Kotlin for Android Development: Simplifying Code and Enhancing Productivity
Android app development has come a long way since its inception. With advancements in technology, the tools and languages used for Android development have evolved as well. One language that has gained immense popularity among Android developers is Kotlin. Developed by JetBrains, Kotlin is a modern, concise, and powerful programming language that runs on the Java Virtual Machine (JVM). In 2017, Google officially announced Kotlin as an official language for Android development, leading to its widespread adoption in the Android community.
Table of Contents
This blog post explores how Kotlin simplifies Android development, boosts developer productivity, and provides code samples to demonstrate its advantages.
1. Why Kotlin for Android Development?
1.1. Concise Syntax
Kotlin’s concise syntax reduces boilerplate code significantly, making it easier to read and maintain. Let’s compare a simple example between Java and Kotlin.
Java code:
java public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Kotlin code:
kotlin fun main() { println("Hello, World!") }
The Kotlin version is notably shorter and more expressive. It removes unnecessary ceremony and allows developers to focus on the core logic.
1.2. Null Safety
Null pointer exceptions are a common source of bugs in Android applications. Kotlin addresses this issue by making null safety a part of its type system. This means variables must be explicitly marked as nullable using the ? modifier when null values are allowed. The compiler will enforce null safety, reducing the risk of null pointer exceptions.
kotlin var name: String? = null // Safe call operator val length = name?.length
1.3. Extension Functions
Kotlin’s extension functions allow developers to add new functionalities to existing classes without modifying their source code. This feature is particularly useful for Android development, as it enables developers to work with Android framework classes and third-party libraries more efficiently.
kotlin // Example of an extension function on the String class fun String.isValidEmail(): Boolean { val pattern = "[a-zA-Z0-9._-]+@[a-z]+\\.+[a-z]+" return matches(Regex(pattern)) }
1.4. Data Classes
In Android development, dealing with data models is a common task. Kotlin’s data classes simplify this process by automatically generating standard methods like equals(), hashCode(), toString(), and copy(). This reduces boilerplate code and enhances productivity.
kotlin data class Person(val name: String, val age: Int)
1.5. Smart Casts
Kotlin’s smart casts automatically cast a variable to a specific type when certain conditions are met. This feature eliminates the need for explicit type casting, leading to cleaner and more readable code.
kotlin fun printLength(obj: Any) { if (obj is String) { println(obj.length) // No need for explicit casting } }
1.6. Coroutines
Asynchronous programming is essential in Android apps to prevent blocking the main thread. Kotlin’s built-in support for coroutines simplifies asynchronous programming, making it easier to write and understand asynchronous code. Coroutines allow developers to write asynchronous tasks in a sequential manner, improving code readability and maintainability.
kotlin // Example of a coroutine fun fetchData() { viewModelScope.launch { val data = fetchDataFromRemote() updateUI(data) } }
2. Boosting Productivity with Kotlin in Android Development
2.1. Enhanced Readability
As mentioned earlier, Kotlin’s concise syntax and null safety lead to more readable code. Clear and readable code reduces the time and effort required for maintenance and debugging.
2.2. Interoperability with Java
Kotlin is fully interoperable with Java, meaning developers can seamlessly use Kotlin in existing Java projects and vice versa. This allows developers to adopt Kotlin gradually and leverage its benefits without rewriting the entire codebase.
2.3. Improved Tooling Support
Kotlin’s increasing popularity has resulted in better tooling support from various Integrated Development Environments (IDEs) such as Android Studio. Code autocompletion, quick fixes, and refactoring tools for Kotlin have improved significantly, further enhancing developer productivity.
2.4. Community and Third-Party Libraries
With Kotlin becoming the language of choice for Android development, its community has grown rapidly. As a result, a vast number of Kotlin-specific libraries, extensions, and resources are available, empowering developers to build Android apps more efficiently.
Conclusion
Kotlin has undoubtedly simplified Android development and revolutionized the way developers create Android apps. Its concise syntax, null safety, extension functions, data classes, smart casts, and coroutines make the development process more enjoyable and productive.
By embracing Kotlin, Android developers can reduce boilerplate code, write more robust and readable applications, and take advantage of the language’s modern features. Additionally, Kotlin’s seamless interoperability with Java and improved tooling support ensure a smooth transition for developers and teams.
Whether you are a seasoned Android developer or just starting with Android app development, learning Kotlin is a valuable investment that will enhance your productivity and make your code more maintainable and efficient. Happy coding!
Table of Contents
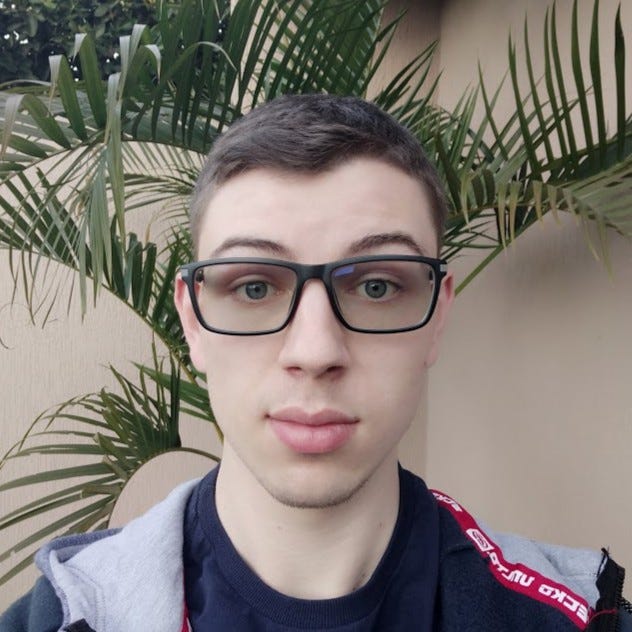
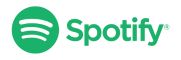