how to listen for changes in a specific location:
java DatabaseReference databaseRef = FirebaseDatabase.getInstance().getReference("users/userId"); databaseRef.addValueEventListener(new ValueEventListener() { @Override public void onDataChange(@NonNull DataSnapshot dataSnapshot) { String name = dataSnapshot.getValue(String.class); // Do something with the retrieved data } @Override public void onCancelled(@NonNull DatabaseError databaseError) { // Handle database error } });
3.5 Listening for Real-Time Updates
Firebase Real-Time Database allows you to listen for real-time updates, so you can receive notifications whenever the data changes. Here’s an example of how to listen for real-time updates:
java ValueEventListener eventListener = new ValueEventListener() { @Override public void onDataChange(@NonNull DataSnapshot dataSnapshot) { // Handle updated data } @Override public void onCancelled(@NonNull DatabaseError databaseError) { // Handle database error } }; databaseRef.addValueEventListener(eventListener);
4. Analytics Integration
4.1 Introduction to Firebase Analytics
Firebase Analytics is a powerful tool that helps you understand user behavior and measure the performance of your app. It provides valuable insights and allows you to track events, user properties, and more.
4.2 Adding Firebase Analytics Dependency
To integrate Firebase Analytics into your Android app, add the following dependency in your app-level build.gradle file:
groovy implementation 'com.google.firebase:firebase-analytics:20.0.1'
4.3 Initializing Firebase Analytics
Initialize Firebase Analytics by adding the following code to your app’s entry point:
java FirebaseAnalytics firebaseAnalytics = FirebaseAnalytics.getInstance(this);
4.4 Logging Events
Firebase Analytics allows you to log custom events to track user interactions and app usage. Here’s an example of how to log a custom event:
java Bundle bundle = new Bundle(); bundle.putString("book_title", "The Great Gatsby"); bundle.putString("category", "Fiction"); firebaseAnalytics.logEvent("book_viewed", bundle);
4.5 Setting User Properties
User properties provide additional information about your app’s users, such as their preferences or demographics. Here’s an example of how to set a user property:
java firebaseAnalytics.setUserProperty("favorite_genre", "Mystery");
Conclusion
Integrating Firebase in your Android applications enables you to leverage its real-time data synchronization and analytics capabilities. In this guide, we explored the step-by-step process of setting up Firebase for your Android project, integrating the Firebase Real-Time Database for real-time data management, and utilizing Firebase Analytics to gain valuable insights into user behavior. By following these instructions and utilizing the provided code samples, you can enhance your Android apps with seamless real-time data and powerful analytics capabilities using Firebase.
Table of Contents
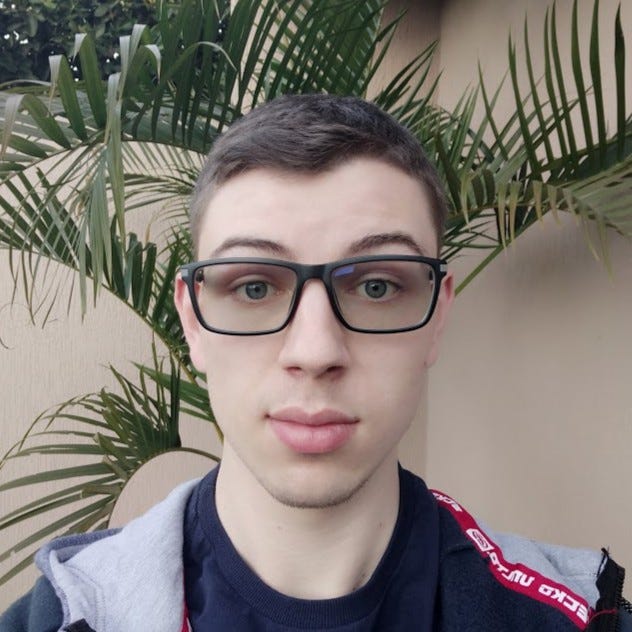
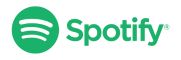