Managing Android Intents: Facilitating Inter-App Communication with Ease
Intents in Android are an essential component in developing robust and interactive applications, a fact well-known by those who regularly hire Android developers. An intent facilitates communication between different components, either within the same app or across different apps. This inter-component communication is a crucial part of creating interactive and user-friendly apps.
In this blog post, we will delve deep into intents, their types, and provide some examples demonstrating how to use them effectively. The insights shared here are beneficial not just for those looking to hire Android developers, but also for aspiring developers and tech enthusiasts alike.
Understanding Intents
Intents in Android are a software mechanism that allows components (like activities, services, and broadcast receivers) to request functionality from other Android components. They are used for various tasks, such as starting an activity, calling a service, or delivering a broadcast.
Intents are classified into two types: Explicit and Implicit Intents.
– Explicit Intents specify the component that should satisfy the intent, typically by providing the fully-qualified class name. They’re often used for activities within your app.
– Implicit Intents do not name a specific component. Instead, they declare a general action to perform, allowing a component from another app to handle it.
Explicit Intent
Let’s consider an example where we have two activities, ActivityA and ActivityB, and we want to navigate from ActivityA to ActivityB. We can do this using an explicit intent.
```java Intent intent = new Intent(ActivityA.this, ActivityB.class); startActivity(intent);
Here, the Intent constructor takes two parameters – a Context and the Class of the activity to start. This snippet will start ActivityB and place it at the top of the activity stack.
Passing Data with Explicit Intents
We can also pass data between activities using intents. For example, if we want to pass a string from ActivityA to ActivityB, we can do this:
```java Intent intent = new Intent(ActivityA.this, ActivityB.class); intent.putExtra("keyName","Hello, ActivityB!"); startActivity(intent);
In ActivityB, we can retrieve the data using the `getIntent().getStringExtra(“keyName”)` method.
```java String value = getIntent().getStringExtra("keyName");
Implicit Intent
Implicit intents do not specify a component. Instead, they specify an action and provide data upon which such an action should be performed. The Android system then compares the intent against all apps installed on the device to find an activity capable of handling the intent.
Consider a scenario where you want to view a webpage. We can use an implicit intent and the `ACTION_VIEW` action, passing the URI of the webpage.
```java Uri webpage = Uri.parse("http://www.example.com"); Intent intent = new Intent(Intent.ACTION_VIEW, webpage); if (intent.resolveActivity(getPackageManager()) != null) { startActivity(intent); }
The `intent.resolveActivity(getPackageManager())` method call is crucial to ensure there is an app on the device that can handle the intent. If not, the app would crash when we try to start the activity.
Using Implicit Intents for Sharing
Implicit intents are not only limited to system actions but can also facilitate inter-app communication. A common use case is sharing content from your app to another app.
Consider a scenario where you want to share a text from your app. We can do this using an implicit intent with `ACTION_SEND` action.
```java Intent intent = new Intent(Intent.ACTION_SEND); intent.setType("text/plain"); intent.putExtra(Intent.EXTRA_TEXT, "Hello, Share Intent!"); if (intent.resolveActivity(getPackageManager()) != null) { startActivity(intent); }
This intent will show a chooser dialog to the user, presenting a list of apps that can handle text sharing, and allow the user to pick an app to share the text.
Using Intents to Take Pictures
Another common use case is to use an intent to take a picture using the device’s camera. Here’s how you can do it:
```java Intent intent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE); if (intent.resolveActivity(getPackageManager()) != null) { startActivityForResult(intent, REQUEST_IMAGE_CAPTURE); }
The `startActivityForResult()` method is used when we expect a result to be returned from the started activity. The `REQUEST_IMAGE_CAPTURE` is a user-defined int constant that the system uses to match the result with the request.
Conclusion
Intents are a powerful feature in Android, enabling inter-communication between app components and different apps. This level of interactivity and flexibility is one reason why many businesses choose to hire Android developers. They offer the capability to perform a wide range of actions, from navigating between activities to executing complex tasks like capturing images or sharing content. By understanding and utilizing intents effectively, skilled Android developers can create rich, interactive experiences in your Android applications. Remember, while intents facilitate broad functionality, they should be used judiciously to respect user privacy and data security.
Table of Contents
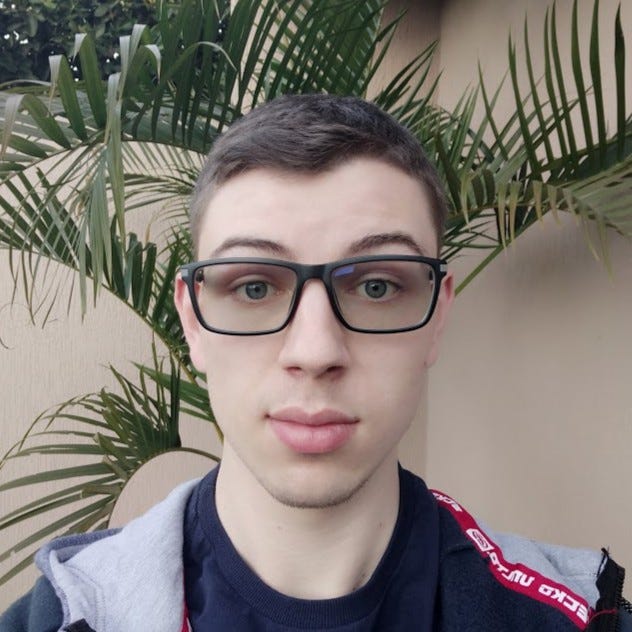
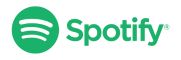