Exploring Android Jetpack: Boosting Development Productivity
Android Jetpack has revolutionized Android app development by providing developers with a comprehensive suite of libraries, tools, and architectural guidance. This powerful collection of components simplifies the development process, enhances code quality, and boosts productivity. In this blog post, we will delve into the world of Android Jetpack and explore its key features, benefits, and how it can accelerate your development workflow.
What is Android Jetpack?
Android Jetpack is a set of libraries, tools, and architectural guidance provided by Google to help developers build high-quality Android applications more efficiently. It encompasses a wide range of components and best practices that adhere to the modern Android development principles. Android Jetpack is designed to simplify and accelerate the development process by providing modular, reusable, and backward-compatible components.
Key Components of Android Jetpack
Android Jetpack comprises several key components, each addressing different aspects of app development. These components are categorized into three main groups: Architecture Components, UI Components, and Behavior Components.
1. Architecture Components
Architecture Components provide a set of libraries that help developers design robust and maintainable architectures for their apps. Two essential components in this category are ViewModel and LiveData.
1.1. ViewModel
ViewModel is a class that holds and manages UI-related data in a lifecycle-aware manner. It survives configuration changes and eliminates the need for manually handling data persistence during configuration changes, such as screen rotations. ViewModel is typically used to store and manage data required for the UI and communicate with other components, such as repositories or services.
Sample code:
kotlin class MyViewModel : ViewModel() { private val data: MutableLiveData<String> = MutableLiveData() fun getData(): LiveData<String> { return data } fun fetchData() { // Fetch data from a repository or service data.value = "Fetched data" } }
1.2. LiveData
LiveData is an observable data holder class that allows components, such as activities, fragments, or services, to observe changes in the data. LiveData is lifecycle-aware, meaning it automatically manages subscriptions and ensures that observers are only notified when the component is in an active state. It provides a simple and efficient way to propagate data updates to the UI.
Sample code:
kotlin class MyFragment : Fragment() { private val viewModel: MyViewModel by viewModels() override fun onViewCreated(view: View, savedInstanceState: Bundle?) { super.onViewCreated(view, savedInstanceState) viewModel.getData().observe(viewLifecycleOwner) { data -> // Update UI with the new data } } }
2. UI Components
UI Components in Android Jetpack are focused on creating intuitive and responsive user interfaces. Two commonly used UI components are RecyclerView and Fragment.
2.1. RecyclerView
RecyclerView is a powerful and flexible view for presenting large datasets efficiently. It replaces the older ListView and GridView components, offering better performance and more customization options. RecyclerView employs a flexible adapter pattern, allowing developers to display complex lists or grids with ease.
Sample code:
kotlin class MyAdapter(private val items: List<String>) : RecyclerView.Adapter<MyViewHolder>() { override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): MyViewHolder { val view = LayoutInflater.from(parent.context).inflate(R.layout.item_layout, parent, false) return MyViewHolder(view) } override fun onBindViewHolder(holder: MyViewHolder, position: Int) { val item = items[position] holder.bind(item) } override fun getItemCount(): Int { return items.size } } class MyViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) { fun bind(item: String) { // Bind data to the views } }
2.2. Fragment
Fragment represents a portion of the user interface or an independent screen in an app’s UI flow. It provides a modular approach to UI design and can be combined or reused in different activities or other fragments. Fragments are essential for building responsive and dynamic user interfaces.
Sample code:
kotlin class MyFragment : Fragment(R.layout.fragment_layout) { // Fragment-specific code }
3. Behavior Components
Behavior Components in Android Jetpack handle common behaviors or tasks that are crucial for many Android apps. Two notable behavior components are Navigation and WorkManager.
3.1. Navigation
Navigation is a component that simplifies the implementation of in-app navigation. It provides a declarative way to define app navigation paths and handles transitions between different destinations, such as fragments or activities. Navigation reduces the boilerplate code required for managing navigation manually.
3.2. WorkManager
WorkManager is a powerful component for efficiently scheduling and executing deferrable background tasks. It takes into account system constraints, such as battery level or network connectivity, to ensure that work executes at the most appropriate time. WorkManager simplifies task scheduling, retries, and chaining, making it easy to handle complex background operations.
4. Android Jetpack Benefits
Using Android Jetpack offers several benefits that significantly enhance the development process and the quality of Android apps.
4.1 Simplified Development
Android Jetpack provides a set of standardized libraries and components that simplify common development tasks. It reduces the boilerplate code required for handling configurations, lifecycles, data management, and navigation. Developers can focus more on building app features and less on infrastructure.
4.2 Enhanced Code Quality
Architecture Components in Android Jetpack promote clean and testable code architectures, such as the Model-View-ViewModel (MVVM) pattern. By following best practices and utilizing components like ViewModel and LiveData, developers can write code that is easier to understand, maintain, and test. This leads to higher code quality and fewer bugs.
4.3 Improved Testing
Jetpack’s architecture components, such as ViewModel and LiveData, are designed to be testable. They provide built-in support for writing unit tests, making it easier to verify the behavior of individual components. Additionally, Jetpack encourages modular app architectures, which further facilitate testing by allowing components to be tested in isolation.
4.4 Increased Productivity
Android Jetpack’s modular nature and backward compatibility enable developers to adopt new features and technologies without rewriting their entire codebase. Jetpack’s extensive set of components also accelerates development by providing ready-to-use solutions for common tasks. This results in faster development cycles, quicker time to market, and increased developer productivity.
5. Exploring Jetpack in Practice
Now, let’s explore how to leverage Android Jetpack in practice by examining the implementation of various components.
5.1 Setting Up Jetpack in Your Project
To get started with Android Jetpack, ensure that you have the necessary dependencies added to your project’s build.gradle file. You can include the desired components by adding their corresponding dependencies to the dependencies block. For example, to include ViewModel and LiveData, add the following lines:
groovy implementation 'androidx.lifecycle:lifecycle-viewmodel-ktx:2.4.0' implementation 'androidx.lifecycle:lifecycle-livedata-ktx:2.4.0'
5.2 Working with ViewModel and LiveData
To use ViewModel and LiveData in your app, create a ViewModel class that extends the ViewModel base class. Inside the ViewModel, define LiveData objects to hold your app’s data. Use the LiveData objects to observe data changes in your UI components and update the UI accordingly.
5.3 Harnessing the Power of RecyclerView
To leverage RecyclerView, create a custom RecyclerView.Adapter class that extends RecyclerView.Adapter. Implement the necessary methods to define the item layout and bind data to the views. Use the adapter in your activity or fragment to display the data in a RecyclerView.
5.4 Navigating with Jetpack Navigation
Jetpack Navigation simplifies app navigation by using a navigation graph to define screens and their relationships. Define the destinations (fragments or activities) and their connections in the navigation graph file. Then, use the Navigation component to navigate between destinations programmatically or via XML attributes.
5.5 Scheduling Tasks with WorkManager
To schedule tasks with WorkManager, create a Worker class that performs the desired background work. Define the constraints and requirements for the task, such as network connectivity or device charging status. Enqueue the task with WorkManager, and it will be executed at the appropriate time, considering the specified constraints.
Conclusion
Android Jetpack is an indispensable toolkit for Android developers, offering a vast array of libraries and components that streamline development, improve code quality, and boost productivity. By adopting Android Jetpack, developers can leverage modern app development practices, simplify complex tasks, and create robust and efficient Android applications. Exploring the various components of Jetpack and incorporating them into your projects will undoubtedly elevate your development workflow and enhance the overall quality of your Android apps. So, get started with Jetpack today and unlock the full potential of Android app development!
Table of Contents
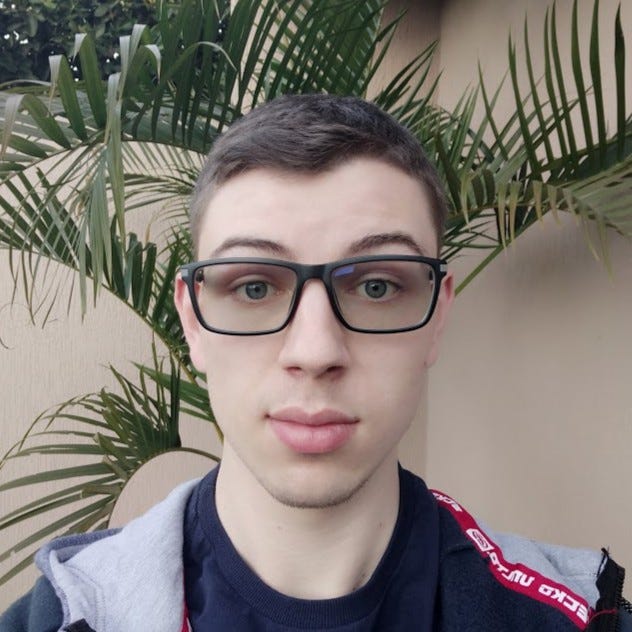
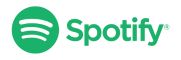